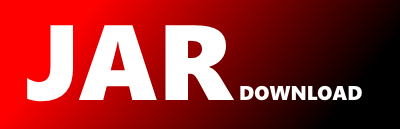
com.pulumi.aws.scheduler.outputs.ScheduleTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.scheduler.outputs;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetDeadLetterConfig;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParameters;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEventbridgeParameters;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetKinesisParameters;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetRetryPolicy;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetSagemakerPipelineParameters;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetSqsParameters;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ScheduleTarget {
/**
* @return ARN of the target of this schedule, such as a SQS queue or ECS cluster. For universal targets, this is a [Service ARN specific to the target service](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html#supported-universal-targets).
*
*/
private String arn;
/**
* @return Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
*
*/
private @Nullable ScheduleTargetDeadLetterConfig deadLetterConfig;
/**
* @return Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
*
*/
private @Nullable ScheduleTargetEcsParameters ecsParameters;
/**
* @return Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
*
*/
private @Nullable ScheduleTargetEventbridgeParameters eventbridgeParameters;
/**
* @return Text, or well-formed JSON, passed to the target. Read more in [Universal target](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html).
*
*/
private @Nullable String input;
/**
* @return Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
*
*/
private @Nullable ScheduleTargetKinesisParameters kinesisParameters;
/**
* @return Information about the retry policy settings. Detailed below.
*
*/
private @Nullable ScheduleTargetRetryPolicy retryPolicy;
/**
* @return ARN of the IAM role that EventBridge Scheduler will use for this target when the schedule is invoked. Read more in [Set up the execution role](https://docs.aws.amazon.com/scheduler/latest/UserGuide/setting-up.html#setting-up-execution-role).
*
* The following arguments are optional:
*
*/
private String roleArn;
/**
* @return Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
*
*/
private @Nullable ScheduleTargetSagemakerPipelineParameters sagemakerPipelineParameters;
/**
* @return The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*
*/
private @Nullable ScheduleTargetSqsParameters sqsParameters;
private ScheduleTarget() {}
/**
* @return ARN of the target of this schedule, such as a SQS queue or ECS cluster. For universal targets, this is a [Service ARN specific to the target service](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html#supported-universal-targets).
*
*/
public String arn() {
return this.arn;
}
/**
* @return Information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue. Detailed below.
*
*/
public Optional deadLetterConfig() {
return Optional.ofNullable(this.deadLetterConfig);
}
/**
* @return Templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation. Detailed below.
*
*/
public Optional ecsParameters() {
return Optional.ofNullable(this.ecsParameters);
}
/**
* @return Templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation. Detailed below.
*
*/
public Optional eventbridgeParameters() {
return Optional.ofNullable(this.eventbridgeParameters);
}
/**
* @return Text, or well-formed JSON, passed to the target. Read more in [Universal target](https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html).
*
*/
public Optional input() {
return Optional.ofNullable(this.input);
}
/**
* @return Templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation. Detailed below.
*
*/
public Optional kinesisParameters() {
return Optional.ofNullable(this.kinesisParameters);
}
/**
* @return Information about the retry policy settings. Detailed below.
*
*/
public Optional retryPolicy() {
return Optional.ofNullable(this.retryPolicy);
}
/**
* @return ARN of the IAM role that EventBridge Scheduler will use for this target when the schedule is invoked. Read more in [Set up the execution role](https://docs.aws.amazon.com/scheduler/latest/UserGuide/setting-up.html#setting-up-execution-role).
*
* The following arguments are optional:
*
*/
public String roleArn() {
return this.roleArn;
}
/**
* @return Templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation. Detailed below.
*
*/
public Optional sagemakerPipelineParameters() {
return Optional.ofNullable(this.sagemakerPipelineParameters);
}
/**
* @return The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Detailed below.
*
*/
public Optional sqsParameters() {
return Optional.ofNullable(this.sqsParameters);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ScheduleTarget defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private @Nullable ScheduleTargetDeadLetterConfig deadLetterConfig;
private @Nullable ScheduleTargetEcsParameters ecsParameters;
private @Nullable ScheduleTargetEventbridgeParameters eventbridgeParameters;
private @Nullable String input;
private @Nullable ScheduleTargetKinesisParameters kinesisParameters;
private @Nullable ScheduleTargetRetryPolicy retryPolicy;
private String roleArn;
private @Nullable ScheduleTargetSagemakerPipelineParameters sagemakerPipelineParameters;
private @Nullable ScheduleTargetSqsParameters sqsParameters;
public Builder() {}
public Builder(ScheduleTarget defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.deadLetterConfig = defaults.deadLetterConfig;
this.ecsParameters = defaults.ecsParameters;
this.eventbridgeParameters = defaults.eventbridgeParameters;
this.input = defaults.input;
this.kinesisParameters = defaults.kinesisParameters;
this.retryPolicy = defaults.retryPolicy;
this.roleArn = defaults.roleArn;
this.sagemakerPipelineParameters = defaults.sagemakerPipelineParameters;
this.sqsParameters = defaults.sqsParameters;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("ScheduleTarget", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder deadLetterConfig(@Nullable ScheduleTargetDeadLetterConfig deadLetterConfig) {
this.deadLetterConfig = deadLetterConfig;
return this;
}
@CustomType.Setter
public Builder ecsParameters(@Nullable ScheduleTargetEcsParameters ecsParameters) {
this.ecsParameters = ecsParameters;
return this;
}
@CustomType.Setter
public Builder eventbridgeParameters(@Nullable ScheduleTargetEventbridgeParameters eventbridgeParameters) {
this.eventbridgeParameters = eventbridgeParameters;
return this;
}
@CustomType.Setter
public Builder input(@Nullable String input) {
this.input = input;
return this;
}
@CustomType.Setter
public Builder kinesisParameters(@Nullable ScheduleTargetKinesisParameters kinesisParameters) {
this.kinesisParameters = kinesisParameters;
return this;
}
@CustomType.Setter
public Builder retryPolicy(@Nullable ScheduleTargetRetryPolicy retryPolicy) {
this.retryPolicy = retryPolicy;
return this;
}
@CustomType.Setter
public Builder roleArn(String roleArn) {
if (roleArn == null) {
throw new MissingRequiredPropertyException("ScheduleTarget", "roleArn");
}
this.roleArn = roleArn;
return this;
}
@CustomType.Setter
public Builder sagemakerPipelineParameters(@Nullable ScheduleTargetSagemakerPipelineParameters sagemakerPipelineParameters) {
this.sagemakerPipelineParameters = sagemakerPipelineParameters;
return this;
}
@CustomType.Setter
public Builder sqsParameters(@Nullable ScheduleTargetSqsParameters sqsParameters) {
this.sqsParameters = sqsParameters;
return this;
}
public ScheduleTarget build() {
final var _resultValue = new ScheduleTarget();
_resultValue.arn = arn;
_resultValue.deadLetterConfig = deadLetterConfig;
_resultValue.ecsParameters = ecsParameters;
_resultValue.eventbridgeParameters = eventbridgeParameters;
_resultValue.input = input;
_resultValue.kinesisParameters = kinesisParameters;
_resultValue.retryPolicy = retryPolicy;
_resultValue.roleArn = roleArn;
_resultValue.sagemakerPipelineParameters = sagemakerPipelineParameters;
_resultValue.sqsParameters = sqsParameters;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy