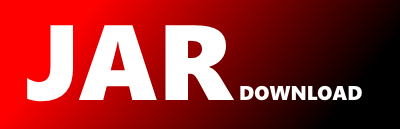
com.pulumi.azurenative.alertsmanagement.AlertsmanagementFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.alertsmanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.alertsmanagement.inputs.GetActionRuleByNameArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetActionRuleByNamePlainArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetAlertProcessingRuleByNameArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetAlertProcessingRuleByNamePlainArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetPrometheusRuleGroupArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetPrometheusRuleGroupPlainArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetSmartDetectorAlertRuleArgs;
import com.pulumi.azurenative.alertsmanagement.inputs.GetSmartDetectorAlertRulePlainArgs;
import com.pulumi.azurenative.alertsmanagement.outputs.GetActionRuleByNameResult;
import com.pulumi.azurenative.alertsmanagement.outputs.GetAlertProcessingRuleByNameResult;
import com.pulumi.azurenative.alertsmanagement.outputs.GetPrometheusRuleGroupResult;
import com.pulumi.azurenative.alertsmanagement.outputs.GetSmartDetectorAlertRuleResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class AlertsmanagementFunctions {
/**
* Get a specific action rule
* Azure REST API version: 2019-05-05-preview.
*
* Other available API versions: 2018-11-02-privatepreview.
*
*/
public static Output getActionRuleByName(GetActionRuleByNameArgs args) {
return getActionRuleByName(args, InvokeOptions.Empty);
}
/**
* Get a specific action rule
* Azure REST API version: 2019-05-05-preview.
*
* Other available API versions: 2018-11-02-privatepreview.
*
*/
public static CompletableFuture getActionRuleByNamePlain(GetActionRuleByNamePlainArgs args) {
return getActionRuleByNamePlain(args, InvokeOptions.Empty);
}
/**
* Get a specific action rule
* Azure REST API version: 2019-05-05-preview.
*
* Other available API versions: 2018-11-02-privatepreview.
*
*/
public static Output getActionRuleByName(GetActionRuleByNameArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:alertsmanagement:getActionRuleByName", TypeShape.of(GetActionRuleByNameResult.class), args, Utilities.withVersion(options));
}
/**
* Get a specific action rule
* Azure REST API version: 2019-05-05-preview.
*
* Other available API versions: 2018-11-02-privatepreview.
*
*/
public static CompletableFuture getActionRuleByNamePlain(GetActionRuleByNamePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:alertsmanagement:getActionRuleByName", TypeShape.of(GetActionRuleByNameResult.class), args, Utilities.withVersion(options));
}
/**
* Get an alert processing rule by name.
* Azure REST API version: 2021-08-08.
*
* Other available API versions: 2023-05-01-preview, 2024-03-01-preview.
*
*/
public static Output getAlertProcessingRuleByName(GetAlertProcessingRuleByNameArgs args) {
return getAlertProcessingRuleByName(args, InvokeOptions.Empty);
}
/**
* Get an alert processing rule by name.
* Azure REST API version: 2021-08-08.
*
* Other available API versions: 2023-05-01-preview, 2024-03-01-preview.
*
*/
public static CompletableFuture getAlertProcessingRuleByNamePlain(GetAlertProcessingRuleByNamePlainArgs args) {
return getAlertProcessingRuleByNamePlain(args, InvokeOptions.Empty);
}
/**
* Get an alert processing rule by name.
* Azure REST API version: 2021-08-08.
*
* Other available API versions: 2023-05-01-preview, 2024-03-01-preview.
*
*/
public static Output getAlertProcessingRuleByName(GetAlertProcessingRuleByNameArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:alertsmanagement:getAlertProcessingRuleByName", TypeShape.of(GetAlertProcessingRuleByNameResult.class), args, Utilities.withVersion(options));
}
/**
* Get an alert processing rule by name.
* Azure REST API version: 2021-08-08.
*
* Other available API versions: 2023-05-01-preview, 2024-03-01-preview.
*
*/
public static CompletableFuture getAlertProcessingRuleByNamePlain(GetAlertProcessingRuleByNamePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:alertsmanagement:getAlertProcessingRuleByName", TypeShape.of(GetAlertProcessingRuleByNameResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve a Prometheus rule group definition.
* Azure REST API version: 2023-03-01.
*
*/
public static Output getPrometheusRuleGroup(GetPrometheusRuleGroupArgs args) {
return getPrometheusRuleGroup(args, InvokeOptions.Empty);
}
/**
* Retrieve a Prometheus rule group definition.
* Azure REST API version: 2023-03-01.
*
*/
public static CompletableFuture getPrometheusRuleGroupPlain(GetPrometheusRuleGroupPlainArgs args) {
return getPrometheusRuleGroupPlain(args, InvokeOptions.Empty);
}
/**
* Retrieve a Prometheus rule group definition.
* Azure REST API version: 2023-03-01.
*
*/
public static Output getPrometheusRuleGroup(GetPrometheusRuleGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:alertsmanagement:getPrometheusRuleGroup", TypeShape.of(GetPrometheusRuleGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve a Prometheus rule group definition.
* Azure REST API version: 2023-03-01.
*
*/
public static CompletableFuture getPrometheusRuleGroupPlain(GetPrometheusRuleGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:alertsmanagement:getPrometheusRuleGroup", TypeShape.of(GetPrometheusRuleGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get a specific Smart Detector alert rule.
* Azure REST API version: 2021-04-01.
*
*/
public static Output getSmartDetectorAlertRule(GetSmartDetectorAlertRuleArgs args) {
return getSmartDetectorAlertRule(args, InvokeOptions.Empty);
}
/**
* Get a specific Smart Detector alert rule.
* Azure REST API version: 2021-04-01.
*
*/
public static CompletableFuture getSmartDetectorAlertRulePlain(GetSmartDetectorAlertRulePlainArgs args) {
return getSmartDetectorAlertRulePlain(args, InvokeOptions.Empty);
}
/**
* Get a specific Smart Detector alert rule.
* Azure REST API version: 2021-04-01.
*
*/
public static Output getSmartDetectorAlertRule(GetSmartDetectorAlertRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:alertsmanagement:getSmartDetectorAlertRule", TypeShape.of(GetSmartDetectorAlertRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Get a specific Smart Detector alert rule.
* Azure REST API version: 2021-04-01.
*
*/
public static CompletableFuture getSmartDetectorAlertRulePlain(GetSmartDetectorAlertRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:alertsmanagement:getSmartDetectorAlertRule", TypeShape.of(GetSmartDetectorAlertRuleResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy