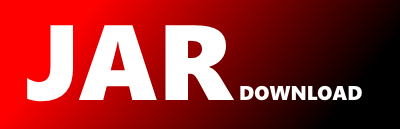
com.pulumi.aws.elasticache.inputs.ServerlessCacheState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticache.inputs;
import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsArgs;
import com.pulumi.aws.elasticache.inputs.ServerlessCacheEndpointArgs;
import com.pulumi.aws.elasticache.inputs.ServerlessCacheReaderEndpointArgs;
import com.pulumi.aws.elasticache.inputs.ServerlessCacheTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServerlessCacheState extends com.pulumi.resources.ResourceArgs {
public static final ServerlessCacheState Empty = new ServerlessCacheState();
/**
* The Amazon Resource Name (ARN) of the serverless cache.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return The Amazon Resource Name (ARN) of the serverless cache.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy