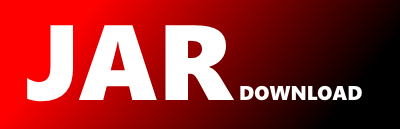
com.pulumi.azurenative.apimanagement.outputs.GetWorkspaceApiResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement.outputs;
import com.pulumi.azurenative.apimanagement.outputs.ApiContactInformationResponse;
import com.pulumi.azurenative.apimanagement.outputs.ApiLicenseInformationResponse;
import com.pulumi.azurenative.apimanagement.outputs.ApiVersionSetContractDetailsResponse;
import com.pulumi.azurenative.apimanagement.outputs.AuthenticationSettingsContractResponse;
import com.pulumi.azurenative.apimanagement.outputs.SubscriptionKeyParameterNamesContractResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetWorkspaceApiResult {
/**
* @return Describes the revision of the API. If no value is provided, default revision 1 is created
*
*/
private @Nullable String apiRevision;
/**
* @return Description of the API Revision.
*
*/
private @Nullable String apiRevisionDescription;
/**
* @return Type of API.
*
*/
private @Nullable String apiType;
/**
* @return Indicates the version identifier of the API if the API is versioned
*
*/
private @Nullable String apiVersion;
/**
* @return Description of the API Version.
*
*/
private @Nullable String apiVersionDescription;
/**
* @return Version set details
*
*/
private @Nullable ApiVersionSetContractDetailsResponse apiVersionSet;
/**
* @return A resource identifier for the related ApiVersionSet.
*
*/
private @Nullable String apiVersionSetId;
/**
* @return Collection of authentication settings included into this API.
*
*/
private @Nullable AuthenticationSettingsContractResponse authenticationSettings;
/**
* @return Contact information for the API.
*
*/
private @Nullable ApiContactInformationResponse contact;
/**
* @return Description of the API. May include HTML formatting tags.
*
*/
private @Nullable String description;
/**
* @return API name. Must be 1 to 300 characters long.
*
*/
private @Nullable String displayName;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Indicates if API revision is current api revision.
*
*/
private @Nullable Boolean isCurrent;
/**
* @return Indicates if API revision is accessible via the gateway.
*
*/
private Boolean isOnline;
/**
* @return License information for the API.
*
*/
private @Nullable ApiLicenseInformationResponse license;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Relative URL uniquely identifying this API and all of its resource paths within the API Management service instance. It is appended to the API endpoint base URL specified during the service instance creation to form a public URL for this API.
*
*/
private String path;
/**
* @return Describes on which protocols the operations in this API can be invoked.
*
*/
private @Nullable List protocols;
/**
* @return Absolute URL of the backend service implementing this API. Cannot be more than 2000 characters long.
*
*/
private @Nullable String serviceUrl;
/**
* @return API identifier of the source API.
*
*/
private @Nullable String sourceApiId;
/**
* @return Protocols over which API is made available.
*
*/
private @Nullable SubscriptionKeyParameterNamesContractResponse subscriptionKeyParameterNames;
/**
* @return Specifies whether an API or Product subscription is required for accessing the API.
*
*/
private @Nullable Boolean subscriptionRequired;
/**
* @return A URL to the Terms of Service for the API. MUST be in the format of a URL.
*
*/
private @Nullable String termsOfServiceUrl;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetWorkspaceApiResult() {}
/**
* @return Describes the revision of the API. If no value is provided, default revision 1 is created
*
*/
public Optional apiRevision() {
return Optional.ofNullable(this.apiRevision);
}
/**
* @return Description of the API Revision.
*
*/
public Optional apiRevisionDescription() {
return Optional.ofNullable(this.apiRevisionDescription);
}
/**
* @return Type of API.
*
*/
public Optional apiType() {
return Optional.ofNullable(this.apiType);
}
/**
* @return Indicates the version identifier of the API if the API is versioned
*
*/
public Optional apiVersion() {
return Optional.ofNullable(this.apiVersion);
}
/**
* @return Description of the API Version.
*
*/
public Optional apiVersionDescription() {
return Optional.ofNullable(this.apiVersionDescription);
}
/**
* @return Version set details
*
*/
public Optional apiVersionSet() {
return Optional.ofNullable(this.apiVersionSet);
}
/**
* @return A resource identifier for the related ApiVersionSet.
*
*/
public Optional apiVersionSetId() {
return Optional.ofNullable(this.apiVersionSetId);
}
/**
* @return Collection of authentication settings included into this API.
*
*/
public Optional authenticationSettings() {
return Optional.ofNullable(this.authenticationSettings);
}
/**
* @return Contact information for the API.
*
*/
public Optional contact() {
return Optional.ofNullable(this.contact);
}
/**
* @return Description of the API. May include HTML formatting tags.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return API name. Must be 1 to 300 characters long.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Indicates if API revision is current api revision.
*
*/
public Optional isCurrent() {
return Optional.ofNullable(this.isCurrent);
}
/**
* @return Indicates if API revision is accessible via the gateway.
*
*/
public Boolean isOnline() {
return this.isOnline;
}
/**
* @return License information for the API.
*
*/
public Optional license() {
return Optional.ofNullable(this.license);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Relative URL uniquely identifying this API and all of its resource paths within the API Management service instance. It is appended to the API endpoint base URL specified during the service instance creation to form a public URL for this API.
*
*/
public String path() {
return this.path;
}
/**
* @return Describes on which protocols the operations in this API can be invoked.
*
*/
public List protocols() {
return this.protocols == null ? List.of() : this.protocols;
}
/**
* @return Absolute URL of the backend service implementing this API. Cannot be more than 2000 characters long.
*
*/
public Optional serviceUrl() {
return Optional.ofNullable(this.serviceUrl);
}
/**
* @return API identifier of the source API.
*
*/
public Optional sourceApiId() {
return Optional.ofNullable(this.sourceApiId);
}
/**
* @return Protocols over which API is made available.
*
*/
public Optional subscriptionKeyParameterNames() {
return Optional.ofNullable(this.subscriptionKeyParameterNames);
}
/**
* @return Specifies whether an API or Product subscription is required for accessing the API.
*
*/
public Optional subscriptionRequired() {
return Optional.ofNullable(this.subscriptionRequired);
}
/**
* @return A URL to the Terms of Service for the API. MUST be in the format of a URL.
*
*/
public Optional termsOfServiceUrl() {
return Optional.ofNullable(this.termsOfServiceUrl);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetWorkspaceApiResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String apiRevision;
private @Nullable String apiRevisionDescription;
private @Nullable String apiType;
private @Nullable String apiVersion;
private @Nullable String apiVersionDescription;
private @Nullable ApiVersionSetContractDetailsResponse apiVersionSet;
private @Nullable String apiVersionSetId;
private @Nullable AuthenticationSettingsContractResponse authenticationSettings;
private @Nullable ApiContactInformationResponse contact;
private @Nullable String description;
private @Nullable String displayName;
private String id;
private @Nullable Boolean isCurrent;
private Boolean isOnline;
private @Nullable ApiLicenseInformationResponse license;
private String name;
private String path;
private @Nullable List protocols;
private @Nullable String serviceUrl;
private @Nullable String sourceApiId;
private @Nullable SubscriptionKeyParameterNamesContractResponse subscriptionKeyParameterNames;
private @Nullable Boolean subscriptionRequired;
private @Nullable String termsOfServiceUrl;
private String type;
public Builder() {}
public Builder(GetWorkspaceApiResult defaults) {
Objects.requireNonNull(defaults);
this.apiRevision = defaults.apiRevision;
this.apiRevisionDescription = defaults.apiRevisionDescription;
this.apiType = defaults.apiType;
this.apiVersion = defaults.apiVersion;
this.apiVersionDescription = defaults.apiVersionDescription;
this.apiVersionSet = defaults.apiVersionSet;
this.apiVersionSetId = defaults.apiVersionSetId;
this.authenticationSettings = defaults.authenticationSettings;
this.contact = defaults.contact;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.id = defaults.id;
this.isCurrent = defaults.isCurrent;
this.isOnline = defaults.isOnline;
this.license = defaults.license;
this.name = defaults.name;
this.path = defaults.path;
this.protocols = defaults.protocols;
this.serviceUrl = defaults.serviceUrl;
this.sourceApiId = defaults.sourceApiId;
this.subscriptionKeyParameterNames = defaults.subscriptionKeyParameterNames;
this.subscriptionRequired = defaults.subscriptionRequired;
this.termsOfServiceUrl = defaults.termsOfServiceUrl;
this.type = defaults.type;
}
@CustomType.Setter
public Builder apiRevision(@Nullable String apiRevision) {
this.apiRevision = apiRevision;
return this;
}
@CustomType.Setter
public Builder apiRevisionDescription(@Nullable String apiRevisionDescription) {
this.apiRevisionDescription = apiRevisionDescription;
return this;
}
@CustomType.Setter
public Builder apiType(@Nullable String apiType) {
this.apiType = apiType;
return this;
}
@CustomType.Setter
public Builder apiVersion(@Nullable String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
@CustomType.Setter
public Builder apiVersionDescription(@Nullable String apiVersionDescription) {
this.apiVersionDescription = apiVersionDescription;
return this;
}
@CustomType.Setter
public Builder apiVersionSet(@Nullable ApiVersionSetContractDetailsResponse apiVersionSet) {
this.apiVersionSet = apiVersionSet;
return this;
}
@CustomType.Setter
public Builder apiVersionSetId(@Nullable String apiVersionSetId) {
this.apiVersionSetId = apiVersionSetId;
return this;
}
@CustomType.Setter
public Builder authenticationSettings(@Nullable AuthenticationSettingsContractResponse authenticationSettings) {
this.authenticationSettings = authenticationSettings;
return this;
}
@CustomType.Setter
public Builder contact(@Nullable ApiContactInformationResponse contact) {
this.contact = contact;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetWorkspaceApiResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isCurrent(@Nullable Boolean isCurrent) {
this.isCurrent = isCurrent;
return this;
}
@CustomType.Setter
public Builder isOnline(Boolean isOnline) {
if (isOnline == null) {
throw new MissingRequiredPropertyException("GetWorkspaceApiResult", "isOnline");
}
this.isOnline = isOnline;
return this;
}
@CustomType.Setter
public Builder license(@Nullable ApiLicenseInformationResponse license) {
this.license = license;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetWorkspaceApiResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder path(String path) {
if (path == null) {
throw new MissingRequiredPropertyException("GetWorkspaceApiResult", "path");
}
this.path = path;
return this;
}
@CustomType.Setter
public Builder protocols(@Nullable List protocols) {
this.protocols = protocols;
return this;
}
public Builder protocols(String... protocols) {
return protocols(List.of(protocols));
}
@CustomType.Setter
public Builder serviceUrl(@Nullable String serviceUrl) {
this.serviceUrl = serviceUrl;
return this;
}
@CustomType.Setter
public Builder sourceApiId(@Nullable String sourceApiId) {
this.sourceApiId = sourceApiId;
return this;
}
@CustomType.Setter
public Builder subscriptionKeyParameterNames(@Nullable SubscriptionKeyParameterNamesContractResponse subscriptionKeyParameterNames) {
this.subscriptionKeyParameterNames = subscriptionKeyParameterNames;
return this;
}
@CustomType.Setter
public Builder subscriptionRequired(@Nullable Boolean subscriptionRequired) {
this.subscriptionRequired = subscriptionRequired;
return this;
}
@CustomType.Setter
public Builder termsOfServiceUrl(@Nullable String termsOfServiceUrl) {
this.termsOfServiceUrl = termsOfServiceUrl;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetWorkspaceApiResult", "type");
}
this.type = type;
return this;
}
public GetWorkspaceApiResult build() {
final var _resultValue = new GetWorkspaceApiResult();
_resultValue.apiRevision = apiRevision;
_resultValue.apiRevisionDescription = apiRevisionDescription;
_resultValue.apiType = apiType;
_resultValue.apiVersion = apiVersion;
_resultValue.apiVersionDescription = apiVersionDescription;
_resultValue.apiVersionSet = apiVersionSet;
_resultValue.apiVersionSetId = apiVersionSetId;
_resultValue.authenticationSettings = authenticationSettings;
_resultValue.contact = contact;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.id = id;
_resultValue.isCurrent = isCurrent;
_resultValue.isOnline = isOnline;
_resultValue.license = license;
_resultValue.name = name;
_resultValue.path = path;
_resultValue.protocols = protocols;
_resultValue.serviceUrl = serviceUrl;
_resultValue.sourceApiId = sourceApiId;
_resultValue.subscriptionKeyParameterNames = subscriptionKeyParameterNames;
_resultValue.subscriptionRequired = subscriptionRequired;
_resultValue.termsOfServiceUrl = termsOfServiceUrl;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy