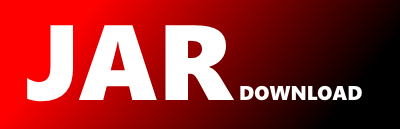
com.pulumi.aws.evidently.Launch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.evidently;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.evidently.LaunchArgs;
import com.pulumi.aws.evidently.inputs.LaunchState;
import com.pulumi.aws.evidently.outputs.LaunchExecution;
import com.pulumi.aws.evidently.outputs.LaunchGroup;
import com.pulumi.aws.evidently.outputs.LaunchMetricMonitor;
import com.pulumi.aws.evidently.outputs.LaunchScheduledSplitsConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a CloudWatch Evidently Launch resource.
*
* ## Example Usage
*
* ### Basic
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .groups(LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.of("Variation1", 0))
* .startTime("2024-01-07 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With description
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .description("example description")
* .groups(LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.of("Variation1", 0))
* .startTime("2024-01-07 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With multiple groups
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .groups(
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .description("first-group")
* .build(),
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation2")
* .variation("Variation2")
* .description("second-group")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.ofEntries(
* Map.entry("Variation1", 0),
* Map.entry("Variation2", 0)
* ))
* .startTime("2024-01-07 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With metric_monitors
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchMetricMonitorArgs;
* import com.pulumi.aws.evidently.inputs.LaunchMetricMonitorMetricDefinitionArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .groups(LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build())
* .metricMonitors(
* LaunchMetricMonitorArgs.builder()
* .metricDefinition(LaunchMetricMonitorMetricDefinitionArgs.builder()
* .entityIdKey("entity_id_key1")
* .eventPattern("{\"Price\":[{\"numeric\":[\">\",11,\"<=\",22]}]}")
* .name("name1")
* .unitLabel("unit_label1")
* .valueKey("value_key1")
* .build())
* .build(),
* LaunchMetricMonitorArgs.builder()
* .metricDefinition(LaunchMetricMonitorMetricDefinitionArgs.builder()
* .entityIdKey("entity_id_key2")
* .eventPattern("{\"Price\":[{\"numeric\":[\">\",9,\"<=\",19]}]}")
* .name("name2")
* .unitLabel("unit_label2")
* .valueKey("value_key2")
* .build())
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.of("Variation1", 0))
* .startTime("2024-01-07 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With randomization_salt
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .randomizationSalt("example randomization salt")
* .groups(LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.of("Variation1", 0))
* .startTime("2024-01-07 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With multiple steps
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .groups(
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build(),
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation2")
* .variation("Variation2")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(
* LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.ofEntries(
* Map.entry("Variation1", 15),
* Map.entry("Variation2", 10)
* ))
* .startTime("2024-01-07 01:43:59+00:00")
* .build(),
* LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.ofEntries(
* Map.entry("Variation1", 20),
* Map.entry("Variation2", 25)
* ))
* .startTime("2024-01-08 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### With segment overrides
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.evidently.Launch;
* import com.pulumi.aws.evidently.LaunchArgs;
* import com.pulumi.aws.evidently.inputs.LaunchGroupArgs;
* import com.pulumi.aws.evidently.inputs.LaunchScheduledSplitsConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Launch("example", LaunchArgs.builder()
* .name("example")
* .project(exampleAwsEvidentlyProject.name())
* .groups(
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation1")
* .variation("Variation1")
* .build(),
* LaunchGroupArgs.builder()
* .feature(exampleAwsEvidentlyFeature.name())
* .name("Variation2")
* .variation("Variation2")
* .build())
* .scheduledSplitsConfig(LaunchScheduledSplitsConfigArgs.builder()
* .steps(LaunchScheduledSplitsConfigStepArgs.builder()
* .groupWeights(Map.ofEntries(
* Map.entry("Variation1", 0),
* Map.entry("Variation2", 0)
* ))
* .segmentOverrides(
* LaunchScheduledSplitsConfigStepSegmentOverrideArgs.builder()
* .evaluationOrder(1)
* .segment(exampleAwsEvidentlySegment.name())
* .weights(Map.of("Variation2", 10000))
* .build(),
* LaunchScheduledSplitsConfigStepSegmentOverrideArgs.builder()
* .evaluationOrder(2)
* .segment(exampleAwsEvidentlySegment.name())
* .weights(Map.ofEntries(
* Map.entry("Variation1", 40000),
* Map.entry("Variation2", 30000)
* ))
* .build())
* .startTime("2024-01-08 01:43:59+00:00")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Import using the `name` of the launch and `arn` of the project separated by a `:`:
*
* __Using `pulumi import` to import__ CloudWatch Evidently Launch using the `name` of the launch and `name` of the project or `arn` of the hosting CloudWatch Evidently Project separated by a `:`. For example:
*
* Import using the `name` of the launch and `name` of the project separated by a `:`:
*
* ```sh
* $ pulumi import aws:evidently/launch:Launch example exampleLaunchName:exampleProjectName
* ```
* Import using the `name` of the launch and `arn` of the project separated by a `:`:
*
* ```sh
* $ pulumi import aws:evidently/launch:Launch example exampleLaunchName:arn:aws:evidently:us-east-1:123456789012:project/exampleProjectName
* ```
*
*/
@ResourceType(type="aws:evidently/launch:Launch")
public class Launch extends com.pulumi.resources.CustomResource {
/**
* The ARN of the launch.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The ARN of the launch.
*
*/
public Output arn() {
return this.arn;
}
/**
* The date and time that the launch is created.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return The date and time that the launch is created.
*
*/
public Output createdTime() {
return this.createdTime;
}
/**
* Specifies the description of the launch.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Specifies the description of the launch.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* A block that contains information about the start and end times of the launch. Detailed below
*
*/
@Export(name="executions", refs={List.class,LaunchExecution.class}, tree="[0,1]")
private Output> executions;
/**
* @return A block that contains information about the start and end times of the launch. Detailed below
*
*/
public Output> executions() {
return this.executions;
}
/**
* One or up to five blocks that contain the feature and variations that are to be used for the launch. Detailed below.
*
*/
@Export(name="groups", refs={List.class,LaunchGroup.class}, tree="[0,1]")
private Output> groups;
/**
* @return One or up to five blocks that contain the feature and variations that are to be used for the launch. Detailed below.
*
*/
public Output> groups() {
return this.groups;
}
/**
* The date and time that the launch was most recently updated.
*
*/
@Export(name="lastUpdatedTime", refs={String.class}, tree="[0]")
private Output lastUpdatedTime;
/**
* @return The date and time that the launch was most recently updated.
*
*/
public Output lastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
* One or up to three blocks that define the metrics that will be used to monitor the launch performance. Detailed below.
*
*/
@Export(name="metricMonitors", refs={List.class,LaunchMetricMonitor.class}, tree="[0,1]")
private Output* @Nullable */ List> metricMonitors;
/**
* @return One or up to three blocks that define the metrics that will be used to monitor the launch performance. Detailed below.
*
*/
public Output>> metricMonitors() {
return Codegen.optional(this.metricMonitors);
}
/**
* The name for the new launch. Minimum length of `1`. Maximum length of `127`.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name for the new launch. Minimum length of `1`. Maximum length of `127`.
*
*/
public Output name() {
return this.name;
}
/**
* The name or ARN of the project that is to contain the new launch.
*
*/
@Export(name="project", refs={String.class}, tree="[0]")
private Output project;
/**
* @return The name or ARN of the project that is to contain the new launch.
*
*/
public Output project() {
return this.project;
}
/**
* When Evidently assigns a particular user session to a launch, it must use a randomization ID to determine which variation the user session is served. This randomization ID is a combination of the entity ID and randomizationSalt. If you omit randomizationSalt, Evidently uses the launch name as the randomizationSalt.
*
*/
@Export(name="randomizationSalt", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> randomizationSalt;
/**
* @return When Evidently assigns a particular user session to a launch, it must use a randomization ID to determine which variation the user session is served. This randomization ID is a combination of the entity ID and randomizationSalt. If you omit randomizationSalt, Evidently uses the launch name as the randomizationSalt.
*
*/
public Output> randomizationSalt() {
return Codegen.optional(this.randomizationSalt);
}
/**
* A block that defines the traffic allocation percentages among the feature variations during each step of the launch. Detailed below.
*
*/
@Export(name="scheduledSplitsConfig", refs={LaunchScheduledSplitsConfig.class}, tree="[0]")
private Output* @Nullable */ LaunchScheduledSplitsConfig> scheduledSplitsConfig;
/**
* @return A block that defines the traffic allocation percentages among the feature variations during each step of the launch. Detailed below.
*
*/
public Output> scheduledSplitsConfig() {
return Codegen.optional(this.scheduledSplitsConfig);
}
/**
* The current state of the launch. Valid values are `CREATED`, `UPDATING`, `RUNNING`, `COMPLETED`, and `CANCELLED`.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The current state of the launch. Valid values are `CREATED`, `UPDATING`, `RUNNING`, `COMPLETED`, and `CANCELLED`.
*
*/
public Output status() {
return this.status;
}
/**
* If the launch was stopped, this is the string that was entered by the person who stopped the launch, to explain why it was stopped.
*
*/
@Export(name="statusReason", refs={String.class}, tree="[0]")
private Output statusReason;
/**
* @return If the launch was stopped, this is the string that was entered by the person who stopped the launch, to explain why it was stopped.
*
*/
public Output statusReason() {
return this.statusReason;
}
/**
* Tags to apply to the launch. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Tags to apply to the launch. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy