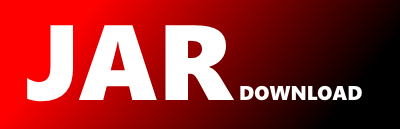
com.pulumi.azurenative.app.inputs.JobConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.enums.TriggerType;
import com.pulumi.azurenative.app.inputs.JobConfigurationEventTriggerConfigArgs;
import com.pulumi.azurenative.app.inputs.JobConfigurationManualTriggerConfigArgs;
import com.pulumi.azurenative.app.inputs.JobConfigurationScheduleTriggerConfigArgs;
import com.pulumi.azurenative.app.inputs.RegistryCredentialsArgs;
import com.pulumi.azurenative.app.inputs.SecretArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Non versioned Container Apps Job configuration properties
*
*/
public final class JobConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final JobConfigurationArgs Empty = new JobConfigurationArgs();
/**
* Trigger configuration of an event driven job.
*
*/
@Import(name="eventTriggerConfig")
private @Nullable Output eventTriggerConfig;
/**
* @return Trigger configuration of an event driven job.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy