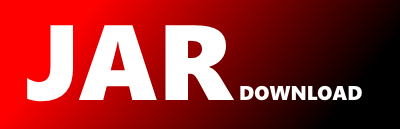
com.pulumi.aws.wafv2.outputs.WebAclRuleStatementRateBasedStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.wafv2.outputs;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementRateBasedStatementCustomKey;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementRateBasedStatementForwardedIpConfig;
import com.pulumi.aws.wafv2.outputs.WebAclRuleStatementRateBasedStatementScopeDownStatement;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class WebAclRuleStatementRateBasedStatement {
/**
* @return Setting that indicates how to aggregate the request counts. Valid values include: `CONSTANT`, `CUSTOM_KEYS`, `FORWARDED_IP`, or `IP`. Default: `IP`.
*
*/
private @Nullable String aggregateKeyType;
/**
* @return Aggregate the request counts using one or more web request components as the aggregate keys. See `custom_key` below for details.
*
*/
private @Nullable List customKeys;
/**
* @return The amount of time, in seconds, that AWS WAF should include in its request counts, looking back from the current time. Valid values are `60`, `120`, `300`, and `600`. Defaults to `300` (5 minutes).
*
* **NOTE:** This setting doesn't determine how often AWS WAF checks the rate, but how far back it looks each time it checks. AWS WAF checks the rate about every 10 seconds.
*
*/
private @Nullable Integer evaluationWindowSec;
/**
* @return Configuration for inspecting IP addresses in an HTTP header that you specify, instead of using the IP address that's reported by the web request origin. If `aggregate_key_type` is set to `FORWARDED_IP`, this block is required. See `forwarded_ip_config` below for details.
*
*/
private @Nullable WebAclRuleStatementRateBasedStatementForwardedIpConfig forwardedIpConfig;
/**
* @return Limit on requests per 5-minute period for a single originating IP address.
*
*/
private Integer limit;
/**
* @return Optional nested statement that narrows the scope of the rate-based statement to matching web requests. This can be any nestable statement, and you can nest statements at any level below this scope-down statement. See `statement` above for details. If `aggregate_key_type` is set to `CONSTANT`, this block is required.
*
*/
private @Nullable WebAclRuleStatementRateBasedStatementScopeDownStatement scopeDownStatement;
private WebAclRuleStatementRateBasedStatement() {}
/**
* @return Setting that indicates how to aggregate the request counts. Valid values include: `CONSTANT`, `CUSTOM_KEYS`, `FORWARDED_IP`, or `IP`. Default: `IP`.
*
*/
public Optional aggregateKeyType() {
return Optional.ofNullable(this.aggregateKeyType);
}
/**
* @return Aggregate the request counts using one or more web request components as the aggregate keys. See `custom_key` below for details.
*
*/
public List customKeys() {
return this.customKeys == null ? List.of() : this.customKeys;
}
/**
* @return The amount of time, in seconds, that AWS WAF should include in its request counts, looking back from the current time. Valid values are `60`, `120`, `300`, and `600`. Defaults to `300` (5 minutes).
*
* **NOTE:** This setting doesn't determine how often AWS WAF checks the rate, but how far back it looks each time it checks. AWS WAF checks the rate about every 10 seconds.
*
*/
public Optional evaluationWindowSec() {
return Optional.ofNullable(this.evaluationWindowSec);
}
/**
* @return Configuration for inspecting IP addresses in an HTTP header that you specify, instead of using the IP address that's reported by the web request origin. If `aggregate_key_type` is set to `FORWARDED_IP`, this block is required. See `forwarded_ip_config` below for details.
*
*/
public Optional forwardedIpConfig() {
return Optional.ofNullable(this.forwardedIpConfig);
}
/**
* @return Limit on requests per 5-minute period for a single originating IP address.
*
*/
public Integer limit() {
return this.limit;
}
/**
* @return Optional nested statement that narrows the scope of the rate-based statement to matching web requests. This can be any nestable statement, and you can nest statements at any level below this scope-down statement. See `statement` above for details. If `aggregate_key_type` is set to `CONSTANT`, this block is required.
*
*/
public Optional scopeDownStatement() {
return Optional.ofNullable(this.scopeDownStatement);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(WebAclRuleStatementRateBasedStatement defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String aggregateKeyType;
private @Nullable List customKeys;
private @Nullable Integer evaluationWindowSec;
private @Nullable WebAclRuleStatementRateBasedStatementForwardedIpConfig forwardedIpConfig;
private Integer limit;
private @Nullable WebAclRuleStatementRateBasedStatementScopeDownStatement scopeDownStatement;
public Builder() {}
public Builder(WebAclRuleStatementRateBasedStatement defaults) {
Objects.requireNonNull(defaults);
this.aggregateKeyType = defaults.aggregateKeyType;
this.customKeys = defaults.customKeys;
this.evaluationWindowSec = defaults.evaluationWindowSec;
this.forwardedIpConfig = defaults.forwardedIpConfig;
this.limit = defaults.limit;
this.scopeDownStatement = defaults.scopeDownStatement;
}
@CustomType.Setter
public Builder aggregateKeyType(@Nullable String aggregateKeyType) {
this.aggregateKeyType = aggregateKeyType;
return this;
}
@CustomType.Setter
public Builder customKeys(@Nullable List customKeys) {
this.customKeys = customKeys;
return this;
}
public Builder customKeys(WebAclRuleStatementRateBasedStatementCustomKey... customKeys) {
return customKeys(List.of(customKeys));
}
@CustomType.Setter
public Builder evaluationWindowSec(@Nullable Integer evaluationWindowSec) {
this.evaluationWindowSec = evaluationWindowSec;
return this;
}
@CustomType.Setter
public Builder forwardedIpConfig(@Nullable WebAclRuleStatementRateBasedStatementForwardedIpConfig forwardedIpConfig) {
this.forwardedIpConfig = forwardedIpConfig;
return this;
}
@CustomType.Setter
public Builder limit(Integer limit) {
if (limit == null) {
throw new MissingRequiredPropertyException("WebAclRuleStatementRateBasedStatement", "limit");
}
this.limit = limit;
return this;
}
@CustomType.Setter
public Builder scopeDownStatement(@Nullable WebAclRuleStatementRateBasedStatementScopeDownStatement scopeDownStatement) {
this.scopeDownStatement = scopeDownStatement;
return this;
}
public WebAclRuleStatementRateBasedStatement build() {
final var _resultValue = new WebAclRuleStatementRateBasedStatement();
_resultValue.aggregateKeyType = aggregateKeyType;
_resultValue.customKeys = customKeys;
_resultValue.evaluationWindowSec = evaluationWindowSec;
_resultValue.forwardedIpConfig = forwardedIpConfig;
_resultValue.limit = limit;
_resultValue.scopeDownStatement = scopeDownStatement;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy