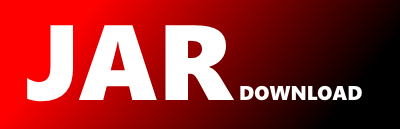
com.pulumi.aws.rds.CustomDbEngineVersionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.rds;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CustomDbEngineVersionArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomDbEngineVersionArgs Empty = new CustomDbEngineVersionArgs();
/**
* The name of the Amazon S3 bucket that contains the database installation files.
*
*/
@Import(name="databaseInstallationFilesS3BucketName")
private @Nullable Output databaseInstallationFilesS3BucketName;
/**
* @return The name of the Amazon S3 bucket that contains the database installation files.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy