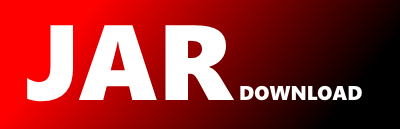
com.pulumi.azurenative.apimanagement.ApiDiagnosticArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.apimanagement.enums.AlwaysLog;
import com.pulumi.azurenative.apimanagement.enums.HttpCorrelationProtocol;
import com.pulumi.azurenative.apimanagement.enums.OperationNameFormat;
import com.pulumi.azurenative.apimanagement.enums.Verbosity;
import com.pulumi.azurenative.apimanagement.inputs.PipelineDiagnosticSettingsArgs;
import com.pulumi.azurenative.apimanagement.inputs.SamplingSettingsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApiDiagnosticArgs extends com.pulumi.resources.ResourceArgs {
public static final ApiDiagnosticArgs Empty = new ApiDiagnosticArgs();
/**
* Specifies for what type of messages sampling settings should not apply.
*
*/
@Import(name="alwaysLog")
private @Nullable Output> alwaysLog;
/**
* @return Specifies for what type of messages sampling settings should not apply.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy