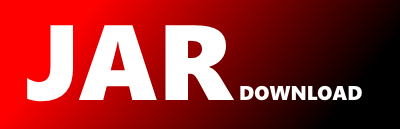
com.pulumi.azurenative.apimanagement.outputs.AdditionalLocationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.azurenative.apimanagement.outputs.ApiManagementServiceSkuPropertiesResponse;
import com.pulumi.azurenative.apimanagement.outputs.VirtualNetworkConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AdditionalLocationResponse {
/**
* @return Property only valid for an Api Management service deployed in multiple locations. This can be used to disable the gateway in this additional location.
*
*/
private @Nullable Boolean disableGateway;
/**
* @return Gateway URL of the API Management service in the Region.
*
*/
private String gatewayRegionalUrl;
/**
* @return The location name of the additional region among Azure Data center regions.
*
*/
private String location;
/**
* @return Property can be used to enable NAT Gateway for this API Management service.
*
*/
private @Nullable String natGatewayState;
/**
* @return Outbound public IPV4 address prefixes associated with NAT Gateway deployed service. Available only for Premium SKU on stv2 platform.
*
*/
private List outboundPublicIPAddresses;
/**
* @return Compute Platform Version running the service.
*
*/
private String platformVersion;
/**
* @return Private Static Load Balanced IP addresses of the API Management service which is deployed in an Internal Virtual Network in a particular additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*
*/
private List privateIPAddresses;
/**
* @return Public Static Load Balanced IP addresses of the API Management service in the additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*
*/
private List publicIPAddresses;
/**
* @return Public Standard SKU IP V4 based IP address to be associated with Virtual Network deployed service in the location. Supported only for Premium SKU being deployed in Virtual Network.
*
*/
private @Nullable String publicIpAddressId;
/**
* @return SKU properties of the API Management service.
*
*/
private ApiManagementServiceSkuPropertiesResponse sku;
/**
* @return Virtual network configuration for the location.
*
*/
private @Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration;
/**
* @return A list of availability zones denoting where the resource needs to come from.
*
*/
private @Nullable List zones;
private AdditionalLocationResponse() {}
/**
* @return Property only valid for an Api Management service deployed in multiple locations. This can be used to disable the gateway in this additional location.
*
*/
public Optional disableGateway() {
return Optional.ofNullable(this.disableGateway);
}
/**
* @return Gateway URL of the API Management service in the Region.
*
*/
public String gatewayRegionalUrl() {
return this.gatewayRegionalUrl;
}
/**
* @return The location name of the additional region among Azure Data center regions.
*
*/
public String location() {
return this.location;
}
/**
* @return Property can be used to enable NAT Gateway for this API Management service.
*
*/
public Optional natGatewayState() {
return Optional.ofNullable(this.natGatewayState);
}
/**
* @return Outbound public IPV4 address prefixes associated with NAT Gateway deployed service. Available only for Premium SKU on stv2 platform.
*
*/
public List outboundPublicIPAddresses() {
return this.outboundPublicIPAddresses;
}
/**
* @return Compute Platform Version running the service.
*
*/
public String platformVersion() {
return this.platformVersion;
}
/**
* @return Private Static Load Balanced IP addresses of the API Management service which is deployed in an Internal Virtual Network in a particular additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*
*/
public List privateIPAddresses() {
return this.privateIPAddresses;
}
/**
* @return Public Static Load Balanced IP addresses of the API Management service in the additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*
*/
public List publicIPAddresses() {
return this.publicIPAddresses;
}
/**
* @return Public Standard SKU IP V4 based IP address to be associated with Virtual Network deployed service in the location. Supported only for Premium SKU being deployed in Virtual Network.
*
*/
public Optional publicIpAddressId() {
return Optional.ofNullable(this.publicIpAddressId);
}
/**
* @return SKU properties of the API Management service.
*
*/
public ApiManagementServiceSkuPropertiesResponse sku() {
return this.sku;
}
/**
* @return Virtual network configuration for the location.
*
*/
public Optional virtualNetworkConfiguration() {
return Optional.ofNullable(this.virtualNetworkConfiguration);
}
/**
* @return A list of availability zones denoting where the resource needs to come from.
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AdditionalLocationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean disableGateway;
private String gatewayRegionalUrl;
private String location;
private @Nullable String natGatewayState;
private List outboundPublicIPAddresses;
private String platformVersion;
private List privateIPAddresses;
private List publicIPAddresses;
private @Nullable String publicIpAddressId;
private ApiManagementServiceSkuPropertiesResponse sku;
private @Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration;
private @Nullable List zones;
public Builder() {}
public Builder(AdditionalLocationResponse defaults) {
Objects.requireNonNull(defaults);
this.disableGateway = defaults.disableGateway;
this.gatewayRegionalUrl = defaults.gatewayRegionalUrl;
this.location = defaults.location;
this.natGatewayState = defaults.natGatewayState;
this.outboundPublicIPAddresses = defaults.outboundPublicIPAddresses;
this.platformVersion = defaults.platformVersion;
this.privateIPAddresses = defaults.privateIPAddresses;
this.publicIPAddresses = defaults.publicIPAddresses;
this.publicIpAddressId = defaults.publicIpAddressId;
this.sku = defaults.sku;
this.virtualNetworkConfiguration = defaults.virtualNetworkConfiguration;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder disableGateway(@Nullable Boolean disableGateway) {
this.disableGateway = disableGateway;
return this;
}
@CustomType.Setter
public Builder gatewayRegionalUrl(String gatewayRegionalUrl) {
if (gatewayRegionalUrl == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "gatewayRegionalUrl");
}
this.gatewayRegionalUrl = gatewayRegionalUrl;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder natGatewayState(@Nullable String natGatewayState) {
this.natGatewayState = natGatewayState;
return this;
}
@CustomType.Setter
public Builder outboundPublicIPAddresses(List outboundPublicIPAddresses) {
if (outboundPublicIPAddresses == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "outboundPublicIPAddresses");
}
this.outboundPublicIPAddresses = outboundPublicIPAddresses;
return this;
}
public Builder outboundPublicIPAddresses(String... outboundPublicIPAddresses) {
return outboundPublicIPAddresses(List.of(outboundPublicIPAddresses));
}
@CustomType.Setter
public Builder platformVersion(String platformVersion) {
if (platformVersion == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "platformVersion");
}
this.platformVersion = platformVersion;
return this;
}
@CustomType.Setter
public Builder privateIPAddresses(List privateIPAddresses) {
if (privateIPAddresses == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "privateIPAddresses");
}
this.privateIPAddresses = privateIPAddresses;
return this;
}
public Builder privateIPAddresses(String... privateIPAddresses) {
return privateIPAddresses(List.of(privateIPAddresses));
}
@CustomType.Setter
public Builder publicIPAddresses(List publicIPAddresses) {
if (publicIPAddresses == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "publicIPAddresses");
}
this.publicIPAddresses = publicIPAddresses;
return this;
}
public Builder publicIPAddresses(String... publicIPAddresses) {
return publicIPAddresses(List.of(publicIPAddresses));
}
@CustomType.Setter
public Builder publicIpAddressId(@Nullable String publicIpAddressId) {
this.publicIpAddressId = publicIpAddressId;
return this;
}
@CustomType.Setter
public Builder sku(ApiManagementServiceSkuPropertiesResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("AdditionalLocationResponse", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder virtualNetworkConfiguration(@Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration) {
this.virtualNetworkConfiguration = virtualNetworkConfiguration;
return this;
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(String... zones) {
return zones(List.of(zones));
}
public AdditionalLocationResponse build() {
final var _resultValue = new AdditionalLocationResponse();
_resultValue.disableGateway = disableGateway;
_resultValue.gatewayRegionalUrl = gatewayRegionalUrl;
_resultValue.location = location;
_resultValue.natGatewayState = natGatewayState;
_resultValue.outboundPublicIPAddresses = outboundPublicIPAddresses;
_resultValue.platformVersion = platformVersion;
_resultValue.privateIPAddresses = privateIPAddresses;
_resultValue.publicIPAddresses = publicIPAddresses;
_resultValue.publicIpAddressId = publicIpAddressId;
_resultValue.sku = sku;
_resultValue.virtualNetworkConfiguration = virtualNetworkConfiguration;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy