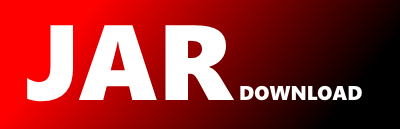
com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParameters Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.scheduler.outputs;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParametersCapacityProviderStrategy;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParametersNetworkConfiguration;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParametersPlacementConstraint;
import com.pulumi.aws.scheduler.outputs.ScheduleTargetEcsParametersPlacementStrategy;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ScheduleTargetEcsParameters {
/**
* @return Up to `6` capacity provider strategies to use for the task. Detailed below.
*
*/
private @Nullable List capacityProviderStrategies;
/**
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see [Tagging Your Amazon ECS Resources](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/ecs-using-tags.html) in the Amazon ECS Developer Guide.
*
*/
private @Nullable Boolean enableEcsManagedTags;
/**
* @return Specifies whether to enable the execute command functionality for the containers in this task.
*
*/
private @Nullable Boolean enableExecuteCommand;
/**
* @return Specifies an ECS task group for the task. At most 255 characters.
*
*/
private @Nullable String group;
/**
* @return Specifies the launch type on which your task is running. The launch type that you specify here must match one of the launch type (compatibilities) of the target task. One of: `EC2`, `FARGATE`, `EXTERNAL`.
*
*/
private @Nullable String launchType;
/**
* @return Configures the networking associated with the task. Detailed below.
*
*/
private @Nullable ScheduleTargetEcsParametersNetworkConfiguration networkConfiguration;
/**
* @return A set of up to 10 placement constraints to use for the task. Detailed below.
*
*/
private @Nullable List placementConstraints;
/**
* @return A set of up to 5 placement strategies. Detailed below.
*
*/
private @Nullable List placementStrategies;
/**
* @return Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as `1.1.0`.
*
*/
private @Nullable String platformVersion;
/**
* @return Specifies whether to propagate the tags from the task definition to the task. One of: `TASK_DEFINITION`.
*
*/
private @Nullable String propagateTags;
/**
* @return Reference ID to use for the task.
*
*/
private @Nullable String referenceId;
/**
* @return The metadata that you apply to the task. Each tag consists of a key and an optional value. For more information, see [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) in the Amazon ECS API Reference.
*
*/
private @Nullable Map tags;
/**
* @return The number of tasks to create. Ranges from `1` (default) to `10`.
*
*/
private @Nullable Integer taskCount;
/**
* @return ARN of the task definition to use.
*
* The following arguments are optional:
*
*/
private String taskDefinitionArn;
private ScheduleTargetEcsParameters() {}
/**
* @return Up to `6` capacity provider strategies to use for the task. Detailed below.
*
*/
public List capacityProviderStrategies() {
return this.capacityProviderStrategies == null ? List.of() : this.capacityProviderStrategies;
}
/**
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see [Tagging Your Amazon ECS Resources](https://docs.aws.amazon.com/AmazonECS/latest/developerguide/ecs-using-tags.html) in the Amazon ECS Developer Guide.
*
*/
public Optional enableEcsManagedTags() {
return Optional.ofNullable(this.enableEcsManagedTags);
}
/**
* @return Specifies whether to enable the execute command functionality for the containers in this task.
*
*/
public Optional enableExecuteCommand() {
return Optional.ofNullable(this.enableExecuteCommand);
}
/**
* @return Specifies an ECS task group for the task. At most 255 characters.
*
*/
public Optional group() {
return Optional.ofNullable(this.group);
}
/**
* @return Specifies the launch type on which your task is running. The launch type that you specify here must match one of the launch type (compatibilities) of the target task. One of: `EC2`, `FARGATE`, `EXTERNAL`.
*
*/
public Optional launchType() {
return Optional.ofNullable(this.launchType);
}
/**
* @return Configures the networking associated with the task. Detailed below.
*
*/
public Optional networkConfiguration() {
return Optional.ofNullable(this.networkConfiguration);
}
/**
* @return A set of up to 10 placement constraints to use for the task. Detailed below.
*
*/
public List placementConstraints() {
return this.placementConstraints == null ? List.of() : this.placementConstraints;
}
/**
* @return A set of up to 5 placement strategies. Detailed below.
*
*/
public List placementStrategies() {
return this.placementStrategies == null ? List.of() : this.placementStrategies;
}
/**
* @return Specifies the platform version for the task. Specify only the numeric portion of the platform version, such as `1.1.0`.
*
*/
public Optional platformVersion() {
return Optional.ofNullable(this.platformVersion);
}
/**
* @return Specifies whether to propagate the tags from the task definition to the task. One of: `TASK_DEFINITION`.
*
*/
public Optional propagateTags() {
return Optional.ofNullable(this.propagateTags);
}
/**
* @return Reference ID to use for the task.
*
*/
public Optional referenceId() {
return Optional.ofNullable(this.referenceId);
}
/**
* @return The metadata that you apply to the task. Each tag consists of a key and an optional value. For more information, see [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) in the Amazon ECS API Reference.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The number of tasks to create. Ranges from `1` (default) to `10`.
*
*/
public Optional taskCount() {
return Optional.ofNullable(this.taskCount);
}
/**
* @return ARN of the task definition to use.
*
* The following arguments are optional:
*
*/
public String taskDefinitionArn() {
return this.taskDefinitionArn;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ScheduleTargetEcsParameters defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List capacityProviderStrategies;
private @Nullable Boolean enableEcsManagedTags;
private @Nullable Boolean enableExecuteCommand;
private @Nullable String group;
private @Nullable String launchType;
private @Nullable ScheduleTargetEcsParametersNetworkConfiguration networkConfiguration;
private @Nullable List placementConstraints;
private @Nullable List placementStrategies;
private @Nullable String platformVersion;
private @Nullable String propagateTags;
private @Nullable String referenceId;
private @Nullable Map tags;
private @Nullable Integer taskCount;
private String taskDefinitionArn;
public Builder() {}
public Builder(ScheduleTargetEcsParameters defaults) {
Objects.requireNonNull(defaults);
this.capacityProviderStrategies = defaults.capacityProviderStrategies;
this.enableEcsManagedTags = defaults.enableEcsManagedTags;
this.enableExecuteCommand = defaults.enableExecuteCommand;
this.group = defaults.group;
this.launchType = defaults.launchType;
this.networkConfiguration = defaults.networkConfiguration;
this.placementConstraints = defaults.placementConstraints;
this.placementStrategies = defaults.placementStrategies;
this.platformVersion = defaults.platformVersion;
this.propagateTags = defaults.propagateTags;
this.referenceId = defaults.referenceId;
this.tags = defaults.tags;
this.taskCount = defaults.taskCount;
this.taskDefinitionArn = defaults.taskDefinitionArn;
}
@CustomType.Setter
public Builder capacityProviderStrategies(@Nullable List capacityProviderStrategies) {
this.capacityProviderStrategies = capacityProviderStrategies;
return this;
}
public Builder capacityProviderStrategies(ScheduleTargetEcsParametersCapacityProviderStrategy... capacityProviderStrategies) {
return capacityProviderStrategies(List.of(capacityProviderStrategies));
}
@CustomType.Setter
public Builder enableEcsManagedTags(@Nullable Boolean enableEcsManagedTags) {
this.enableEcsManagedTags = enableEcsManagedTags;
return this;
}
@CustomType.Setter
public Builder enableExecuteCommand(@Nullable Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
return this;
}
@CustomType.Setter
public Builder group(@Nullable String group) {
this.group = group;
return this;
}
@CustomType.Setter
public Builder launchType(@Nullable String launchType) {
this.launchType = launchType;
return this;
}
@CustomType.Setter
public Builder networkConfiguration(@Nullable ScheduleTargetEcsParametersNetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
return this;
}
@CustomType.Setter
public Builder placementConstraints(@Nullable List placementConstraints) {
this.placementConstraints = placementConstraints;
return this;
}
public Builder placementConstraints(ScheduleTargetEcsParametersPlacementConstraint... placementConstraints) {
return placementConstraints(List.of(placementConstraints));
}
@CustomType.Setter
public Builder placementStrategies(@Nullable List placementStrategies) {
this.placementStrategies = placementStrategies;
return this;
}
public Builder placementStrategies(ScheduleTargetEcsParametersPlacementStrategy... placementStrategies) {
return placementStrategies(List.of(placementStrategies));
}
@CustomType.Setter
public Builder platformVersion(@Nullable String platformVersion) {
this.platformVersion = platformVersion;
return this;
}
@CustomType.Setter
public Builder propagateTags(@Nullable String propagateTags) {
this.propagateTags = propagateTags;
return this;
}
@CustomType.Setter
public Builder referenceId(@Nullable String referenceId) {
this.referenceId = referenceId;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder taskCount(@Nullable Integer taskCount) {
this.taskCount = taskCount;
return this;
}
@CustomType.Setter
public Builder taskDefinitionArn(String taskDefinitionArn) {
if (taskDefinitionArn == null) {
throw new MissingRequiredPropertyException("ScheduleTargetEcsParameters", "taskDefinitionArn");
}
this.taskDefinitionArn = taskDefinitionArn;
return this;
}
public ScheduleTargetEcsParameters build() {
final var _resultValue = new ScheduleTargetEcsParameters();
_resultValue.capacityProviderStrategies = capacityProviderStrategies;
_resultValue.enableEcsManagedTags = enableEcsManagedTags;
_resultValue.enableExecuteCommand = enableExecuteCommand;
_resultValue.group = group;
_resultValue.launchType = launchType;
_resultValue.networkConfiguration = networkConfiguration;
_resultValue.placementConstraints = placementConstraints;
_resultValue.placementStrategies = placementStrategies;
_resultValue.platformVersion = platformVersion;
_resultValue.propagateTags = propagateTags;
_resultValue.referenceId = referenceId;
_resultValue.tags = tags;
_resultValue.taskCount = taskCount;
_resultValue.taskDefinitionArn = taskDefinitionArn;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy