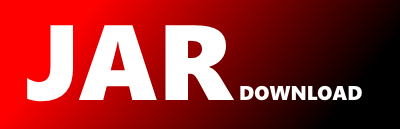
com.pulumi.azurenative.apimanagement.outputs.GetUserResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.azurenative.apimanagement.outputs.GroupContractPropertiesResponse;
import com.pulumi.azurenative.apimanagement.outputs.UserIdentityContractResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetUserResult {
/**
* @return Email address.
*
*/
private @Nullable String email;
/**
* @return First name.
*
*/
private @Nullable String firstName;
/**
* @return Collection of groups user is part of.
*
*/
private List groups;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Collection of user identities.
*
*/
private @Nullable List identities;
/**
* @return Last name.
*
*/
private @Nullable String lastName;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Optional note about a user set by the administrator.
*
*/
private @Nullable String note;
/**
* @return Date of user registration. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
private @Nullable String registrationDate;
/**
* @return Account state. Specifies whether the user is active or not. Blocked users are unable to sign into the developer portal or call any APIs of subscribed products. Default state is Active.
*
*/
private @Nullable String state;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetUserResult() {}
/**
* @return Email address.
*
*/
public Optional email() {
return Optional.ofNullable(this.email);
}
/**
* @return First name.
*
*/
public Optional firstName() {
return Optional.ofNullable(this.firstName);
}
/**
* @return Collection of groups user is part of.
*
*/
public List groups() {
return this.groups;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Collection of user identities.
*
*/
public List identities() {
return this.identities == null ? List.of() : this.identities;
}
/**
* @return Last name.
*
*/
public Optional lastName() {
return Optional.ofNullable(this.lastName);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Optional note about a user set by the administrator.
*
*/
public Optional note() {
return Optional.ofNullable(this.note);
}
/**
* @return Date of user registration. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Optional registrationDate() {
return Optional.ofNullable(this.registrationDate);
}
/**
* @return Account state. Specifies whether the user is active or not. Blocked users are unable to sign into the developer portal or call any APIs of subscribed products. Default state is Active.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetUserResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String email;
private @Nullable String firstName;
private List groups;
private String id;
private @Nullable List identities;
private @Nullable String lastName;
private String name;
private @Nullable String note;
private @Nullable String registrationDate;
private @Nullable String state;
private String type;
public Builder() {}
public Builder(GetUserResult defaults) {
Objects.requireNonNull(defaults);
this.email = defaults.email;
this.firstName = defaults.firstName;
this.groups = defaults.groups;
this.id = defaults.id;
this.identities = defaults.identities;
this.lastName = defaults.lastName;
this.name = defaults.name;
this.note = defaults.note;
this.registrationDate = defaults.registrationDate;
this.state = defaults.state;
this.type = defaults.type;
}
@CustomType.Setter
public Builder email(@Nullable String email) {
this.email = email;
return this;
}
@CustomType.Setter
public Builder firstName(@Nullable String firstName) {
this.firstName = firstName;
return this;
}
@CustomType.Setter
public Builder groups(List groups) {
if (groups == null) {
throw new MissingRequiredPropertyException("GetUserResult", "groups");
}
this.groups = groups;
return this;
}
public Builder groups(GroupContractPropertiesResponse... groups) {
return groups(List.of(groups));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetUserResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identities(@Nullable List identities) {
this.identities = identities;
return this;
}
public Builder identities(UserIdentityContractResponse... identities) {
return identities(List.of(identities));
}
@CustomType.Setter
public Builder lastName(@Nullable String lastName) {
this.lastName = lastName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetUserResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder note(@Nullable String note) {
this.note = note;
return this;
}
@CustomType.Setter
public Builder registrationDate(@Nullable String registrationDate) {
this.registrationDate = registrationDate;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetUserResult", "type");
}
this.type = type;
return this;
}
public GetUserResult build() {
final var _resultValue = new GetUserResult();
_resultValue.email = email;
_resultValue.firstName = firstName;
_resultValue.groups = groups;
_resultValue.id = id;
_resultValue.identities = identities;
_resultValue.lastName = lastName;
_resultValue.name = name;
_resultValue.note = note;
_resultValue.registrationDate = registrationDate;
_resultValue.state = state;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy