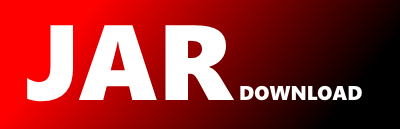
com.pulumi.azurenative.app.inputs.DaprArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.enums.AppProtocol;
import com.pulumi.azurenative.app.enums.LogLevel;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Container App Dapr configuration.
*
*/
public final class DaprArgs extends com.pulumi.resources.ResourceArgs {
public static final DaprArgs Empty = new DaprArgs();
/**
* Dapr application identifier
*
*/
@Import(name="appId")
private @Nullable Output appId;
/**
* @return Dapr application identifier
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy