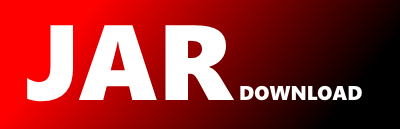
com.pulumi.aws.keyspaces.inputs.TableState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.keyspaces.inputs;
import com.pulumi.aws.keyspaces.inputs.TableCapacitySpecificationArgs;
import com.pulumi.aws.keyspaces.inputs.TableClientSideTimestampsArgs;
import com.pulumi.aws.keyspaces.inputs.TableCommentArgs;
import com.pulumi.aws.keyspaces.inputs.TableEncryptionSpecificationArgs;
import com.pulumi.aws.keyspaces.inputs.TablePointInTimeRecoveryArgs;
import com.pulumi.aws.keyspaces.inputs.TableSchemaDefinitionArgs;
import com.pulumi.aws.keyspaces.inputs.TableTtlArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TableState extends com.pulumi.resources.ResourceArgs {
public static final TableState Empty = new TableState();
/**
* The ARN of the table.
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return The ARN of the table.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy