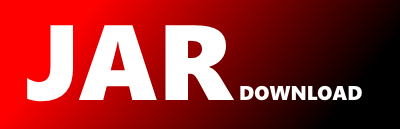
com.pulumi.azurenative.app.inputs.IngressArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.enums.IngressClientCertificateMode;
import com.pulumi.azurenative.app.enums.IngressTransportMethod;
import com.pulumi.azurenative.app.inputs.CorsPolicyArgs;
import com.pulumi.azurenative.app.inputs.CustomDomainArgs;
import com.pulumi.azurenative.app.inputs.IpSecurityRestrictionRuleArgs;
import com.pulumi.azurenative.app.inputs.TrafficWeightArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Container App Ingress configuration.
*
*/
public final class IngressArgs extends com.pulumi.resources.ResourceArgs {
public static final IngressArgs Empty = new IngressArgs();
/**
* Bool indicating if HTTP connections to is allowed. If set to false HTTP connections are automatically redirected to HTTPS connections
*
*/
@Import(name="allowInsecure")
private @Nullable Output allowInsecure;
/**
* @return Bool indicating if HTTP connections to is allowed. If set to false HTTP connections are automatically redirected to HTTPS connections
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy