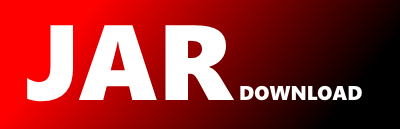
com.pulumi.azurenative.ProviderArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProviderArgs extends com.pulumi.resources.ResourceArgs {
public static final ProviderArgs Empty = new ProviderArgs();
/**
* Any additional Tenant IDs which should be used for authentication.
*
*/
@Import(name="auxiliaryTenantIds", json=true)
private @Nullable Output> auxiliaryTenantIds;
/**
* @return Any additional Tenant IDs which should be used for authentication.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy