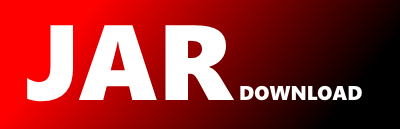
com.pulumi.aws.transfer.inputs.WorkflowStepArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.transfer.inputs;
import com.pulumi.aws.transfer.inputs.WorkflowStepCopyStepDetailsArgs;
import com.pulumi.aws.transfer.inputs.WorkflowStepCustomStepDetailsArgs;
import com.pulumi.aws.transfer.inputs.WorkflowStepDecryptStepDetailsArgs;
import com.pulumi.aws.transfer.inputs.WorkflowStepDeleteStepDetailsArgs;
import com.pulumi.aws.transfer.inputs.WorkflowStepTagStepDetailsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkflowStepArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkflowStepArgs Empty = new WorkflowStepArgs();
/**
* Details for a step that performs a file copy. See Copy Step Details below.
*
*/
@Import(name="copyStepDetails")
private @Nullable Output copyStepDetails;
/**
* @return Details for a step that performs a file copy. See Copy Step Details below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy