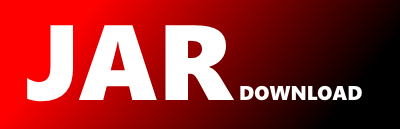
com.pulumi.aws.inputs.GetServicePlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.inputs;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetServicePlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetServicePlainArgs Empty = new GetServicePlainArgs();
/**
* DNS name of the service (_e.g.,_ `rds.us-east-1.amazonaws.com`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
*/
@Import(name="dnsName")
private @Nullable String dnsName;
/**
* @return DNS name of the service (_e.g.,_ `rds.us-east-1.amazonaws.com`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
*/
public Optional dnsName() {
return Optional.ofNullable(this.dnsName);
}
@Import(name="id")
private @Nullable String id;
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* Region of the service (_e.g.,_ `us-west-2`, `ap-northeast-1`).
*
*/
@Import(name="region")
private @Nullable String region;
/**
* @return Region of the service (_e.g.,_ `us-west-2`, `ap-northeast-1`).
*
*/
public Optional region() {
return Optional.ofNullable(this.region);
}
/**
* Reverse DNS name of the service (_e.g.,_ `com.amazonaws.us-west-2.s3`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
*/
@Import(name="reverseDnsName")
private @Nullable String reverseDnsName;
/**
* @return Reverse DNS name of the service (_e.g.,_ `com.amazonaws.us-west-2.s3`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
*/
public Optional reverseDnsName() {
return Optional.ofNullable(this.reverseDnsName);
}
/**
* Prefix of the service (_e.g.,_ `com.amazonaws` in AWS Commercial, `cn.com.amazonaws` in AWS China).
*
*/
@Import(name="reverseDnsPrefix")
private @Nullable String reverseDnsPrefix;
/**
* @return Prefix of the service (_e.g.,_ `com.amazonaws` in AWS Commercial, `cn.com.amazonaws` in AWS China).
*
*/
public Optional reverseDnsPrefix() {
return Optional.ofNullable(this.reverseDnsPrefix);
}
/**
* Service endpoint ID (_e.g.,_ `s3`, `rds`, `ec2`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required. A service's endpoint ID can be found in the [_AWS General Reference_](https://docs.aws.amazon.com/general/latest/gr/aws-service-information.html).
*
*/
@Import(name="serviceId")
private @Nullable String serviceId;
/**
* @return Service endpoint ID (_e.g.,_ `s3`, `rds`, `ec2`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required. A service's endpoint ID can be found in the [_AWS General Reference_](https://docs.aws.amazon.com/general/latest/gr/aws-service-information.html).
*
*/
public Optional serviceId() {
return Optional.ofNullable(this.serviceId);
}
private GetServicePlainArgs() {}
private GetServicePlainArgs(GetServicePlainArgs $) {
this.dnsName = $.dnsName;
this.id = $.id;
this.region = $.region;
this.reverseDnsName = $.reverseDnsName;
this.reverseDnsPrefix = $.reverseDnsPrefix;
this.serviceId = $.serviceId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServicePlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetServicePlainArgs $;
public Builder() {
$ = new GetServicePlainArgs();
}
public Builder(GetServicePlainArgs defaults) {
$ = new GetServicePlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param dnsName DNS name of the service (_e.g.,_ `rds.us-east-1.amazonaws.com`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
* @return builder
*
*/
public Builder dnsName(@Nullable String dnsName) {
$.dnsName = dnsName;
return this;
}
public Builder id(@Nullable String id) {
$.id = id;
return this;
}
/**
* @param region Region of the service (_e.g.,_ `us-west-2`, `ap-northeast-1`).
*
* @return builder
*
*/
public Builder region(@Nullable String region) {
$.region = region;
return this;
}
/**
* @param reverseDnsName Reverse DNS name of the service (_e.g.,_ `com.amazonaws.us-west-2.s3`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*
* @return builder
*
*/
public Builder reverseDnsName(@Nullable String reverseDnsName) {
$.reverseDnsName = reverseDnsName;
return this;
}
/**
* @param reverseDnsPrefix Prefix of the service (_e.g.,_ `com.amazonaws` in AWS Commercial, `cn.com.amazonaws` in AWS China).
*
* @return builder
*
*/
public Builder reverseDnsPrefix(@Nullable String reverseDnsPrefix) {
$.reverseDnsPrefix = reverseDnsPrefix;
return this;
}
/**
* @param serviceId Service endpoint ID (_e.g.,_ `s3`, `rds`, `ec2`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required. A service's endpoint ID can be found in the [_AWS General Reference_](https://docs.aws.amazon.com/general/latest/gr/aws-service-information.html).
*
* @return builder
*
*/
public Builder serviceId(@Nullable String serviceId) {
$.serviceId = serviceId;
return this;
}
public GetServicePlainArgs build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy