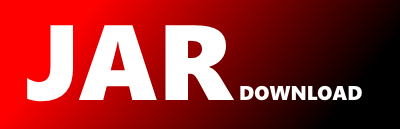
com.pulumi.aws.elasticbeanstalk.Environment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticbeanstalk;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.elasticbeanstalk.EnvironmentArgs;
import com.pulumi.aws.elasticbeanstalk.inputs.EnvironmentState;
import com.pulumi.aws.elasticbeanstalk.outputs.EnvironmentAllSetting;
import com.pulumi.aws.elasticbeanstalk.outputs.EnvironmentSetting;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Elastic Beanstalk Environment Resource. Elastic Beanstalk allows
* you to deploy and manage applications in the AWS cloud without worrying about
* the infrastructure that runs those applications.
*
* Environments are often things such as `development`, `integration`, or
* `production`.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticbeanstalk.Application;
* import com.pulumi.aws.elasticbeanstalk.ApplicationArgs;
* import com.pulumi.aws.elasticbeanstalk.Environment;
* import com.pulumi.aws.elasticbeanstalk.EnvironmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var tftest = new Application("tftest", ApplicationArgs.builder()
* .name("tf-test-name")
* .description("tf-test-desc")
* .build());
*
* var tfenvtest = new Environment("tfenvtest", EnvironmentArgs.builder()
* .name("tf-test-name")
* .application(tftest.name())
* .solutionStackName("64bit Amazon Linux 2015.03 v2.0.3 running Go 1.4")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Option Settings
*
* Some options can be stack-specific, check [AWS Docs](https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/command-options-general.html)
* for supported options and examples.
*
* The `setting` and `all_settings` mappings support the following format:
*
* * `namespace` - unique namespace identifying the option's associated AWS resource
* * `name` - name of the configuration option
* * `value` - value for the configuration option
* * `resource` - (Optional) resource name for [scheduled action](https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/command-options-general.html#command-options-general-autoscalingscheduledaction)
*
* ### Example With Options
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticbeanstalk.Application;
* import com.pulumi.aws.elasticbeanstalk.ApplicationArgs;
* import com.pulumi.aws.elasticbeanstalk.Environment;
* import com.pulumi.aws.elasticbeanstalk.EnvironmentArgs;
* import com.pulumi.aws.elasticbeanstalk.inputs.EnvironmentSettingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var tftest = new Application("tftest", ApplicationArgs.builder()
* .name("tf-test-name")
* .description("tf-test-desc")
* .build());
*
* var tfenvtest = new Environment("tfenvtest", EnvironmentArgs.builder()
* .name("tf-test-name")
* .application(tftest.name())
* .solutionStackName("64bit Amazon Linux 2015.03 v2.0.3 running Go 1.4")
* .settings(
* EnvironmentSettingArgs.builder()
* .namespace("aws:ec2:vpc")
* .name("VPCId")
* .value("vpc-xxxxxxxx")
* .build(),
* EnvironmentSettingArgs.builder()
* .namespace("aws:ec2:vpc")
* .name("Subnets")
* .value("subnet-xxxxxxxx")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Elastic Beanstalk Environments using the `id`. For example:
*
* ```sh
* $ pulumi import aws:elasticbeanstalk/environment:Environment prodenv e-rpqsewtp2j
* ```
*
*/
@ResourceType(type="aws:elasticbeanstalk/environment:Environment")
public class Environment extends com.pulumi.resources.CustomResource {
/**
* List of all option settings configured in this Environment. These
* are a combination of default settings and their overrides from `setting` in
* the configuration.
*
*/
@Export(name="allSettings", refs={List.class,EnvironmentAllSetting.class}, tree="[0,1]")
private Output> allSettings;
/**
* @return List of all option settings configured in this Environment. These
* are a combination of default settings and their overrides from `setting` in
* the configuration.
*
*/
public Output> allSettings() {
return this.allSettings;
}
/**
* Name of the application that contains the version
* to be deployed
*
*/
@Export(name="application", refs={String.class}, tree="[0]")
private Output application;
/**
* @return Name of the application that contains the version
* to be deployed
*
*/
public Output application() {
return this.application;
}
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
public Output arn() {
return this.arn;
}
/**
* The autoscaling groups used by this Environment.
*
*/
@Export(name="autoscalingGroups", refs={List.class,String.class}, tree="[0,1]")
private Output> autoscalingGroups;
/**
* @return The autoscaling groups used by this Environment.
*
*/
public Output> autoscalingGroups() {
return this.autoscalingGroups;
}
/**
* Fully qualified DNS name for this Environment.
*
*/
@Export(name="cname", refs={String.class}, tree="[0]")
private Output cname;
/**
* @return Fully qualified DNS name for this Environment.
*
*/
public Output cname() {
return this.cname;
}
/**
* Prefix to use for the fully qualified DNS name of
* the Environment.
*
*/
@Export(name="cnamePrefix", refs={String.class}, tree="[0]")
private Output cnamePrefix;
/**
* @return Prefix to use for the fully qualified DNS name of
* the Environment.
*
*/
public Output cnamePrefix() {
return this.cnamePrefix;
}
/**
* Short description of the Environment
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Short description of the Environment
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The URL to the Load Balancer for this Environment
*
*/
@Export(name="endpointUrl", refs={String.class}, tree="[0]")
private Output endpointUrl;
/**
* @return The URL to the Load Balancer for this Environment
*
*/
public Output endpointUrl() {
return this.endpointUrl;
}
/**
* Instances used by this Environment.
*
*/
@Export(name="instances", refs={List.class,String.class}, tree="[0,1]")
private Output> instances;
/**
* @return Instances used by this Environment.
*
*/
public Output> instances() {
return this.instances;
}
/**
* Launch configurations in use by this Environment.
*
*/
@Export(name="launchConfigurations", refs={List.class,String.class}, tree="[0,1]")
private Output> launchConfigurations;
/**
* @return Launch configurations in use by this Environment.
*
*/
public Output> launchConfigurations() {
return this.launchConfigurations;
}
/**
* Elastic load balancers in use by this Environment.
*
*/
@Export(name="loadBalancers", refs={List.class,String.class}, tree="[0,1]")
private Output> loadBalancers;
/**
* @return Elastic load balancers in use by this Environment.
*
*/
public Output> loadBalancers() {
return this.loadBalancers;
}
/**
* A unique name for this Environment. This name is used
* in the application URL
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return A unique name for this Environment. This name is used
* in the application URL
*
*/
public Output name() {
return this.name;
}
/**
* The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the Elastic Beanstalk [Platform](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment.html#cfn-beanstalk-environment-platformarn)
* to use in deployment
*
*/
@Export(name="platformArn", refs={String.class}, tree="[0]")
private Output platformArn;
/**
* @return The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the Elastic Beanstalk [Platform](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-beanstalk-environment.html#cfn-beanstalk-environment-platformarn)
* to use in deployment
*
*/
public Output platformArn() {
return this.platformArn;
}
/**
* The time between polling the AWS API to
* check if changes have been applied. Use this to adjust the rate of API calls
* for any `create` or `update` action. Minimum `10s`, maximum `180s`. Omit this to
* use the default behavior, which is an exponential backoff
*
*/
@Export(name="pollInterval", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> pollInterval;
/**
* @return The time between polling the AWS API to
* check if changes have been applied. Use this to adjust the rate of API calls
* for any `create` or `update` action. Minimum `10s`, maximum `180s`. Omit this to
* use the default behavior, which is an exponential backoff
*
*/
public Output> pollInterval() {
return Codegen.optional(this.pollInterval);
}
/**
* SQS queues in use by this Environment.
*
*/
@Export(name="queues", refs={List.class,String.class}, tree="[0,1]")
private Output> queues;
/**
* @return SQS queues in use by this Environment.
*
*/
public Output> queues() {
return this.queues;
}
/**
* Option settings to configure the new Environment. These
* override specific values that are set as defaults. The format is detailed
* below in Option Settings
*
*/
@Export(name="settings", refs={List.class,EnvironmentSetting.class}, tree="[0,1]")
private Output* @Nullable */ List> settings;
/**
* @return Option settings to configure the new Environment. These
* override specific values that are set as defaults. The format is detailed
* below in Option Settings
*
*/
public Output>> settings() {
return Codegen.optional(this.settings);
}
/**
* A solution stack to base your environment
* off of. Example stacks can be found in the [Amazon API documentation](https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/concepts.platforms.html)
*
*/
@Export(name="solutionStackName", refs={String.class}, tree="[0]")
private Output solutionStackName;
/**
* @return A solution stack to base your environment
* off of. Example stacks can be found in the [Amazon API documentation](https://docs.aws.amazon.com/elasticbeanstalk/latest/dg/concepts.platforms.html)
*
*/
public Output solutionStackName() {
return this.solutionStackName;
}
/**
* A set of tags to apply to the Environment. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A set of tags to apply to the Environment. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy