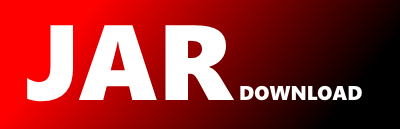
com.pulumi.azurenative.app.outputs.DaprResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DaprResponse {
/**
* @return Dapr application identifier
*
*/
private @Nullable String appId;
/**
* @return Tells Dapr which port your application is listening on
*
*/
private @Nullable Integer appPort;
/**
* @return Tells Dapr which protocol your application is using. Valid options are http and grpc. Default is http
*
*/
private @Nullable String appProtocol;
/**
* @return Enables API logging for the Dapr sidecar
*
*/
private @Nullable Boolean enableApiLogging;
/**
* @return Boolean indicating if the Dapr side car is enabled
*
*/
private @Nullable Boolean enabled;
/**
* @return Increasing max size of request body http and grpc servers parameter in MB to handle uploading of big files. Default is 4 MB.
*
*/
private @Nullable Integer httpMaxRequestSize;
/**
* @return Dapr max size of http header read buffer in KB to handle when sending multi-KB headers. Default is 65KB.
*
*/
private @Nullable Integer httpReadBufferSize;
/**
* @return Sets the log level for the Dapr sidecar. Allowed values are debug, info, warn, error. Default is info.
*
*/
private @Nullable String logLevel;
private DaprResponse() {}
/**
* @return Dapr application identifier
*
*/
public Optional appId() {
return Optional.ofNullable(this.appId);
}
/**
* @return Tells Dapr which port your application is listening on
*
*/
public Optional appPort() {
return Optional.ofNullable(this.appPort);
}
/**
* @return Tells Dapr which protocol your application is using. Valid options are http and grpc. Default is http
*
*/
public Optional appProtocol() {
return Optional.ofNullable(this.appProtocol);
}
/**
* @return Enables API logging for the Dapr sidecar
*
*/
public Optional enableApiLogging() {
return Optional.ofNullable(this.enableApiLogging);
}
/**
* @return Boolean indicating if the Dapr side car is enabled
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* @return Increasing max size of request body http and grpc servers parameter in MB to handle uploading of big files. Default is 4 MB.
*
*/
public Optional httpMaxRequestSize() {
return Optional.ofNullable(this.httpMaxRequestSize);
}
/**
* @return Dapr max size of http header read buffer in KB to handle when sending multi-KB headers. Default is 65KB.
*
*/
public Optional httpReadBufferSize() {
return Optional.ofNullable(this.httpReadBufferSize);
}
/**
* @return Sets the log level for the Dapr sidecar. Allowed values are debug, info, warn, error. Default is info.
*
*/
public Optional logLevel() {
return Optional.ofNullable(this.logLevel);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DaprResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String appId;
private @Nullable Integer appPort;
private @Nullable String appProtocol;
private @Nullable Boolean enableApiLogging;
private @Nullable Boolean enabled;
private @Nullable Integer httpMaxRequestSize;
private @Nullable Integer httpReadBufferSize;
private @Nullable String logLevel;
public Builder() {}
public Builder(DaprResponse defaults) {
Objects.requireNonNull(defaults);
this.appId = defaults.appId;
this.appPort = defaults.appPort;
this.appProtocol = defaults.appProtocol;
this.enableApiLogging = defaults.enableApiLogging;
this.enabled = defaults.enabled;
this.httpMaxRequestSize = defaults.httpMaxRequestSize;
this.httpReadBufferSize = defaults.httpReadBufferSize;
this.logLevel = defaults.logLevel;
}
@CustomType.Setter
public Builder appId(@Nullable String appId) {
this.appId = appId;
return this;
}
@CustomType.Setter
public Builder appPort(@Nullable Integer appPort) {
this.appPort = appPort;
return this;
}
@CustomType.Setter
public Builder appProtocol(@Nullable String appProtocol) {
this.appProtocol = appProtocol;
return this;
}
@CustomType.Setter
public Builder enableApiLogging(@Nullable Boolean enableApiLogging) {
this.enableApiLogging = enableApiLogging;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder httpMaxRequestSize(@Nullable Integer httpMaxRequestSize) {
this.httpMaxRequestSize = httpMaxRequestSize;
return this;
}
@CustomType.Setter
public Builder httpReadBufferSize(@Nullable Integer httpReadBufferSize) {
this.httpReadBufferSize = httpReadBufferSize;
return this;
}
@CustomType.Setter
public Builder logLevel(@Nullable String logLevel) {
this.logLevel = logLevel;
return this;
}
public DaprResponse build() {
final var _resultValue = new DaprResponse();
_resultValue.appId = appId;
_resultValue.appPort = appPort;
_resultValue.appProtocol = appProtocol;
_resultValue.enableApiLogging = enableApiLogging;
_resultValue.enabled = enabled;
_resultValue.httpMaxRequestSize = httpMaxRequestSize;
_resultValue.httpReadBufferSize = httpReadBufferSize;
_resultValue.logLevel = logLevel;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy