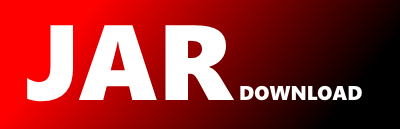
com.pulumi.aws.elastictranscoder.Pipeline Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elastictranscoder;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.elastictranscoder.PipelineArgs;
import com.pulumi.aws.elastictranscoder.inputs.PipelineState;
import com.pulumi.aws.elastictranscoder.outputs.PipelineContentConfig;
import com.pulumi.aws.elastictranscoder.outputs.PipelineContentConfigPermission;
import com.pulumi.aws.elastictranscoder.outputs.PipelineNotifications;
import com.pulumi.aws.elastictranscoder.outputs.PipelineThumbnailConfig;
import com.pulumi.aws.elastictranscoder.outputs.PipelineThumbnailConfigPermission;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Elastic Transcoder pipeline resource.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elastictranscoder.Pipeline;
* import com.pulumi.aws.elastictranscoder.PipelineArgs;
* import com.pulumi.aws.elastictranscoder.inputs.PipelineContentConfigArgs;
* import com.pulumi.aws.elastictranscoder.inputs.PipelineThumbnailConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var bar = new Pipeline("bar", PipelineArgs.builder()
* .inputBucket(inputBucket.id())
* .name("aws_elastictranscoder_pipeline_my_test_")
* .role(testRole.arn())
* .contentConfig(PipelineContentConfigArgs.builder()
* .bucket(contentBucket.id())
* .storageClass("Standard")
* .build())
* .thumbnailConfig(PipelineThumbnailConfigArgs.builder()
* .bucket(thumbBucket.id())
* .storageClass("Standard")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Elastic Transcoder pipelines using the `id`. For example:
*
* ```sh
* $ pulumi import aws:elastictranscoder/pipeline:Pipeline basic_pipeline 1407981661351-cttk8b
* ```
*
*/
@ResourceType(type="aws:elastictranscoder/pipeline:Pipeline")
public class Pipeline extends com.pulumi.resources.CustomResource {
/**
* The ARN of the Elastictranscoder pipeline.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the Elastictranscoder pipeline.
*
*/
public Output arn() {
return this.arn;
}
/**
* The AWS Key Management Service (AWS KMS) key that you want to use with this pipeline.
*
*/
@Export(name="awsKmsKeyArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> awsKmsKeyArn;
/**
* @return The AWS Key Management Service (AWS KMS) key that you want to use with this pipeline.
*
*/
public Output> awsKmsKeyArn() {
return Codegen.optional(this.awsKmsKeyArn);
}
/**
* The ContentConfig object specifies information about the Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and playlists. (documented below)
*
*/
@Export(name="contentConfig", refs={PipelineContentConfig.class}, tree="[0]")
private Output contentConfig;
/**
* @return The ContentConfig object specifies information about the Amazon S3 bucket in which you want Elastic Transcoder to save transcoded files and playlists. (documented below)
*
*/
public Output contentConfig() {
return this.contentConfig;
}
/**
* The permissions for the `content_config` object. (documented below)
*
*/
@Export(name="contentConfigPermissions", refs={List.class,PipelineContentConfigPermission.class}, tree="[0,1]")
private Output* @Nullable */ List> contentConfigPermissions;
/**
* @return The permissions for the `content_config` object. (documented below)
*
*/
public Output>> contentConfigPermissions() {
return Codegen.optional(this.contentConfigPermissions);
}
/**
* The Amazon S3 bucket in which you saved the media files that you want to transcode and the graphics that you want to use as watermarks.
*
*/
@Export(name="inputBucket", refs={String.class}, tree="[0]")
private Output inputBucket;
/**
* @return The Amazon S3 bucket in which you saved the media files that you want to transcode and the graphics that you want to use as watermarks.
*
*/
public Output inputBucket() {
return this.inputBucket;
}
/**
* The name of the pipeline. Maximum 40 characters
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the pipeline. Maximum 40 characters
*
*/
public Output name() {
return this.name;
}
/**
* The Amazon Simple Notification Service (Amazon SNS) topic that you want to notify to report job status. (documented below)
*
*/
@Export(name="notifications", refs={PipelineNotifications.class}, tree="[0]")
private Output* @Nullable */ PipelineNotifications> notifications;
/**
* @return The Amazon Simple Notification Service (Amazon SNS) topic that you want to notify to report job status. (documented below)
*
*/
public Output> notifications() {
return Codegen.optional(this.notifications);
}
/**
* The Amazon S3 bucket in which you want Elastic Transcoder to save the transcoded files.
*
*/
@Export(name="outputBucket", refs={String.class}, tree="[0]")
private Output outputBucket;
/**
* @return The Amazon S3 bucket in which you want Elastic Transcoder to save the transcoded files.
*
*/
public Output outputBucket() {
return this.outputBucket;
}
/**
* The IAM Amazon Resource Name (ARN) for the role that you want Elastic Transcoder to use to transcode jobs for this pipeline.
*
*/
@Export(name="role", refs={String.class}, tree="[0]")
private Output role;
/**
* @return The IAM Amazon Resource Name (ARN) for the role that you want Elastic Transcoder to use to transcode jobs for this pipeline.
*
*/
public Output role() {
return this.role;
}
/**
* The ThumbnailConfig object specifies information about the Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files. (documented below)
*
*/
@Export(name="thumbnailConfig", refs={PipelineThumbnailConfig.class}, tree="[0]")
private Output thumbnailConfig;
/**
* @return The ThumbnailConfig object specifies information about the Amazon S3 bucket in which you want Elastic Transcoder to save thumbnail files. (documented below)
*
*/
public Output thumbnailConfig() {
return this.thumbnailConfig;
}
/**
* The permissions for the `thumbnail_config` object. (documented below)
*
* The `content_config` object specifies information about the Amazon S3 bucket in
* which you want Elastic Transcoder to save transcoded files and playlists: which
* bucket to use, and the storage class that you want to assign to the files. If
* you specify values for `content_config`, you must also specify values for
* `thumbnail_config`. If you specify values for `content_config` and
* `thumbnail_config`, omit the `output_bucket` object.
*
*/
@Export(name="thumbnailConfigPermissions", refs={List.class,PipelineThumbnailConfigPermission.class}, tree="[0,1]")
private Output* @Nullable */ List> thumbnailConfigPermissions;
/**
* @return The permissions for the `thumbnail_config` object. (documented below)
*
* The `content_config` object specifies information about the Amazon S3 bucket in
* which you want Elastic Transcoder to save transcoded files and playlists: which
* bucket to use, and the storage class that you want to assign to the files. If
* you specify values for `content_config`, you must also specify values for
* `thumbnail_config`. If you specify values for `content_config` and
* `thumbnail_config`, omit the `output_bucket` object.
*
*/
public Output>> thumbnailConfigPermissions() {
return Codegen.optional(this.thumbnailConfigPermissions);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Pipeline(java.lang.String name) {
this(name, PipelineArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Pipeline(java.lang.String name, PipelineArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Pipeline(java.lang.String name, PipelineArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:elastictranscoder/pipeline:Pipeline", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Pipeline(java.lang.String name, Output id, @Nullable PipelineState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:elastictranscoder/pipeline:Pipeline", name, state, makeResourceOptions(options, id), false);
}
private static PipelineArgs makeArgs(PipelineArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? PipelineArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Pipeline get(java.lang.String name, Output id, @Nullable PipelineState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Pipeline(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy