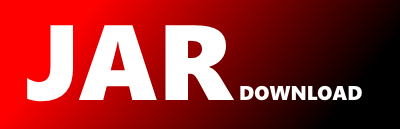
com.pulumi.aws.kms.outputs.GetPublicKeyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.kms.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetPublicKeyResult {
/**
* @return Key ARN of the asymmetric CMK from which the public key was downloaded.
*
*/
private String arn;
/**
* @return Type of the public key that was downloaded.
*
*/
private String customerMasterKeySpec;
/**
* @return Encryption algorithms that AWS KMS supports for this key. Only set when the `key_usage` of the public key is `ENCRYPT_DECRYPT`.
*
*/
private List encryptionAlgorithms;
private @Nullable List grantTokens;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String keyId;
/**
* @return Permitted use of the public key. Valid values are `ENCRYPT_DECRYPT` or `SIGN_VERIFY`
*
*/
private String keyUsage;
/**
* @return Exported public key. The value is a DER-encoded X.509 public key, also known as SubjectPublicKeyInfo (SPKI), as defined in [RFC 5280](https://tools.ietf.org/html/rfc5280). The value is Base64-encoded.
*
*/
private String publicKey;
/**
* @return Exported public key. The value is Privacy Enhanced Mail (PEM) encoded.
*
*/
private String publicKeyPem;
/**
* @return Signing algorithms that AWS KMS supports for this key. Only set when the `key_usage` of the public key is `SIGN_VERIFY`.
*
*/
private List signingAlgorithms;
private GetPublicKeyResult() {}
/**
* @return Key ARN of the asymmetric CMK from which the public key was downloaded.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Type of the public key that was downloaded.
*
*/
public String customerMasterKeySpec() {
return this.customerMasterKeySpec;
}
/**
* @return Encryption algorithms that AWS KMS supports for this key. Only set when the `key_usage` of the public key is `ENCRYPT_DECRYPT`.
*
*/
public List encryptionAlgorithms() {
return this.encryptionAlgorithms;
}
public List grantTokens() {
return this.grantTokens == null ? List.of() : this.grantTokens;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String keyId() {
return this.keyId;
}
/**
* @return Permitted use of the public key. Valid values are `ENCRYPT_DECRYPT` or `SIGN_VERIFY`
*
*/
public String keyUsage() {
return this.keyUsage;
}
/**
* @return Exported public key. The value is a DER-encoded X.509 public key, also known as SubjectPublicKeyInfo (SPKI), as defined in [RFC 5280](https://tools.ietf.org/html/rfc5280). The value is Base64-encoded.
*
*/
public String publicKey() {
return this.publicKey;
}
/**
* @return Exported public key. The value is Privacy Enhanced Mail (PEM) encoded.
*
*/
public String publicKeyPem() {
return this.publicKeyPem;
}
/**
* @return Signing algorithms that AWS KMS supports for this key. Only set when the `key_usage` of the public key is `SIGN_VERIFY`.
*
*/
public List signingAlgorithms() {
return this.signingAlgorithms;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPublicKeyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String customerMasterKeySpec;
private List encryptionAlgorithms;
private @Nullable List grantTokens;
private String id;
private String keyId;
private String keyUsage;
private String publicKey;
private String publicKeyPem;
private List signingAlgorithms;
public Builder() {}
public Builder(GetPublicKeyResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.customerMasterKeySpec = defaults.customerMasterKeySpec;
this.encryptionAlgorithms = defaults.encryptionAlgorithms;
this.grantTokens = defaults.grantTokens;
this.id = defaults.id;
this.keyId = defaults.keyId;
this.keyUsage = defaults.keyUsage;
this.publicKey = defaults.publicKey;
this.publicKeyPem = defaults.publicKeyPem;
this.signingAlgorithms = defaults.signingAlgorithms;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder customerMasterKeySpec(String customerMasterKeySpec) {
if (customerMasterKeySpec == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "customerMasterKeySpec");
}
this.customerMasterKeySpec = customerMasterKeySpec;
return this;
}
@CustomType.Setter
public Builder encryptionAlgorithms(List encryptionAlgorithms) {
if (encryptionAlgorithms == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "encryptionAlgorithms");
}
this.encryptionAlgorithms = encryptionAlgorithms;
return this;
}
public Builder encryptionAlgorithms(String... encryptionAlgorithms) {
return encryptionAlgorithms(List.of(encryptionAlgorithms));
}
@CustomType.Setter
public Builder grantTokens(@Nullable List grantTokens) {
this.grantTokens = grantTokens;
return this;
}
public Builder grantTokens(String... grantTokens) {
return grantTokens(List.of(grantTokens));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keyId(String keyId) {
if (keyId == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "keyId");
}
this.keyId = keyId;
return this;
}
@CustomType.Setter
public Builder keyUsage(String keyUsage) {
if (keyUsage == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "keyUsage");
}
this.keyUsage = keyUsage;
return this;
}
@CustomType.Setter
public Builder publicKey(String publicKey) {
if (publicKey == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "publicKey");
}
this.publicKey = publicKey;
return this;
}
@CustomType.Setter
public Builder publicKeyPem(String publicKeyPem) {
if (publicKeyPem == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "publicKeyPem");
}
this.publicKeyPem = publicKeyPem;
return this;
}
@CustomType.Setter
public Builder signingAlgorithms(List signingAlgorithms) {
if (signingAlgorithms == null) {
throw new MissingRequiredPropertyException("GetPublicKeyResult", "signingAlgorithms");
}
this.signingAlgorithms = signingAlgorithms;
return this;
}
public Builder signingAlgorithms(String... signingAlgorithms) {
return signingAlgorithms(List.of(signingAlgorithms));
}
public GetPublicKeyResult build() {
final var _resultValue = new GetPublicKeyResult();
_resultValue.arn = arn;
_resultValue.customerMasterKeySpec = customerMasterKeySpec;
_resultValue.encryptionAlgorithms = encryptionAlgorithms;
_resultValue.grantTokens = grantTokens;
_resultValue.id = id;
_resultValue.keyId = keyId;
_resultValue.keyUsage = keyUsage;
_resultValue.publicKey = publicKey;
_resultValue.publicKeyPem = publicKeyPem;
_resultValue.signingAlgorithms = signingAlgorithms;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy