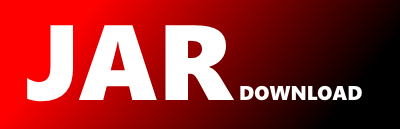
com.pulumi.azurenative.apimanagement.outputs.GetAuthorizationServerResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.azurenative.apimanagement.outputs.TokenBodyParameterContractResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAuthorizationServerResult {
/**
* @return OAuth authorization endpoint. See http://tools.ietf.org/html/rfc6749#section-3.2.
*
*/
private String authorizationEndpoint;
/**
* @return HTTP verbs supported by the authorization endpoint. GET must be always present. POST is optional.
*
*/
private @Nullable List authorizationMethods;
/**
* @return Specifies the mechanism by which access token is passed to the API.
*
*/
private @Nullable List bearerTokenSendingMethods;
/**
* @return Method of authentication supported by the token endpoint of this authorization server. Possible values are Basic and/or Body. When Body is specified, client credentials and other parameters are passed within the request body in the application/x-www-form-urlencoded format.
*
*/
private @Nullable List clientAuthenticationMethod;
/**
* @return Client or app id registered with this authorization server.
*
*/
private String clientId;
/**
* @return Optional reference to a page where client or app registration for this authorization server is performed. Contains absolute URL to entity being referenced.
*
*/
private String clientRegistrationEndpoint;
/**
* @return Client or app secret registered with this authorization server. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
private @Nullable String clientSecret;
/**
* @return Access token scope that is going to be requested by default. Can be overridden at the API level. Should be provided in the form of a string containing space-delimited values.
*
*/
private @Nullable String defaultScope;
/**
* @return Description of the authorization server. Can contain HTML formatting tags.
*
*/
private @Nullable String description;
/**
* @return User-friendly authorization server name.
*
*/
private String displayName;
/**
* @return Form of an authorization grant, which the client uses to request the access token.
*
*/
private List grantTypes;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner password.
*
*/
private @Nullable String resourceOwnerPassword;
/**
* @return Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner username.
*
*/
private @Nullable String resourceOwnerUsername;
/**
* @return If true, authorization server will include state parameter from the authorization request to its response. Client may use state parameter to raise protocol security.
*
*/
private @Nullable Boolean supportState;
/**
* @return Additional parameters required by the token endpoint of this authorization server represented as an array of JSON objects with name and value string properties, i.e. {"name" : "name value", "value": "a value"}.
*
*/
private @Nullable List tokenBodyParameters;
/**
* @return OAuth token endpoint. Contains absolute URI to entity being referenced.
*
*/
private @Nullable String tokenEndpoint;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return If true, the authorization server will be used in the API documentation in the developer portal. False by default if no value is provided.
*
*/
private @Nullable Boolean useInApiDocumentation;
/**
* @return If true, the authorization server may be used in the developer portal test console. True by default if no value is provided.
*
*/
private @Nullable Boolean useInTestConsole;
private GetAuthorizationServerResult() {}
/**
* @return OAuth authorization endpoint. See http://tools.ietf.org/html/rfc6749#section-3.2.
*
*/
public String authorizationEndpoint() {
return this.authorizationEndpoint;
}
/**
* @return HTTP verbs supported by the authorization endpoint. GET must be always present. POST is optional.
*
*/
public List authorizationMethods() {
return this.authorizationMethods == null ? List.of() : this.authorizationMethods;
}
/**
* @return Specifies the mechanism by which access token is passed to the API.
*
*/
public List bearerTokenSendingMethods() {
return this.bearerTokenSendingMethods == null ? List.of() : this.bearerTokenSendingMethods;
}
/**
* @return Method of authentication supported by the token endpoint of this authorization server. Possible values are Basic and/or Body. When Body is specified, client credentials and other parameters are passed within the request body in the application/x-www-form-urlencoded format.
*
*/
public List clientAuthenticationMethod() {
return this.clientAuthenticationMethod == null ? List.of() : this.clientAuthenticationMethod;
}
/**
* @return Client or app id registered with this authorization server.
*
*/
public String clientId() {
return this.clientId;
}
/**
* @return Optional reference to a page where client or app registration for this authorization server is performed. Contains absolute URL to entity being referenced.
*
*/
public String clientRegistrationEndpoint() {
return this.clientRegistrationEndpoint;
}
/**
* @return Client or app secret registered with this authorization server. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
public Optional clientSecret() {
return Optional.ofNullable(this.clientSecret);
}
/**
* @return Access token scope that is going to be requested by default. Can be overridden at the API level. Should be provided in the form of a string containing space-delimited values.
*
*/
public Optional defaultScope() {
return Optional.ofNullable(this.defaultScope);
}
/**
* @return Description of the authorization server. Can contain HTML formatting tags.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return User-friendly authorization server name.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Form of an authorization grant, which the client uses to request the access token.
*
*/
public List grantTypes() {
return this.grantTypes;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner password.
*
*/
public Optional resourceOwnerPassword() {
return Optional.ofNullable(this.resourceOwnerPassword);
}
/**
* @return Can be optionally specified when resource owner password grant type is supported by this authorization server. Default resource owner username.
*
*/
public Optional resourceOwnerUsername() {
return Optional.ofNullable(this.resourceOwnerUsername);
}
/**
* @return If true, authorization server will include state parameter from the authorization request to its response. Client may use state parameter to raise protocol security.
*
*/
public Optional supportState() {
return Optional.ofNullable(this.supportState);
}
/**
* @return Additional parameters required by the token endpoint of this authorization server represented as an array of JSON objects with name and value string properties, i.e. {"name" : "name value", "value": "a value"}.
*
*/
public List tokenBodyParameters() {
return this.tokenBodyParameters == null ? List.of() : this.tokenBodyParameters;
}
/**
* @return OAuth token endpoint. Contains absolute URI to entity being referenced.
*
*/
public Optional tokenEndpoint() {
return Optional.ofNullable(this.tokenEndpoint);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return If true, the authorization server will be used in the API documentation in the developer portal. False by default if no value is provided.
*
*/
public Optional useInApiDocumentation() {
return Optional.ofNullable(this.useInApiDocumentation);
}
/**
* @return If true, the authorization server may be used in the developer portal test console. True by default if no value is provided.
*
*/
public Optional useInTestConsole() {
return Optional.ofNullable(this.useInTestConsole);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAuthorizationServerResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String authorizationEndpoint;
private @Nullable List authorizationMethods;
private @Nullable List bearerTokenSendingMethods;
private @Nullable List clientAuthenticationMethod;
private String clientId;
private String clientRegistrationEndpoint;
private @Nullable String clientSecret;
private @Nullable String defaultScope;
private @Nullable String description;
private String displayName;
private List grantTypes;
private String id;
private String name;
private @Nullable String resourceOwnerPassword;
private @Nullable String resourceOwnerUsername;
private @Nullable Boolean supportState;
private @Nullable List tokenBodyParameters;
private @Nullable String tokenEndpoint;
private String type;
private @Nullable Boolean useInApiDocumentation;
private @Nullable Boolean useInTestConsole;
public Builder() {}
public Builder(GetAuthorizationServerResult defaults) {
Objects.requireNonNull(defaults);
this.authorizationEndpoint = defaults.authorizationEndpoint;
this.authorizationMethods = defaults.authorizationMethods;
this.bearerTokenSendingMethods = defaults.bearerTokenSendingMethods;
this.clientAuthenticationMethod = defaults.clientAuthenticationMethod;
this.clientId = defaults.clientId;
this.clientRegistrationEndpoint = defaults.clientRegistrationEndpoint;
this.clientSecret = defaults.clientSecret;
this.defaultScope = defaults.defaultScope;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.grantTypes = defaults.grantTypes;
this.id = defaults.id;
this.name = defaults.name;
this.resourceOwnerPassword = defaults.resourceOwnerPassword;
this.resourceOwnerUsername = defaults.resourceOwnerUsername;
this.supportState = defaults.supportState;
this.tokenBodyParameters = defaults.tokenBodyParameters;
this.tokenEndpoint = defaults.tokenEndpoint;
this.type = defaults.type;
this.useInApiDocumentation = defaults.useInApiDocumentation;
this.useInTestConsole = defaults.useInTestConsole;
}
@CustomType.Setter
public Builder authorizationEndpoint(String authorizationEndpoint) {
if (authorizationEndpoint == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "authorizationEndpoint");
}
this.authorizationEndpoint = authorizationEndpoint;
return this;
}
@CustomType.Setter
public Builder authorizationMethods(@Nullable List authorizationMethods) {
this.authorizationMethods = authorizationMethods;
return this;
}
public Builder authorizationMethods(String... authorizationMethods) {
return authorizationMethods(List.of(authorizationMethods));
}
@CustomType.Setter
public Builder bearerTokenSendingMethods(@Nullable List bearerTokenSendingMethods) {
this.bearerTokenSendingMethods = bearerTokenSendingMethods;
return this;
}
public Builder bearerTokenSendingMethods(String... bearerTokenSendingMethods) {
return bearerTokenSendingMethods(List.of(bearerTokenSendingMethods));
}
@CustomType.Setter
public Builder clientAuthenticationMethod(@Nullable List clientAuthenticationMethod) {
this.clientAuthenticationMethod = clientAuthenticationMethod;
return this;
}
public Builder clientAuthenticationMethod(String... clientAuthenticationMethod) {
return clientAuthenticationMethod(List.of(clientAuthenticationMethod));
}
@CustomType.Setter
public Builder clientId(String clientId) {
if (clientId == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "clientId");
}
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder clientRegistrationEndpoint(String clientRegistrationEndpoint) {
if (clientRegistrationEndpoint == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "clientRegistrationEndpoint");
}
this.clientRegistrationEndpoint = clientRegistrationEndpoint;
return this;
}
@CustomType.Setter
public Builder clientSecret(@Nullable String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
@CustomType.Setter
public Builder defaultScope(@Nullable String defaultScope) {
this.defaultScope = defaultScope;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder grantTypes(List grantTypes) {
if (grantTypes == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "grantTypes");
}
this.grantTypes = grantTypes;
return this;
}
public Builder grantTypes(String... grantTypes) {
return grantTypes(List.of(grantTypes));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder resourceOwnerPassword(@Nullable String resourceOwnerPassword) {
this.resourceOwnerPassword = resourceOwnerPassword;
return this;
}
@CustomType.Setter
public Builder resourceOwnerUsername(@Nullable String resourceOwnerUsername) {
this.resourceOwnerUsername = resourceOwnerUsername;
return this;
}
@CustomType.Setter
public Builder supportState(@Nullable Boolean supportState) {
this.supportState = supportState;
return this;
}
@CustomType.Setter
public Builder tokenBodyParameters(@Nullable List tokenBodyParameters) {
this.tokenBodyParameters = tokenBodyParameters;
return this;
}
public Builder tokenBodyParameters(TokenBodyParameterContractResponse... tokenBodyParameters) {
return tokenBodyParameters(List.of(tokenBodyParameters));
}
@CustomType.Setter
public Builder tokenEndpoint(@Nullable String tokenEndpoint) {
this.tokenEndpoint = tokenEndpoint;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAuthorizationServerResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder useInApiDocumentation(@Nullable Boolean useInApiDocumentation) {
this.useInApiDocumentation = useInApiDocumentation;
return this;
}
@CustomType.Setter
public Builder useInTestConsole(@Nullable Boolean useInTestConsole) {
this.useInTestConsole = useInTestConsole;
return this;
}
public GetAuthorizationServerResult build() {
final var _resultValue = new GetAuthorizationServerResult();
_resultValue.authorizationEndpoint = authorizationEndpoint;
_resultValue.authorizationMethods = authorizationMethods;
_resultValue.bearerTokenSendingMethods = bearerTokenSendingMethods;
_resultValue.clientAuthenticationMethod = clientAuthenticationMethod;
_resultValue.clientId = clientId;
_resultValue.clientRegistrationEndpoint = clientRegistrationEndpoint;
_resultValue.clientSecret = clientSecret;
_resultValue.defaultScope = defaultScope;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.grantTypes = grantTypes;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.resourceOwnerPassword = resourceOwnerPassword;
_resultValue.resourceOwnerUsername = resourceOwnerUsername;
_resultValue.supportState = supportState;
_resultValue.tokenBodyParameters = tokenBodyParameters;
_resultValue.tokenEndpoint = tokenEndpoint;
_resultValue.type = type;
_resultValue.useInApiDocumentation = useInApiDocumentation;
_resultValue.useInTestConsole = useInTestConsole;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy