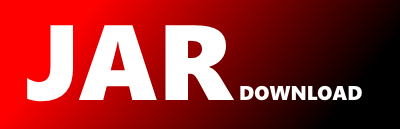
com.pulumi.azurenative.app.inputs.ContainerAppProbeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.enums.Type;
import com.pulumi.azurenative.app.inputs.ContainerAppProbeHttpGetArgs;
import com.pulumi.azurenative.app.inputs.ContainerAppProbeTcpSocketArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Probe describes a health check to be performed against a container to determine whether it is alive or ready to receive traffic.
*
*/
public final class ContainerAppProbeArgs extends com.pulumi.resources.ResourceArgs {
public static final ContainerAppProbeArgs Empty = new ContainerAppProbeArgs();
/**
* Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
*/
@Import(name="failureThreshold")
private @Nullable Output failureThreshold;
/**
* @return Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy