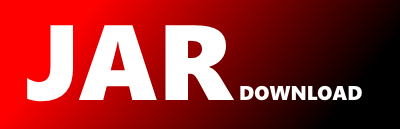
com.pulumi.azurenative.apimanagement.outputs.GetProductResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetProductResult {
/**
* @return whether subscription approval is required. If false, new subscriptions will be approved automatically enabling developers to call the product’s APIs immediately after subscribing. If true, administrators must manually approve the subscription before the developer can any of the product’s APIs. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
private @Nullable Boolean approvalRequired;
/**
* @return Product description. May include HTML formatting tags.
*
*/
private @Nullable String description;
/**
* @return Product name.
*
*/
private String displayName;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return whether product is published or not. Published products are discoverable by users of developer portal. Non published products are visible only to administrators. Default state of Product is notPublished.
*
*/
private @Nullable String state;
/**
* @return Whether a product subscription is required for accessing APIs included in this product. If true, the product is referred to as "protected" and a valid subscription key is required for a request to an API included in the product to succeed. If false, the product is referred to as "open" and requests to an API included in the product can be made without a subscription key. If property is omitted when creating a new product it's value is assumed to be true.
*
*/
private @Nullable Boolean subscriptionRequired;
/**
* @return Whether the number of subscriptions a user can have to this product at the same time. Set to null or omit to allow unlimited per user subscriptions. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
private @Nullable Integer subscriptionsLimit;
/**
* @return Product terms of use. Developers trying to subscribe to the product will be presented and required to accept these terms before they can complete the subscription process.
*
*/
private @Nullable String terms;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetProductResult() {}
/**
* @return whether subscription approval is required. If false, new subscriptions will be approved automatically enabling developers to call the product’s APIs immediately after subscribing. If true, administrators must manually approve the subscription before the developer can any of the product’s APIs. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
public Optional approvalRequired() {
return Optional.ofNullable(this.approvalRequired);
}
/**
* @return Product description. May include HTML formatting tags.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Product name.
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return whether product is published or not. Published products are discoverable by users of developer portal. Non published products are visible only to administrators. Default state of Product is notPublished.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return Whether a product subscription is required for accessing APIs included in this product. If true, the product is referred to as "protected" and a valid subscription key is required for a request to an API included in the product to succeed. If false, the product is referred to as "open" and requests to an API included in the product can be made without a subscription key. If property is omitted when creating a new product it's value is assumed to be true.
*
*/
public Optional subscriptionRequired() {
return Optional.ofNullable(this.subscriptionRequired);
}
/**
* @return Whether the number of subscriptions a user can have to this product at the same time. Set to null or omit to allow unlimited per user subscriptions. Can be present only if subscriptionRequired property is present and has a value of false.
*
*/
public Optional subscriptionsLimit() {
return Optional.ofNullable(this.subscriptionsLimit);
}
/**
* @return Product terms of use. Developers trying to subscribe to the product will be presented and required to accept these terms before they can complete the subscription process.
*
*/
public Optional terms() {
return Optional.ofNullable(this.terms);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetProductResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean approvalRequired;
private @Nullable String description;
private String displayName;
private String id;
private String name;
private @Nullable String state;
private @Nullable Boolean subscriptionRequired;
private @Nullable Integer subscriptionsLimit;
private @Nullable String terms;
private String type;
public Builder() {}
public Builder(GetProductResult defaults) {
Objects.requireNonNull(defaults);
this.approvalRequired = defaults.approvalRequired;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.id = defaults.id;
this.name = defaults.name;
this.state = defaults.state;
this.subscriptionRequired = defaults.subscriptionRequired;
this.subscriptionsLimit = defaults.subscriptionsLimit;
this.terms = defaults.terms;
this.type = defaults.type;
}
@CustomType.Setter
public Builder approvalRequired(@Nullable Boolean approvalRequired) {
this.approvalRequired = approvalRequired;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetProductResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetProductResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetProductResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder subscriptionRequired(@Nullable Boolean subscriptionRequired) {
this.subscriptionRequired = subscriptionRequired;
return this;
}
@CustomType.Setter
public Builder subscriptionsLimit(@Nullable Integer subscriptionsLimit) {
this.subscriptionsLimit = subscriptionsLimit;
return this;
}
@CustomType.Setter
public Builder terms(@Nullable String terms) {
this.terms = terms;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetProductResult", "type");
}
this.type = type;
return this;
}
public GetProductResult build() {
final var _resultValue = new GetProductResult();
_resultValue.approvalRequired = approvalRequired;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.state = state;
_resultValue.subscriptionRequired = subscriptionRequired;
_resultValue.subscriptionsLimit = subscriptionsLimit;
_resultValue.terms = terms;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy