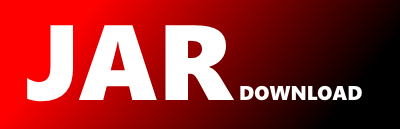
com.pulumi.aws.mskconnect.ConnectorArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.mskconnect;
import com.pulumi.aws.mskconnect.inputs.ConnectorCapacityArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorKafkaClusterArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorKafkaClusterClientAuthenticationArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorKafkaClusterEncryptionInTransitArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorLogDeliveryArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorPluginArgs;
import com.pulumi.aws.mskconnect.inputs.ConnectorWorkerConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ConnectorArgs extends com.pulumi.resources.ResourceArgs {
public static final ConnectorArgs Empty = new ConnectorArgs();
/**
* Information about the capacity allocated to the connector. See `capacity` Block for details.
*
*/
@Import(name="capacity", required=true)
private Output capacity;
/**
* @return Information about the capacity allocated to the connector. See `capacity` Block for details.
*
*/
public Output capacity() {
return this.capacity;
}
/**
* A map of keys to values that represent the configuration for the connector.
*
*/
@Import(name="connectorConfiguration", required=true)
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy