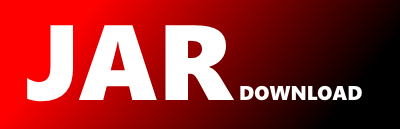
com.pulumi.aws.iam.inputs.GetPolicyDocumentStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iam.inputs;
import com.pulumi.aws.iam.inputs.GetPolicyDocumentStatementCondition;
import com.pulumi.aws.iam.inputs.GetPolicyDocumentStatementNotPrincipal;
import com.pulumi.aws.iam.inputs.GetPolicyDocumentStatementPrincipal;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetPolicyDocumentStatement extends com.pulumi.resources.InvokeArgs {
public static final GetPolicyDocumentStatement Empty = new GetPolicyDocumentStatement();
/**
* List of actions that this statement either allows or denies. For example, `["ec2:RunInstances", "s3:*"]`.
*
*/
@Import(name="actions")
private @Nullable List actions;
/**
* @return List of actions that this statement either allows or denies. For example, `["ec2:RunInstances", "s3:*"]`.
*
*/
public Optional> actions() {
return Optional.ofNullable(this.actions);
}
/**
* Configuration block for a condition. Detailed below.
*
*/
@Import(name="conditions")
private @Nullable List conditions;
/**
* @return Configuration block for a condition. Detailed below.
*
*/
public Optional> conditions() {
return Optional.ofNullable(this.conditions);
}
/**
* Whether this statement allows or denies the given actions. Valid values are `Allow` and `Deny`. Defaults to `Allow`.
*
*/
@Import(name="effect")
private @Nullable String effect;
/**
* @return Whether this statement allows or denies the given actions. Valid values are `Allow` and `Deny`. Defaults to `Allow`.
*
*/
public Optional effect() {
return Optional.ofNullable(this.effect);
}
/**
* List of actions that this statement does *not* apply to. Use to apply a policy statement to all actions *except* those listed.
*
*/
@Import(name="notActions")
private @Nullable List notActions;
/**
* @return List of actions that this statement does *not* apply to. Use to apply a policy statement to all actions *except* those listed.
*
*/
public Optional> notActions() {
return Optional.ofNullable(this.notActions);
}
/**
* Like `principals` except these are principals that the statement does *not* apply to.
*
*/
@Import(name="notPrincipals")
private @Nullable List notPrincipals;
/**
* @return Like `principals` except these are principals that the statement does *not* apply to.
*
*/
public Optional> notPrincipals() {
return Optional.ofNullable(this.notPrincipals);
}
/**
* List of resource ARNs that this statement does *not* apply to. Use to apply a policy statement to all resources *except* those listed. Conflicts with `resources`.
*
*/
@Import(name="notResources")
private @Nullable List notResources;
/**
* @return List of resource ARNs that this statement does *not* apply to. Use to apply a policy statement to all resources *except* those listed. Conflicts with `resources`.
*
*/
public Optional> notResources() {
return Optional.ofNullable(this.notResources);
}
/**
* Configuration block for principals. Detailed below.
*
*/
@Import(name="principals")
private @Nullable List principals;
/**
* @return Configuration block for principals. Detailed below.
*
*/
public Optional> principals() {
return Optional.ofNullable(this.principals);
}
/**
* List of resource ARNs that this statement applies to. This is required by AWS if used for an IAM policy. Conflicts with `not_resources`.
*
*/
@Import(name="resources")
private @Nullable List resources;
/**
* @return List of resource ARNs that this statement applies to. This is required by AWS if used for an IAM policy. Conflicts with `not_resources`.
*
*/
public Optional> resources() {
return Optional.ofNullable(this.resources);
}
/**
* Sid (statement ID) is an identifier for a policy statement.
*
*/
@Import(name="sid")
private @Nullable String sid;
/**
* @return Sid (statement ID) is an identifier for a policy statement.
*
*/
public Optional sid() {
return Optional.ofNullable(this.sid);
}
private GetPolicyDocumentStatement() {}
private GetPolicyDocumentStatement(GetPolicyDocumentStatement $) {
this.actions = $.actions;
this.conditions = $.conditions;
this.effect = $.effect;
this.notActions = $.notActions;
this.notPrincipals = $.notPrincipals;
this.notResources = $.notResources;
this.principals = $.principals;
this.resources = $.resources;
this.sid = $.sid;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPolicyDocumentStatement defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetPolicyDocumentStatement $;
public Builder() {
$ = new GetPolicyDocumentStatement();
}
public Builder(GetPolicyDocumentStatement defaults) {
$ = new GetPolicyDocumentStatement(Objects.requireNonNull(defaults));
}
/**
* @param actions List of actions that this statement either allows or denies. For example, `["ec2:RunInstances", "s3:*"]`.
*
* @return builder
*
*/
public Builder actions(@Nullable List actions) {
$.actions = actions;
return this;
}
/**
* @param actions List of actions that this statement either allows or denies. For example, `["ec2:RunInstances", "s3:*"]`.
*
* @return builder
*
*/
public Builder actions(String... actions) {
return actions(List.of(actions));
}
/**
* @param conditions Configuration block for a condition. Detailed below.
*
* @return builder
*
*/
public Builder conditions(@Nullable List conditions) {
$.conditions = conditions;
return this;
}
/**
* @param conditions Configuration block for a condition. Detailed below.
*
* @return builder
*
*/
public Builder conditions(GetPolicyDocumentStatementCondition... conditions) {
return conditions(List.of(conditions));
}
/**
* @param effect Whether this statement allows or denies the given actions. Valid values are `Allow` and `Deny`. Defaults to `Allow`.
*
* @return builder
*
*/
public Builder effect(@Nullable String effect) {
$.effect = effect;
return this;
}
/**
* @param notActions List of actions that this statement does *not* apply to. Use to apply a policy statement to all actions *except* those listed.
*
* @return builder
*
*/
public Builder notActions(@Nullable List notActions) {
$.notActions = notActions;
return this;
}
/**
* @param notActions List of actions that this statement does *not* apply to. Use to apply a policy statement to all actions *except* those listed.
*
* @return builder
*
*/
public Builder notActions(String... notActions) {
return notActions(List.of(notActions));
}
/**
* @param notPrincipals Like `principals` except these are principals that the statement does *not* apply to.
*
* @return builder
*
*/
public Builder notPrincipals(@Nullable List notPrincipals) {
$.notPrincipals = notPrincipals;
return this;
}
/**
* @param notPrincipals Like `principals` except these are principals that the statement does *not* apply to.
*
* @return builder
*
*/
public Builder notPrincipals(GetPolicyDocumentStatementNotPrincipal... notPrincipals) {
return notPrincipals(List.of(notPrincipals));
}
/**
* @param notResources List of resource ARNs that this statement does *not* apply to. Use to apply a policy statement to all resources *except* those listed. Conflicts with `resources`.
*
* @return builder
*
*/
public Builder notResources(@Nullable List notResources) {
$.notResources = notResources;
return this;
}
/**
* @param notResources List of resource ARNs that this statement does *not* apply to. Use to apply a policy statement to all resources *except* those listed. Conflicts with `resources`.
*
* @return builder
*
*/
public Builder notResources(String... notResources) {
return notResources(List.of(notResources));
}
/**
* @param principals Configuration block for principals. Detailed below.
*
* @return builder
*
*/
public Builder principals(@Nullable List principals) {
$.principals = principals;
return this;
}
/**
* @param principals Configuration block for principals. Detailed below.
*
* @return builder
*
*/
public Builder principals(GetPolicyDocumentStatementPrincipal... principals) {
return principals(List.of(principals));
}
/**
* @param resources List of resource ARNs that this statement applies to. This is required by AWS if used for an IAM policy. Conflicts with `not_resources`.
*
* @return builder
*
*/
public Builder resources(@Nullable List resources) {
$.resources = resources;
return this;
}
/**
* @param resources List of resource ARNs that this statement applies to. This is required by AWS if used for an IAM policy. Conflicts with `not_resources`.
*
* @return builder
*
*/
public Builder resources(String... resources) {
return resources(List.of(resources));
}
/**
* @param sid Sid (statement ID) is an identifier for a policy statement.
*
* @return builder
*
*/
public Builder sid(@Nullable String sid) {
$.sid = sid;
return this;
}
public GetPolicyDocumentStatement build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy