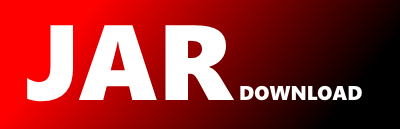
com.pulumi.azurenative.aad.outputs.GetDomainServiceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad.outputs;
import com.pulumi.azurenative.aad.outputs.ConfigDiagnosticsResponse;
import com.pulumi.azurenative.aad.outputs.DomainSecuritySettingsResponse;
import com.pulumi.azurenative.aad.outputs.LdapsSettingsResponse;
import com.pulumi.azurenative.aad.outputs.MigrationPropertiesResponse;
import com.pulumi.azurenative.aad.outputs.NotificationSettingsResponse;
import com.pulumi.azurenative.aad.outputs.ReplicaSetResponse;
import com.pulumi.azurenative.aad.outputs.ResourceForestSettingsResponse;
import com.pulumi.azurenative.aad.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDomainServiceResult {
/**
* @return Configuration diagnostics data containing latest execution from client.
*
*/
private @Nullable ConfigDiagnosticsResponse configDiagnostics;
/**
* @return Deployment Id
*
*/
private String deploymentId;
/**
* @return Domain Configuration Type
*
*/
private @Nullable String domainConfigurationType;
/**
* @return The name of the Azure domain that the user would like to deploy Domain Services to.
*
*/
private @Nullable String domainName;
/**
* @return DomainSecurity Settings
*
*/
private @Nullable DomainSecuritySettingsResponse domainSecuritySettings;
/**
* @return Resource etag
*
*/
private @Nullable String etag;
/**
* @return Enabled or Disabled flag to turn on Group-based filtered sync
*
*/
private @Nullable String filteredSync;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Secure LDAP Settings
*
*/
private @Nullable LdapsSettingsResponse ldapsSettings;
/**
* @return Resource location
*
*/
private @Nullable String location;
/**
* @return Migration Properties
*
*/
private MigrationPropertiesResponse migrationProperties;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Notification Settings
*
*/
private @Nullable NotificationSettingsResponse notificationSettings;
/**
* @return the current deployment or provisioning state, which only appears in the response.
*
*/
private String provisioningState;
/**
* @return List of ReplicaSets
*
*/
private @Nullable List replicaSets;
/**
* @return Resource Forest Settings
*
*/
private @Nullable ResourceForestSettingsResponse resourceForestSettings;
/**
* @return Sku Type
*
*/
private @Nullable String sku;
/**
* @return The unique sync application id of the Azure AD Domain Services deployment.
*
*/
private String syncApplicationId;
/**
* @return SyncOwner ReplicaSet Id
*
*/
private String syncOwner;
/**
* @return All or CloudOnly, All users in AAD are synced to AAD DS domain or only users actively syncing in the cloud
*
*/
private @Nullable String syncScope;
/**
* @return The system meta data relating to this resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Azure Active Directory Tenant Id
*
*/
private String tenantId;
/**
* @return Resource type
*
*/
private String type;
/**
* @return Data Model Version
*
*/
private Integer version;
private GetDomainServiceResult() {}
/**
* @return Configuration diagnostics data containing latest execution from client.
*
*/
public Optional configDiagnostics() {
return Optional.ofNullable(this.configDiagnostics);
}
/**
* @return Deployment Id
*
*/
public String deploymentId() {
return this.deploymentId;
}
/**
* @return Domain Configuration Type
*
*/
public Optional domainConfigurationType() {
return Optional.ofNullable(this.domainConfigurationType);
}
/**
* @return The name of the Azure domain that the user would like to deploy Domain Services to.
*
*/
public Optional domainName() {
return Optional.ofNullable(this.domainName);
}
/**
* @return DomainSecurity Settings
*
*/
public Optional domainSecuritySettings() {
return Optional.ofNullable(this.domainSecuritySettings);
}
/**
* @return Resource etag
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return Enabled or Disabled flag to turn on Group-based filtered sync
*
*/
public Optional filteredSync() {
return Optional.ofNullable(this.filteredSync);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Secure LDAP Settings
*
*/
public Optional ldapsSettings() {
return Optional.ofNullable(this.ldapsSettings);
}
/**
* @return Resource location
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Migration Properties
*
*/
public MigrationPropertiesResponse migrationProperties() {
return this.migrationProperties;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Notification Settings
*
*/
public Optional notificationSettings() {
return Optional.ofNullable(this.notificationSettings);
}
/**
* @return the current deployment or provisioning state, which only appears in the response.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return List of ReplicaSets
*
*/
public List replicaSets() {
return this.replicaSets == null ? List.of() : this.replicaSets;
}
/**
* @return Resource Forest Settings
*
*/
public Optional resourceForestSettings() {
return Optional.ofNullable(this.resourceForestSettings);
}
/**
* @return Sku Type
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The unique sync application id of the Azure AD Domain Services deployment.
*
*/
public String syncApplicationId() {
return this.syncApplicationId;
}
/**
* @return SyncOwner ReplicaSet Id
*
*/
public String syncOwner() {
return this.syncOwner;
}
/**
* @return All or CloudOnly, All users in AAD are synced to AAD DS domain or only users actively syncing in the cloud
*
*/
public Optional syncScope() {
return Optional.ofNullable(this.syncScope);
}
/**
* @return The system meta data relating to this resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Azure Active Directory Tenant Id
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
/**
* @return Data Model Version
*
*/
public Integer version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDomainServiceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ConfigDiagnosticsResponse configDiagnostics;
private String deploymentId;
private @Nullable String domainConfigurationType;
private @Nullable String domainName;
private @Nullable DomainSecuritySettingsResponse domainSecuritySettings;
private @Nullable String etag;
private @Nullable String filteredSync;
private String id;
private @Nullable LdapsSettingsResponse ldapsSettings;
private @Nullable String location;
private MigrationPropertiesResponse migrationProperties;
private String name;
private @Nullable NotificationSettingsResponse notificationSettings;
private String provisioningState;
private @Nullable List replicaSets;
private @Nullable ResourceForestSettingsResponse resourceForestSettings;
private @Nullable String sku;
private String syncApplicationId;
private String syncOwner;
private @Nullable String syncScope;
private SystemDataResponse systemData;
private @Nullable Map tags;
private String tenantId;
private String type;
private Integer version;
public Builder() {}
public Builder(GetDomainServiceResult defaults) {
Objects.requireNonNull(defaults);
this.configDiagnostics = defaults.configDiagnostics;
this.deploymentId = defaults.deploymentId;
this.domainConfigurationType = defaults.domainConfigurationType;
this.domainName = defaults.domainName;
this.domainSecuritySettings = defaults.domainSecuritySettings;
this.etag = defaults.etag;
this.filteredSync = defaults.filteredSync;
this.id = defaults.id;
this.ldapsSettings = defaults.ldapsSettings;
this.location = defaults.location;
this.migrationProperties = defaults.migrationProperties;
this.name = defaults.name;
this.notificationSettings = defaults.notificationSettings;
this.provisioningState = defaults.provisioningState;
this.replicaSets = defaults.replicaSets;
this.resourceForestSettings = defaults.resourceForestSettings;
this.sku = defaults.sku;
this.syncApplicationId = defaults.syncApplicationId;
this.syncOwner = defaults.syncOwner;
this.syncScope = defaults.syncScope;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
this.version = defaults.version;
}
@CustomType.Setter
public Builder configDiagnostics(@Nullable ConfigDiagnosticsResponse configDiagnostics) {
this.configDiagnostics = configDiagnostics;
return this;
}
@CustomType.Setter
public Builder deploymentId(String deploymentId) {
if (deploymentId == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "deploymentId");
}
this.deploymentId = deploymentId;
return this;
}
@CustomType.Setter
public Builder domainConfigurationType(@Nullable String domainConfigurationType) {
this.domainConfigurationType = domainConfigurationType;
return this;
}
@CustomType.Setter
public Builder domainName(@Nullable String domainName) {
this.domainName = domainName;
return this;
}
@CustomType.Setter
public Builder domainSecuritySettings(@Nullable DomainSecuritySettingsResponse domainSecuritySettings) {
this.domainSecuritySettings = domainSecuritySettings;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder filteredSync(@Nullable String filteredSync) {
this.filteredSync = filteredSync;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ldapsSettings(@Nullable LdapsSettingsResponse ldapsSettings) {
this.ldapsSettings = ldapsSettings;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder migrationProperties(MigrationPropertiesResponse migrationProperties) {
if (migrationProperties == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "migrationProperties");
}
this.migrationProperties = migrationProperties;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder notificationSettings(@Nullable NotificationSettingsResponse notificationSettings) {
this.notificationSettings = notificationSettings;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder replicaSets(@Nullable List replicaSets) {
this.replicaSets = replicaSets;
return this;
}
public Builder replicaSets(ReplicaSetResponse... replicaSets) {
return replicaSets(List.of(replicaSets));
}
@CustomType.Setter
public Builder resourceForestSettings(@Nullable ResourceForestSettingsResponse resourceForestSettings) {
this.resourceForestSettings = resourceForestSettings;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable String sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder syncApplicationId(String syncApplicationId) {
if (syncApplicationId == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "syncApplicationId");
}
this.syncApplicationId = syncApplicationId;
return this;
}
@CustomType.Setter
public Builder syncOwner(String syncOwner) {
if (syncOwner == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "syncOwner");
}
this.syncOwner = syncOwner;
return this;
}
@CustomType.Setter
public Builder syncScope(@Nullable String syncScope) {
this.syncScope = syncScope;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder version(Integer version) {
if (version == null) {
throw new MissingRequiredPropertyException("GetDomainServiceResult", "version");
}
this.version = version;
return this;
}
public GetDomainServiceResult build() {
final var _resultValue = new GetDomainServiceResult();
_resultValue.configDiagnostics = configDiagnostics;
_resultValue.deploymentId = deploymentId;
_resultValue.domainConfigurationType = domainConfigurationType;
_resultValue.domainName = domainName;
_resultValue.domainSecuritySettings = domainSecuritySettings;
_resultValue.etag = etag;
_resultValue.filteredSync = filteredSync;
_resultValue.id = id;
_resultValue.ldapsSettings = ldapsSettings;
_resultValue.location = location;
_resultValue.migrationProperties = migrationProperties;
_resultValue.name = name;
_resultValue.notificationSettings = notificationSettings;
_resultValue.provisioningState = provisioningState;
_resultValue.replicaSets = replicaSets;
_resultValue.resourceForestSettings = resourceForestSettings;
_resultValue.sku = sku;
_resultValue.syncApplicationId = syncApplicationId;
_resultValue.syncOwner = syncOwner;
_resultValue.syncScope = syncScope;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy