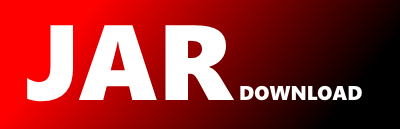
com.pulumi.azurenative.analysisservices.ServerDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.analysisservices;
import com.pulumi.azurenative.analysisservices.enums.ConnectionMode;
import com.pulumi.azurenative.analysisservices.inputs.GatewayDetailsArgs;
import com.pulumi.azurenative.analysisservices.inputs.IPv4FirewallSettingsArgs;
import com.pulumi.azurenative.analysisservices.inputs.ResourceSkuArgs;
import com.pulumi.azurenative.analysisservices.inputs.ServerAdministratorsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServerDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final ServerDetailsArgs Empty = new ServerDetailsArgs();
/**
* A collection of AS server administrators
*
*/
@Import(name="asAdministrators")
private @Nullable Output asAdministrators;
/**
* @return A collection of AS server administrators
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy