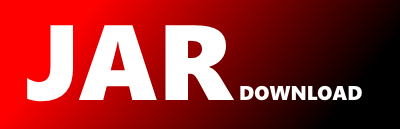
com.pulumi.aws.efs.outputs.GetFileSystemResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.efs.outputs;
import com.pulumi.aws.efs.outputs.GetFileSystemLifecyclePolicy;
import com.pulumi.aws.efs.outputs.GetFileSystemProtection;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetFileSystemResult {
/**
* @return Amazon Resource Name of the file system.
*
*/
private String arn;
/**
* @return The identifier of the Availability Zone in which the file system's One Zone storage classes exist.
*
*/
private String availabilityZoneId;
/**
* @return The Availability Zone name in which the file system's One Zone storage classes exist.
*
*/
private String availabilityZoneName;
private String creationToken;
/**
* @return DNS name for the filesystem per [documented convention](http://docs.aws.amazon.com/efs/latest/ug/mounting-fs-mount-cmd-dns-name.html).
*
*/
private String dnsName;
/**
* @return Whether EFS is encrypted.
*
*/
private Boolean encrypted;
private String fileSystemId;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return ARN for the KMS encryption key.
*
*/
private String kmsKeyId;
private List lifecyclePolicies;
/**
* @return File system [lifecycle policy](https://docs.aws.amazon.com/efs/latest/ug/API_LifecyclePolicy.html) object.
*
* @deprecated
* Use `lifecycle_policies` instead. This field will be removed in the next major version.
*
*/
@Deprecated /* Use `lifecycle_policies` instead. This field will be removed in the next major version. */
private GetFileSystemLifecyclePolicy lifecyclePolicy;
/**
* @return The value of the file system's `Name` tag.
*
*/
private String name;
/**
* @return File system performance mode.
*
*/
private String performanceMode;
private List protections;
/**
* @return The throughput, measured in MiB/s, that you want to provision for the file system.
*
*/
private Double provisionedThroughputInMibps;
/**
* @return Current byte count used by the file system.
*
*/
private Integer sizeInBytes;
/**
* @return A map of tags to assign to the file system.
*
*/
private Map tags;
/**
* @return Throughput mode for the file system.
*
*/
private String throughputMode;
private GetFileSystemResult() {}
/**
* @return Amazon Resource Name of the file system.
*
*/
public String arn() {
return this.arn;
}
/**
* @return The identifier of the Availability Zone in which the file system's One Zone storage classes exist.
*
*/
public String availabilityZoneId() {
return this.availabilityZoneId;
}
/**
* @return The Availability Zone name in which the file system's One Zone storage classes exist.
*
*/
public String availabilityZoneName() {
return this.availabilityZoneName;
}
public String creationToken() {
return this.creationToken;
}
/**
* @return DNS name for the filesystem per [documented convention](http://docs.aws.amazon.com/efs/latest/ug/mounting-fs-mount-cmd-dns-name.html).
*
*/
public String dnsName() {
return this.dnsName;
}
/**
* @return Whether EFS is encrypted.
*
*/
public Boolean encrypted() {
return this.encrypted;
}
public String fileSystemId() {
return this.fileSystemId;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return ARN for the KMS encryption key.
*
*/
public String kmsKeyId() {
return this.kmsKeyId;
}
public List lifecyclePolicies() {
return this.lifecyclePolicies;
}
/**
* @return File system [lifecycle policy](https://docs.aws.amazon.com/efs/latest/ug/API_LifecyclePolicy.html) object.
*
* @deprecated
* Use `lifecycle_policies` instead. This field will be removed in the next major version.
*
*/
@Deprecated /* Use `lifecycle_policies` instead. This field will be removed in the next major version. */
public GetFileSystemLifecyclePolicy lifecyclePolicy() {
return this.lifecyclePolicy;
}
/**
* @return The value of the file system's `Name` tag.
*
*/
public String name() {
return this.name;
}
/**
* @return File system performance mode.
*
*/
public String performanceMode() {
return this.performanceMode;
}
public List protections() {
return this.protections;
}
/**
* @return The throughput, measured in MiB/s, that you want to provision for the file system.
*
*/
public Double provisionedThroughputInMibps() {
return this.provisionedThroughputInMibps;
}
/**
* @return Current byte count used by the file system.
*
*/
public Integer sizeInBytes() {
return this.sizeInBytes;
}
/**
* @return A map of tags to assign to the file system.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Throughput mode for the file system.
*
*/
public String throughputMode() {
return this.throughputMode;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetFileSystemResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String availabilityZoneId;
private String availabilityZoneName;
private String creationToken;
private String dnsName;
private Boolean encrypted;
private String fileSystemId;
private String id;
private String kmsKeyId;
private List lifecyclePolicies;
private GetFileSystemLifecyclePolicy lifecyclePolicy;
private String name;
private String performanceMode;
private List protections;
private Double provisionedThroughputInMibps;
private Integer sizeInBytes;
private Map tags;
private String throughputMode;
public Builder() {}
public Builder(GetFileSystemResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.availabilityZoneId = defaults.availabilityZoneId;
this.availabilityZoneName = defaults.availabilityZoneName;
this.creationToken = defaults.creationToken;
this.dnsName = defaults.dnsName;
this.encrypted = defaults.encrypted;
this.fileSystemId = defaults.fileSystemId;
this.id = defaults.id;
this.kmsKeyId = defaults.kmsKeyId;
this.lifecyclePolicies = defaults.lifecyclePolicies;
this.lifecyclePolicy = defaults.lifecyclePolicy;
this.name = defaults.name;
this.performanceMode = defaults.performanceMode;
this.protections = defaults.protections;
this.provisionedThroughputInMibps = defaults.provisionedThroughputInMibps;
this.sizeInBytes = defaults.sizeInBytes;
this.tags = defaults.tags;
this.throughputMode = defaults.throughputMode;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder availabilityZoneId(String availabilityZoneId) {
if (availabilityZoneId == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "availabilityZoneId");
}
this.availabilityZoneId = availabilityZoneId;
return this;
}
@CustomType.Setter
public Builder availabilityZoneName(String availabilityZoneName) {
if (availabilityZoneName == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "availabilityZoneName");
}
this.availabilityZoneName = availabilityZoneName;
return this;
}
@CustomType.Setter
public Builder creationToken(String creationToken) {
if (creationToken == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "creationToken");
}
this.creationToken = creationToken;
return this;
}
@CustomType.Setter
public Builder dnsName(String dnsName) {
if (dnsName == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "dnsName");
}
this.dnsName = dnsName;
return this;
}
@CustomType.Setter
public Builder encrypted(Boolean encrypted) {
if (encrypted == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "encrypted");
}
this.encrypted = encrypted;
return this;
}
@CustomType.Setter
public Builder fileSystemId(String fileSystemId) {
if (fileSystemId == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "fileSystemId");
}
this.fileSystemId = fileSystemId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(String kmsKeyId) {
if (kmsKeyId == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "kmsKeyId");
}
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder lifecyclePolicies(List lifecyclePolicies) {
if (lifecyclePolicies == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "lifecyclePolicies");
}
this.lifecyclePolicies = lifecyclePolicies;
return this;
}
public Builder lifecyclePolicies(GetFileSystemLifecyclePolicy... lifecyclePolicies) {
return lifecyclePolicies(List.of(lifecyclePolicies));
}
@CustomType.Setter
public Builder lifecyclePolicy(GetFileSystemLifecyclePolicy lifecyclePolicy) {
if (lifecyclePolicy == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "lifecyclePolicy");
}
this.lifecyclePolicy = lifecyclePolicy;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder performanceMode(String performanceMode) {
if (performanceMode == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "performanceMode");
}
this.performanceMode = performanceMode;
return this;
}
@CustomType.Setter
public Builder protections(List protections) {
if (protections == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "protections");
}
this.protections = protections;
return this;
}
public Builder protections(GetFileSystemProtection... protections) {
return protections(List.of(protections));
}
@CustomType.Setter
public Builder provisionedThroughputInMibps(Double provisionedThroughputInMibps) {
if (provisionedThroughputInMibps == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "provisionedThroughputInMibps");
}
this.provisionedThroughputInMibps = provisionedThroughputInMibps;
return this;
}
@CustomType.Setter
public Builder sizeInBytes(Integer sizeInBytes) {
if (sizeInBytes == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "sizeInBytes");
}
this.sizeInBytes = sizeInBytes;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder throughputMode(String throughputMode) {
if (throughputMode == null) {
throw new MissingRequiredPropertyException("GetFileSystemResult", "throughputMode");
}
this.throughputMode = throughputMode;
return this;
}
public GetFileSystemResult build() {
final var _resultValue = new GetFileSystemResult();
_resultValue.arn = arn;
_resultValue.availabilityZoneId = availabilityZoneId;
_resultValue.availabilityZoneName = availabilityZoneName;
_resultValue.creationToken = creationToken;
_resultValue.dnsName = dnsName;
_resultValue.encrypted = encrypted;
_resultValue.fileSystemId = fileSystemId;
_resultValue.id = id;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.lifecyclePolicies = lifecyclePolicies;
_resultValue.lifecyclePolicy = lifecyclePolicy;
_resultValue.name = name;
_resultValue.performanceMode = performanceMode;
_resultValue.protections = protections;
_resultValue.provisionedThroughputInMibps = provisionedThroughputInMibps;
_resultValue.sizeInBytes = sizeInBytes;
_resultValue.tags = tags;
_resultValue.throughputMode = throughputMode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy