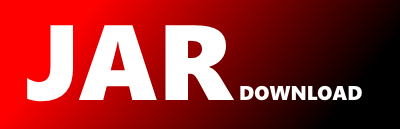
com.pulumi.aws.scheduler.Schedule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.scheduler;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.scheduler.ScheduleArgs;
import com.pulumi.aws.scheduler.inputs.ScheduleState;
import com.pulumi.aws.scheduler.outputs.ScheduleFlexibleTimeWindow;
import com.pulumi.aws.scheduler.outputs.ScheduleTarget;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an EventBridge Scheduler Schedule resource.
*
* You can find out more about EventBridge Scheduler in the [User Guide](https://docs.aws.amazon.com/scheduler/latest/UserGuide/what-is-scheduler.html).
*
* > **Note:** EventBridge was formerly known as CloudWatch Events. The functionality is identical.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.scheduler.Schedule;
* import com.pulumi.aws.scheduler.ScheduleArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleFlexibleTimeWindowArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Schedule("example", ScheduleArgs.builder()
* .name("my-schedule")
* .groupName("default")
* .flexibleTimeWindow(ScheduleFlexibleTimeWindowArgs.builder()
* .mode("OFF")
* .build())
* .scheduleExpression("rate(1 hours)")
* .target(ScheduleTargetArgs.builder()
* .arn(exampleAwsSqsQueue.arn())
* .roleArn(exampleAwsIamRole.arn())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Universal Target
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sqs.Queue;
* import com.pulumi.aws.scheduler.Schedule;
* import com.pulumi.aws.scheduler.ScheduleArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleFlexibleTimeWindowArgs;
* import com.pulumi.aws.scheduler.inputs.ScheduleTargetArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new Queue("example");
*
* var exampleSchedule = new Schedule("exampleSchedule", ScheduleArgs.builder()
* .name("my-schedule")
* .flexibleTimeWindow(ScheduleFlexibleTimeWindowArgs.builder()
* .mode("OFF")
* .build())
* .scheduleExpression("rate(1 hours)")
* .target(ScheduleTargetArgs.builder()
* .arn("arn:aws:scheduler:::aws-sdk:sqs:sendMessage")
* .roleArn(exampleAwsIamRole.arn())
* .input(example.url().applyValue(url -> serializeJson(
* jsonObject(
* jsonProperty("MessageBody", "Greetings, programs!"),
* jsonProperty("QueueUrl", url)
* ))))
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import schedules using the combination `group_name/name`. For example:
*
* ```sh
* $ pulumi import aws:scheduler/schedule:Schedule example my-schedule-group/my-schedule
* ```
*
*/
@ResourceType(type="aws:scheduler/schedule:Schedule")
public class Schedule extends com.pulumi.resources.CustomResource {
/**
* ARN of the schedule.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the schedule.
*
*/
public Output arn() {
return this.arn;
}
/**
* Brief description of the schedule.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Brief description of the schedule.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The date, in UTC, before which the schedule can invoke its target. Depending on the schedule's recurrence expression, invocations might stop on, or before, the end date you specify. EventBridge Scheduler ignores the end date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*
*/
@Export(name="endDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> endDate;
/**
* @return The date, in UTC, before which the schedule can invoke its target. Depending on the schedule's recurrence expression, invocations might stop on, or before, the end date you specify. EventBridge Scheduler ignores the end date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*
*/
public Output> endDate() {
return Codegen.optional(this.endDate);
}
/**
* Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
*
*/
@Export(name="flexibleTimeWindow", refs={ScheduleFlexibleTimeWindow.class}, tree="[0]")
private Output flexibleTimeWindow;
/**
* @return Configures a time window during which EventBridge Scheduler invokes the schedule. Detailed below.
*
*/
public Output flexibleTimeWindow() {
return this.flexibleTimeWindow;
}
/**
* Name of the schedule group to associate with this schedule. When omitted, the `default` schedule group is used.
*
*/
@Export(name="groupName", refs={String.class}, tree="[0]")
private Output groupName;
/**
* @return Name of the schedule group to associate with this schedule. When omitted, the `default` schedule group is used.
*
*/
public Output groupName() {
return this.groupName;
}
/**
* ARN for the customer managed KMS key that EventBridge Scheduler will use to encrypt and decrypt your data.
*
*/
@Export(name="kmsKeyArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsKeyArn;
/**
* @return ARN for the customer managed KMS key that EventBridge Scheduler will use to encrypt and decrypt your data.
*
*/
public Output> kmsKeyArn() {
return Codegen.optional(this.kmsKeyArn);
}
/**
* Name of the schedule. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the schedule. If omitted, the provider will assign a random, unique name. Conflicts with `name_prefix`.
*
*/
public Output name() {
return this.name;
}
/**
* Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*
*/
@Export(name="namePrefix", refs={String.class}, tree="[0]")
private Output namePrefix;
/**
* @return Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*
*/
public Output namePrefix() {
return this.namePrefix;
}
/**
* Defines when the schedule runs. Read more in [Schedule types on EventBridge Scheduler](https://docs.aws.amazon.com/scheduler/latest/UserGuide/schedule-types.html).
*
*/
@Export(name="scheduleExpression", refs={String.class}, tree="[0]")
private Output scheduleExpression;
/**
* @return Defines when the schedule runs. Read more in [Schedule types on EventBridge Scheduler](https://docs.aws.amazon.com/scheduler/latest/UserGuide/schedule-types.html).
*
*/
public Output scheduleExpression() {
return this.scheduleExpression;
}
/**
* Timezone in which the scheduling expression is evaluated. Defaults to `UTC`. Example: `Australia/Sydney`.
*
*/
@Export(name="scheduleExpressionTimezone", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> scheduleExpressionTimezone;
/**
* @return Timezone in which the scheduling expression is evaluated. Defaults to `UTC`. Example: `Australia/Sydney`.
*
*/
public Output> scheduleExpressionTimezone() {
return Codegen.optional(this.scheduleExpressionTimezone);
}
/**
* The date, in UTC, after which the schedule can begin invoking its target. Depending on the schedule's recurrence expression, invocations might occur on, or after, the start date you specify. EventBridge Scheduler ignores the start date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*
*/
@Export(name="startDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> startDate;
/**
* @return The date, in UTC, after which the schedule can begin invoking its target. Depending on the schedule's recurrence expression, invocations might occur on, or after, the start date you specify. EventBridge Scheduler ignores the start date for one-time schedules. Example: `2030-01-01T01:00:00Z`.
*
*/
public Output> startDate() {
return Codegen.optional(this.startDate);
}
/**
* Specifies whether the schedule is enabled or disabled. One of: `ENABLED` (default), `DISABLED`.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> state;
/**
* @return Specifies whether the schedule is enabled or disabled. One of: `ENABLED` (default), `DISABLED`.
*
*/
public Output> state() {
return Codegen.optional(this.state);
}
/**
* Configures the target of the schedule. Detailed below.
*
* The following arguments are optional:
*
*/
@Export(name="target", refs={ScheduleTarget.class}, tree="[0]")
private Output target;
/**
* @return Configures the target of the schedule. Detailed below.
*
* The following arguments are optional:
*
*/
public Output target() {
return this.target;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Schedule(java.lang.String name) {
this(name, ScheduleArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Schedule(java.lang.String name, ScheduleArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Schedule(java.lang.String name, ScheduleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:scheduler/schedule:Schedule", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Schedule(java.lang.String name, Output id, @Nullable ScheduleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:scheduler/schedule:Schedule", name, state, makeResourceOptions(options, id), false);
}
private static ScheduleArgs makeArgs(ScheduleArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ScheduleArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Schedule get(java.lang.String name, Output id, @Nullable ScheduleState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Schedule(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy