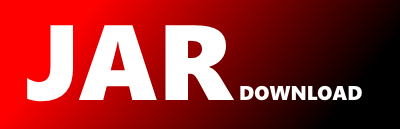
com.pulumi.aws.servicecatalog.ProvisionedProduct Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.servicecatalog;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.servicecatalog.ProvisionedProductArgs;
import com.pulumi.aws.servicecatalog.inputs.ProvisionedProductState;
import com.pulumi.aws.servicecatalog.outputs.ProvisionedProductOutput;
import com.pulumi.aws.servicecatalog.outputs.ProvisionedProductProvisioningParameter;
import com.pulumi.aws.servicecatalog.outputs.ProvisionedProductStackSetProvisioningPreferences;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* This resource provisions and manages a Service Catalog provisioned product.
*
* A provisioned product is a resourced instance of a product. For example, provisioning a product based on a CloudFormation template launches a CloudFormation stack and its underlying resources.
*
* Like this resource, the `aws_servicecatalog_record` data source also provides information about a provisioned product. Although a Service Catalog record provides some overlapping information with this resource, a record is tied to a provisioned product event, such as provisioning, termination, and updating.
*
* > **Tip:** If you include conflicted keys as tags, AWS will report an error, "Parameter validation failed: Missing required parameter in Tags[N]:Value".
*
* > **Tip:** A "provisioning artifact" is also referred to as a "version." A "distributor" is also referred to as a "vendor."
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.servicecatalog.ProvisionedProduct;
* import com.pulumi.aws.servicecatalog.ProvisionedProductArgs;
* import com.pulumi.aws.servicecatalog.inputs.ProvisionedProductProvisioningParameterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ProvisionedProduct("example", ProvisionedProductArgs.builder()
* .name("example")
* .productName("Example product")
* .provisioningArtifactName("Example version")
* .provisioningParameters(ProvisionedProductProvisioningParameterArgs.builder()
* .key("foo")
* .value("bar")
* .build())
* .tags(Map.of("foo", "bar"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_servicecatalog_provisioned_product` using the provisioned product ID. For example:
*
* ```sh
* $ pulumi import aws:servicecatalog/provisionedProduct:ProvisionedProduct example pp-dnigbtea24ste
* ```
*
*/
@ResourceType(type="aws:servicecatalog/provisionedProduct:ProvisionedProduct")
public class ProvisionedProduct extends com.pulumi.resources.CustomResource {
/**
* Language code. Valid values: `en` (English), `jp` (Japanese), `zh` (Chinese). Default value is `en`.
*
*/
@Export(name="acceptLanguage", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> acceptLanguage;
/**
* @return Language code. Valid values: `en` (English), `jp` (Japanese), `zh` (Chinese). Default value is `en`.
*
*/
public Output> acceptLanguage() {
return Codegen.optional(this.acceptLanguage);
}
/**
* ARN of the provisioned product.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the provisioned product.
*
*/
public Output arn() {
return this.arn;
}
/**
* Set of CloudWatch dashboards that were created when provisioning the product.
*
*/
@Export(name="cloudwatchDashboardNames", refs={List.class,String.class}, tree="[0,1]")
private Output> cloudwatchDashboardNames;
/**
* @return Set of CloudWatch dashboards that were created when provisioning the product.
*
*/
public Output> cloudwatchDashboardNames() {
return this.cloudwatchDashboardNames;
}
/**
* Time when the provisioned product was created.
*
*/
@Export(name="createdTime", refs={String.class}, tree="[0]")
private Output createdTime;
/**
* @return Time when the provisioned product was created.
*
*/
public Output createdTime() {
return this.createdTime;
}
/**
* _Only applies to deleting._ If set to `true`, AWS Service Catalog stops managing the specified provisioned product even if it cannot delete the underlying resources. The default value is `false`.
*
*/
@Export(name="ignoreErrors", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> ignoreErrors;
/**
* @return _Only applies to deleting._ If set to `true`, AWS Service Catalog stops managing the specified provisioned product even if it cannot delete the underlying resources. The default value is `false`.
*
*/
public Output> ignoreErrors() {
return Codegen.optional(this.ignoreErrors);
}
/**
* Record identifier of the last request performed on this provisioned product of the following types: `ProvisionedProduct`, `UpdateProvisionedProduct`, `ExecuteProvisionedProductPlan`, `TerminateProvisionedProduct`.
*
*/
@Export(name="lastProvisioningRecordId", refs={String.class}, tree="[0]")
private Output lastProvisioningRecordId;
/**
* @return Record identifier of the last request performed on this provisioned product of the following types: `ProvisionedProduct`, `UpdateProvisionedProduct`, `ExecuteProvisionedProductPlan`, `TerminateProvisionedProduct`.
*
*/
public Output lastProvisioningRecordId() {
return this.lastProvisioningRecordId;
}
/**
* Record identifier of the last request performed on this provisioned product.
*
*/
@Export(name="lastRecordId", refs={String.class}, tree="[0]")
private Output lastRecordId;
/**
* @return Record identifier of the last request performed on this provisioned product.
*
*/
public Output lastRecordId() {
return this.lastRecordId;
}
/**
* Record identifier of the last successful request performed on this provisioned product of the following types: `ProvisionedProduct`, `UpdateProvisionedProduct`, `ExecuteProvisionedProductPlan`, `TerminateProvisionedProduct`.
*
*/
@Export(name="lastSuccessfulProvisioningRecordId", refs={String.class}, tree="[0]")
private Output lastSuccessfulProvisioningRecordId;
/**
* @return Record identifier of the last successful request performed on this provisioned product of the following types: `ProvisionedProduct`, `UpdateProvisionedProduct`, `ExecuteProvisionedProductPlan`, `TerminateProvisionedProduct`.
*
*/
public Output lastSuccessfulProvisioningRecordId() {
return this.lastSuccessfulProvisioningRecordId;
}
/**
* ARN of the launch role associated with the provisioned product.
*
*/
@Export(name="launchRoleArn", refs={String.class}, tree="[0]")
private Output launchRoleArn;
/**
* @return ARN of the launch role associated with the provisioned product.
*
*/
public Output launchRoleArn() {
return this.launchRoleArn;
}
/**
* User-friendly name of the provisioned product.
*
* The following arguments are optional:
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return User-friendly name of the provisioned product.
*
* The following arguments are optional:
*
*/
public Output name() {
return this.name;
}
/**
* Passed to CloudFormation. The SNS topic ARNs to which to publish stack-related events.
*
*/
@Export(name="notificationArns", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> notificationArns;
/**
* @return Passed to CloudFormation. The SNS topic ARNs to which to publish stack-related events.
*
*/
public Output>> notificationArns() {
return Codegen.optional(this.notificationArns);
}
/**
* The set of outputs for the product created.
*
*/
@Export(name="outputs", refs={List.class,ProvisionedProductOutput.class}, tree="[0,1]")
private Output> outputs;
/**
* @return The set of outputs for the product created.
*
*/
public Output> outputs() {
return this.outputs;
}
/**
* Path identifier of the product. This value is optional if the product has a default path, and required if the product has more than one path. To list the paths for a product, use `aws.servicecatalog.getLaunchPaths`. When required, you must provide `path_id` or `path_name`, but not both.
*
*/
@Export(name="pathId", refs={String.class}, tree="[0]")
private Output pathId;
/**
* @return Path identifier of the product. This value is optional if the product has a default path, and required if the product has more than one path. To list the paths for a product, use `aws.servicecatalog.getLaunchPaths`. When required, you must provide `path_id` or `path_name`, but not both.
*
*/
public Output pathId() {
return this.pathId;
}
/**
* Name of the path. You must provide `path_id` or `path_name`, but not both.
*
*/
@Export(name="pathName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> pathName;
/**
* @return Name of the path. You must provide `path_id` or `path_name`, but not both.
*
*/
public Output> pathName() {
return Codegen.optional(this.pathName);
}
/**
* Product identifier. For example, `prod-abcdzk7xy33qa`. You must provide `product_id` or `product_name`, but not both.
*
*/
@Export(name="productId", refs={String.class}, tree="[0]")
private Output productId;
/**
* @return Product identifier. For example, `prod-abcdzk7xy33qa`. You must provide `product_id` or `product_name`, but not both.
*
*/
public Output productId() {
return this.productId;
}
/**
* Name of the product. You must provide `product_id` or `product_name`, but not both.
*
*/
@Export(name="productName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> productName;
/**
* @return Name of the product. You must provide `product_id` or `product_name`, but not both.
*
*/
public Output> productName() {
return Codegen.optional(this.productName);
}
/**
* Identifier of the provisioning artifact. For example, `pa-4abcdjnxjj6ne`. You must provide the `provisioning_artifact_id` or `provisioning_artifact_name`, but not both.
*
*/
@Export(name="provisioningArtifactId", refs={String.class}, tree="[0]")
private Output provisioningArtifactId;
/**
* @return Identifier of the provisioning artifact. For example, `pa-4abcdjnxjj6ne`. You must provide the `provisioning_artifact_id` or `provisioning_artifact_name`, but not both.
*
*/
public Output provisioningArtifactId() {
return this.provisioningArtifactId;
}
/**
* Name of the provisioning artifact. You must provide the `provisioning_artifact_id` or `provisioning_artifact_name`, but not both.
*
*/
@Export(name="provisioningArtifactName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> provisioningArtifactName;
/**
* @return Name of the provisioning artifact. You must provide the `provisioning_artifact_id` or `provisioning_artifact_name`, but not both.
*
*/
public Output> provisioningArtifactName() {
return Codegen.optional(this.provisioningArtifactName);
}
/**
* Configuration block with parameters specified by the administrator that are required for provisioning the product. See `provisioning_parameters` Block for details.
*
*/
@Export(name="provisioningParameters", refs={List.class,ProvisionedProductProvisioningParameter.class}, tree="[0,1]")
private Output* @Nullable */ List> provisioningParameters;
/**
* @return Configuration block with parameters specified by the administrator that are required for provisioning the product. See `provisioning_parameters` Block for details.
*
*/
public Output>> provisioningParameters() {
return Codegen.optional(this.provisioningParameters);
}
/**
* _Only applies to deleting._ Whether to delete the Service Catalog provisioned product but leave the CloudFormation stack, stack set, or the underlying resources of the deleted provisioned product. The default value is `false`.
*
*/
@Export(name="retainPhysicalResources", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> retainPhysicalResources;
/**
* @return _Only applies to deleting._ Whether to delete the Service Catalog provisioned product but leave the CloudFormation stack, stack set, or the underlying resources of the deleted provisioned product. The default value is `false`.
*
*/
public Output> retainPhysicalResources() {
return Codegen.optional(this.retainPhysicalResources);
}
/**
* Configuration block with information about the provisioning preferences for a stack set. See `stack_set_provisioning_preferences` Block for details.
*
*/
@Export(name="stackSetProvisioningPreferences", refs={ProvisionedProductStackSetProvisioningPreferences.class}, tree="[0]")
private Output* @Nullable */ ProvisionedProductStackSetProvisioningPreferences> stackSetProvisioningPreferences;
/**
* @return Configuration block with information about the provisioning preferences for a stack set. See `stack_set_provisioning_preferences` Block for details.
*
*/
public Output> stackSetProvisioningPreferences() {
return Codegen.optional(this.stackSetProvisioningPreferences);
}
/**
* Current status of the provisioned product. See meanings below.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Current status of the provisioned product. See meanings below.
*
*/
public Output status() {
return this.status;
}
/**
* Current status message of the provisioned product.
*
*/
@Export(name="statusMessage", refs={String.class}, tree="[0]")
private Output statusMessage;
/**
* @return Current status message of the provisioned product.
*
*/
public Output statusMessage() {
return this.statusMessage;
}
/**
* Tags to apply to the provisioned product. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Tags to apply to the provisioned product. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy