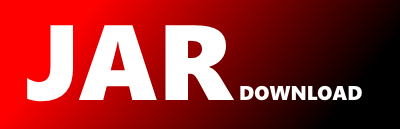
com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamElasticsearchConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.kinesis.outputs;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamElasticsearchConfigurationCloudwatchLoggingOptions;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamElasticsearchConfigurationProcessingConfiguration;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamElasticsearchConfigurationS3Configuration;
import com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamElasticsearchConfigurationVpcConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FirehoseDeliveryStreamElasticsearchConfiguration {
/**
* @return Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
*
*/
private @Nullable Integer bufferingInterval;
/**
* @return Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
*
*/
private @Nullable Integer bufferingSize;
/**
* @return The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions;
/**
* @return The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
*
*/
private @Nullable String clusterEndpoint;
/**
* @return The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
*
*/
private @Nullable String domainArn;
/**
* @return The Elasticsearch index name.
*
*/
private String indexName;
/**
* @return The Elasticsearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
*
*/
private @Nullable String indexRotationPeriod;
/**
* @return The data processing configuration. See `processing_configuration` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationProcessingConfiguration processingConfiguration;
/**
* @return After an initial failure to deliver to Amazon Elasticsearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
*
*/
private @Nullable Integer retryDuration;
/**
* @return The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeElasticsearchDomain`, `DescribeElasticsearchDomains`, and `DescribeElasticsearchDomainConfig`. The pattern needs to be `arn:.*`.
*
*/
private String roleArn;
/**
* @return Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
*
*/
private @Nullable String s3BackupMode;
/**
* @return The S3 Configuration. See `s3_configuration` block below for details.
*
*/
private FirehoseDeliveryStreamElasticsearchConfigurationS3Configuration s3Configuration;
/**
* @return The Elasticsearch type name with maximum length of 100 characters.
*
*/
private @Nullable String typeName;
/**
* @return The VPC configuration for the delivery stream to connect to Elastic Search associated with the VPC. See `vpc_config` block below for details.
*
*/
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationVpcConfig vpcConfig;
private FirehoseDeliveryStreamElasticsearchConfiguration() {}
/**
* @return Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
*
*/
public Optional bufferingInterval() {
return Optional.ofNullable(this.bufferingInterval);
}
/**
* @return Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
*
*/
public Optional bufferingSize() {
return Optional.ofNullable(this.bufferingSize);
}
/**
* @return The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*
*/
public Optional cloudwatchLoggingOptions() {
return Optional.ofNullable(this.cloudwatchLoggingOptions);
}
/**
* @return The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
*
*/
public Optional clusterEndpoint() {
return Optional.ofNullable(this.clusterEndpoint);
}
/**
* @return The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
*
*/
public Optional domainArn() {
return Optional.ofNullable(this.domainArn);
}
/**
* @return The Elasticsearch index name.
*
*/
public String indexName() {
return this.indexName;
}
/**
* @return The Elasticsearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
*
*/
public Optional indexRotationPeriod() {
return Optional.ofNullable(this.indexRotationPeriod);
}
/**
* @return The data processing configuration. See `processing_configuration` block below for details.
*
*/
public Optional processingConfiguration() {
return Optional.ofNullable(this.processingConfiguration);
}
/**
* @return After an initial failure to deliver to Amazon Elasticsearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
*
*/
public Optional retryDuration() {
return Optional.ofNullable(this.retryDuration);
}
/**
* @return The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeElasticsearchDomain`, `DescribeElasticsearchDomains`, and `DescribeElasticsearchDomainConfig`. The pattern needs to be `arn:.*`.
*
*/
public String roleArn() {
return this.roleArn;
}
/**
* @return Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
*
*/
public Optional s3BackupMode() {
return Optional.ofNullable(this.s3BackupMode);
}
/**
* @return The S3 Configuration. See `s3_configuration` block below for details.
*
*/
public FirehoseDeliveryStreamElasticsearchConfigurationS3Configuration s3Configuration() {
return this.s3Configuration;
}
/**
* @return The Elasticsearch type name with maximum length of 100 characters.
*
*/
public Optional typeName() {
return Optional.ofNullable(this.typeName);
}
/**
* @return The VPC configuration for the delivery stream to connect to Elastic Search associated with the VPC. See `vpc_config` block below for details.
*
*/
public Optional vpcConfig() {
return Optional.ofNullable(this.vpcConfig);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FirehoseDeliveryStreamElasticsearchConfiguration defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer bufferingInterval;
private @Nullable Integer bufferingSize;
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions;
private @Nullable String clusterEndpoint;
private @Nullable String domainArn;
private String indexName;
private @Nullable String indexRotationPeriod;
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationProcessingConfiguration processingConfiguration;
private @Nullable Integer retryDuration;
private String roleArn;
private @Nullable String s3BackupMode;
private FirehoseDeliveryStreamElasticsearchConfigurationS3Configuration s3Configuration;
private @Nullable String typeName;
private @Nullable FirehoseDeliveryStreamElasticsearchConfigurationVpcConfig vpcConfig;
public Builder() {}
public Builder(FirehoseDeliveryStreamElasticsearchConfiguration defaults) {
Objects.requireNonNull(defaults);
this.bufferingInterval = defaults.bufferingInterval;
this.bufferingSize = defaults.bufferingSize;
this.cloudwatchLoggingOptions = defaults.cloudwatchLoggingOptions;
this.clusterEndpoint = defaults.clusterEndpoint;
this.domainArn = defaults.domainArn;
this.indexName = defaults.indexName;
this.indexRotationPeriod = defaults.indexRotationPeriod;
this.processingConfiguration = defaults.processingConfiguration;
this.retryDuration = defaults.retryDuration;
this.roleArn = defaults.roleArn;
this.s3BackupMode = defaults.s3BackupMode;
this.s3Configuration = defaults.s3Configuration;
this.typeName = defaults.typeName;
this.vpcConfig = defaults.vpcConfig;
}
@CustomType.Setter
public Builder bufferingInterval(@Nullable Integer bufferingInterval) {
this.bufferingInterval = bufferingInterval;
return this;
}
@CustomType.Setter
public Builder bufferingSize(@Nullable Integer bufferingSize) {
this.bufferingSize = bufferingSize;
return this;
}
@CustomType.Setter
public Builder cloudwatchLoggingOptions(@Nullable FirehoseDeliveryStreamElasticsearchConfigurationCloudwatchLoggingOptions cloudwatchLoggingOptions) {
this.cloudwatchLoggingOptions = cloudwatchLoggingOptions;
return this;
}
@CustomType.Setter
public Builder clusterEndpoint(@Nullable String clusterEndpoint) {
this.clusterEndpoint = clusterEndpoint;
return this;
}
@CustomType.Setter
public Builder domainArn(@Nullable String domainArn) {
this.domainArn = domainArn;
return this;
}
@CustomType.Setter
public Builder indexName(String indexName) {
if (indexName == null) {
throw new MissingRequiredPropertyException("FirehoseDeliveryStreamElasticsearchConfiguration", "indexName");
}
this.indexName = indexName;
return this;
}
@CustomType.Setter
public Builder indexRotationPeriod(@Nullable String indexRotationPeriod) {
this.indexRotationPeriod = indexRotationPeriod;
return this;
}
@CustomType.Setter
public Builder processingConfiguration(@Nullable FirehoseDeliveryStreamElasticsearchConfigurationProcessingConfiguration processingConfiguration) {
this.processingConfiguration = processingConfiguration;
return this;
}
@CustomType.Setter
public Builder retryDuration(@Nullable Integer retryDuration) {
this.retryDuration = retryDuration;
return this;
}
@CustomType.Setter
public Builder roleArn(String roleArn) {
if (roleArn == null) {
throw new MissingRequiredPropertyException("FirehoseDeliveryStreamElasticsearchConfiguration", "roleArn");
}
this.roleArn = roleArn;
return this;
}
@CustomType.Setter
public Builder s3BackupMode(@Nullable String s3BackupMode) {
this.s3BackupMode = s3BackupMode;
return this;
}
@CustomType.Setter
public Builder s3Configuration(FirehoseDeliveryStreamElasticsearchConfigurationS3Configuration s3Configuration) {
if (s3Configuration == null) {
throw new MissingRequiredPropertyException("FirehoseDeliveryStreamElasticsearchConfiguration", "s3Configuration");
}
this.s3Configuration = s3Configuration;
return this;
}
@CustomType.Setter
public Builder typeName(@Nullable String typeName) {
this.typeName = typeName;
return this;
}
@CustomType.Setter
public Builder vpcConfig(@Nullable FirehoseDeliveryStreamElasticsearchConfigurationVpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
public FirehoseDeliveryStreamElasticsearchConfiguration build() {
final var _resultValue = new FirehoseDeliveryStreamElasticsearchConfiguration();
_resultValue.bufferingInterval = bufferingInterval;
_resultValue.bufferingSize = bufferingSize;
_resultValue.cloudwatchLoggingOptions = cloudwatchLoggingOptions;
_resultValue.clusterEndpoint = clusterEndpoint;
_resultValue.domainArn = domainArn;
_resultValue.indexName = indexName;
_resultValue.indexRotationPeriod = indexRotationPeriod;
_resultValue.processingConfiguration = processingConfiguration;
_resultValue.retryDuration = retryDuration;
_resultValue.roleArn = roleArn;
_resultValue.s3BackupMode = s3BackupMode;
_resultValue.s3Configuration = s3Configuration;
_resultValue.typeName = typeName;
_resultValue.vpcConfig = vpcConfig;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy