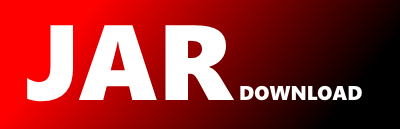
com.pulumi.aws.elasticsearch.Domain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticsearch;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.elasticsearch.DomainArgs;
import com.pulumi.aws.elasticsearch.inputs.DomainState;
import com.pulumi.aws.elasticsearch.outputs.DomainAdvancedSecurityOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainAutoTuneOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainClusterConfig;
import com.pulumi.aws.elasticsearch.outputs.DomainCognitoOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainDomainEndpointOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainEbsOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainEncryptAtRest;
import com.pulumi.aws.elasticsearch.outputs.DomainLogPublishingOption;
import com.pulumi.aws.elasticsearch.outputs.DomainNodeToNodeEncryption;
import com.pulumi.aws.elasticsearch.outputs.DomainSnapshotOptions;
import com.pulumi.aws.elasticsearch.outputs.DomainVpcOptions;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an AWS Elasticsearch Domain.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticsearch.Domain;
* import com.pulumi.aws.elasticsearch.DomainArgs;
* import com.pulumi.aws.elasticsearch.inputs.DomainClusterConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Domain("example", DomainArgs.builder()
* .domainName("example")
* .elasticsearchVersion("7.10")
* .clusterConfig(DomainClusterConfigArgs.builder()
* .instanceType("r4.large.elasticsearch")
* .build())
* .tags(Map.of("Domain", "TestDomain"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Access Policy
*
* > See also: `aws.elasticsearch.DomainPolicy` resource
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.elasticsearch.Domain;
* import com.pulumi.aws.elasticsearch.DomainArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var domain = config.get("domain").orElse("tf-test");
* final var current = AwsFunctions.getRegion();
*
* final var currentGetCallerIdentity = AwsFunctions.getCallerIdentity();
*
* var example = new Domain("example", DomainArgs.builder()
* .domainName(domain)
* .accessPolicies("""
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "es:*",
* "Principal": "*",
* "Effect": "Allow",
* "Resource": "arn:aws:es:%s:%s:domain/%s/*",
* "Condition": {
* "IpAddress": {"aws:SourceIp": ["66.193.100.22/32"]}
* }
* }
* ]
* }
* ", current.applyValue(getRegionResult -> getRegionResult.name()),currentGetCallerIdentity.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()),domain))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Log Publishing to CloudWatch Logs
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogGroup;
* import com.pulumi.aws.cloudwatch.LogGroupArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.cloudwatch.LogResourcePolicy;
* import com.pulumi.aws.cloudwatch.LogResourcePolicyArgs;
* import com.pulumi.aws.elasticsearch.Domain;
* import com.pulumi.aws.elasticsearch.DomainArgs;
* import com.pulumi.aws.elasticsearch.inputs.DomainLogPublishingOptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleLogGroup = new LogGroup("exampleLogGroup", LogGroupArgs.builder()
* .name("example")
* .build());
*
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("es.amazonaws.com")
* .build())
* .actions(
* "logs:PutLogEvents",
* "logs:PutLogEventsBatch",
* "logs:CreateLogStream")
* .resources("arn:aws:logs:*")
* .build())
* .build());
*
* var exampleLogResourcePolicy = new LogResourcePolicy("exampleLogResourcePolicy", LogResourcePolicyArgs.builder()
* .policyName("example")
* .policyDocument(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
*
* var exampleDomain = new Domain("exampleDomain", DomainArgs.builder()
* .logPublishingOptions(DomainLogPublishingOptionArgs.builder()
* .cloudwatchLogGroupArn(exampleLogGroup.arn())
* .logType("INDEX_SLOW_LOGS")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### VPC based ES
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Ec2Functions;
* import com.pulumi.aws.ec2.inputs.GetVpcArgs;
* import com.pulumi.aws.ec2.inputs.GetSubnetsArgs;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetRegionArgs;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.ec2.SecurityGroup;
* import com.pulumi.aws.ec2.SecurityGroupArgs;
* import com.pulumi.aws.ec2.inputs.SecurityGroupIngressArgs;
* import com.pulumi.aws.iam.ServiceLinkedRole;
* import com.pulumi.aws.iam.ServiceLinkedRoleArgs;
* import com.pulumi.aws.elasticsearch.Domain;
* import com.pulumi.aws.elasticsearch.DomainArgs;
* import com.pulumi.aws.elasticsearch.inputs.DomainClusterConfigArgs;
* import com.pulumi.aws.elasticsearch.inputs.DomainVpcOptionsArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var vpc = config.get("vpc");
* final var domain = config.get("domain").orElse("tf-test");
* final var selected = Ec2Functions.getVpc(GetVpcArgs.builder()
* .tags(Map.of("Name", vpc))
* .build());
*
* final var selectedGetSubnets = Ec2Functions.getSubnets(GetSubnetsArgs.builder()
* .filters(GetSubnetsFilterArgs.builder()
* .name("vpc-id")
* .values(selected.applyValue(getVpcResult -> getVpcResult.id()))
* .build())
* .tags(Map.of("Tier", "private"))
* .build());
*
* final var current = AwsFunctions.getRegion();
*
* final var currentGetCallerIdentity = AwsFunctions.getCallerIdentity();
*
* var es = new SecurityGroup("es", SecurityGroupArgs.builder()
* .name(String.format("%s-elasticsearch-%s", vpc,domain))
* .description("Managed by Pulumi")
* .vpcId(selected.applyValue(getVpcResult -> getVpcResult.id()))
* .ingress(SecurityGroupIngressArgs.builder()
* .fromPort(443)
* .toPort(443)
* .protocol("tcp")
* .cidrBlocks(selected.applyValue(getVpcResult -> getVpcResult.cidrBlock()))
* .build())
* .build());
*
* var esServiceLinkedRole = new ServiceLinkedRole("esServiceLinkedRole", ServiceLinkedRoleArgs.builder()
* .awsServiceName("opensearchservice.amazonaws.com")
* .build());
*
* var esDomain = new Domain("esDomain", DomainArgs.builder()
* .domainName(domain)
* .elasticsearchVersion("6.3")
* .clusterConfig(DomainClusterConfigArgs.builder()
* .instanceType("m4.large.elasticsearch")
* .zoneAwarenessEnabled(true)
* .build())
* .vpcOptions(DomainVpcOptionsArgs.builder()
* .subnetIds(
* selectedGetSubnets.applyValue(getSubnetsResult -> getSubnetsResult.ids()[0]),
* selectedGetSubnets.applyValue(getSubnetsResult -> getSubnetsResult.ids()[1]))
* .securityGroupIds(es.id())
* .build())
* .advancedOptions(Map.of("rest.action.multi.allow_explicit_index", "true"))
* .accessPolicies("""
* {
* "Version": "2012-10-17",
* "Statement": [
* {
* "Action": "es:*",
* "Principal": "*",
* "Effect": "Allow",
* "Resource": "arn:aws:es:%s:%s:domain/%s/*"
* }
* ]
* }
* ", current.applyValue(getRegionResult -> getRegionResult.name()),currentGetCallerIdentity.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()),domain))
* .tags(Map.of("Domain", "TestDomain"))
* .build(), CustomResourceOptions.builder()
* .dependsOn(esServiceLinkedRole)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Elasticsearch domains using the `domain_name`. For example:
*
* ```sh
* $ pulumi import aws:elasticsearch/domain:Domain example domain_name
* ```
*
*/
@ResourceType(type="aws:elasticsearch/domain:Domain")
public class Domain extends com.pulumi.resources.CustomResource {
/**
* IAM policy document specifying the access policies for the domain.
*
*/
@Export(name="accessPolicies", refs={String.class}, tree="[0]")
private Output accessPolicies;
/**
* @return IAM policy document specifying the access policies for the domain.
*
*/
public Output accessPolicies() {
return this.accessPolicies;
}
/**
* Key-value string pairs to specify advanced configuration options. Note that the values for these configuration options must be strings (wrapped in quotes) or they may be wrong and cause a perpetual diff, causing the provider to want to recreate your Elasticsearch domain on every apply.
*
*/
@Export(name="advancedOptions", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy