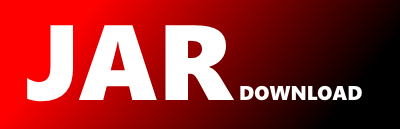
com.pulumi.azurenative.app.outputs.IngressResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.outputs;
import com.pulumi.azurenative.app.outputs.CorsPolicyResponse;
import com.pulumi.azurenative.app.outputs.CustomDomainResponse;
import com.pulumi.azurenative.app.outputs.IpSecurityRestrictionRuleResponse;
import com.pulumi.azurenative.app.outputs.TrafficWeightResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IngressResponse {
/**
* @return Bool indicating if HTTP connections to is allowed. If set to false HTTP connections are automatically redirected to HTTPS connections
*
*/
private @Nullable Boolean allowInsecure;
/**
* @return Client certificate mode for mTLS authentication. Ignore indicates server drops client certificate on forwarding. Accept indicates server forwards client certificate but does not require a client certificate. Require indicates server requires a client certificate.
*
*/
private @Nullable String clientCertificateMode;
/**
* @return CORS policy for container app
*
*/
private @Nullable CorsPolicyResponse corsPolicy;
/**
* @return custom domain bindings for Container Apps' hostnames.
*
*/
private @Nullable List customDomains;
/**
* @return Exposed Port in containers for TCP traffic from ingress
*
*/
private @Nullable Integer exposedPort;
/**
* @return Bool indicating if app exposes an external http endpoint
*
*/
private @Nullable Boolean external;
/**
* @return Hostname.
*
*/
private String fqdn;
/**
* @return Rules to restrict incoming IP address.
*
*/
private @Nullable List ipSecurityRestrictions;
/**
* @return Target Port in containers for traffic from ingress
*
*/
private @Nullable Integer targetPort;
/**
* @return Traffic weights for app's revisions
*
*/
private @Nullable List traffic;
/**
* @return Ingress transport protocol
*
*/
private @Nullable String transport;
private IngressResponse() {}
/**
* @return Bool indicating if HTTP connections to is allowed. If set to false HTTP connections are automatically redirected to HTTPS connections
*
*/
public Optional allowInsecure() {
return Optional.ofNullable(this.allowInsecure);
}
/**
* @return Client certificate mode for mTLS authentication. Ignore indicates server drops client certificate on forwarding. Accept indicates server forwards client certificate but does not require a client certificate. Require indicates server requires a client certificate.
*
*/
public Optional clientCertificateMode() {
return Optional.ofNullable(this.clientCertificateMode);
}
/**
* @return CORS policy for container app
*
*/
public Optional corsPolicy() {
return Optional.ofNullable(this.corsPolicy);
}
/**
* @return custom domain bindings for Container Apps' hostnames.
*
*/
public List customDomains() {
return this.customDomains == null ? List.of() : this.customDomains;
}
/**
* @return Exposed Port in containers for TCP traffic from ingress
*
*/
public Optional exposedPort() {
return Optional.ofNullable(this.exposedPort);
}
/**
* @return Bool indicating if app exposes an external http endpoint
*
*/
public Optional external() {
return Optional.ofNullable(this.external);
}
/**
* @return Hostname.
*
*/
public String fqdn() {
return this.fqdn;
}
/**
* @return Rules to restrict incoming IP address.
*
*/
public List ipSecurityRestrictions() {
return this.ipSecurityRestrictions == null ? List.of() : this.ipSecurityRestrictions;
}
/**
* @return Target Port in containers for traffic from ingress
*
*/
public Optional targetPort() {
return Optional.ofNullable(this.targetPort);
}
/**
* @return Traffic weights for app's revisions
*
*/
public List traffic() {
return this.traffic == null ? List.of() : this.traffic;
}
/**
* @return Ingress transport protocol
*
*/
public Optional transport() {
return Optional.ofNullable(this.transport);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IngressResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowInsecure;
private @Nullable String clientCertificateMode;
private @Nullable CorsPolicyResponse corsPolicy;
private @Nullable List customDomains;
private @Nullable Integer exposedPort;
private @Nullable Boolean external;
private String fqdn;
private @Nullable List ipSecurityRestrictions;
private @Nullable Integer targetPort;
private @Nullable List traffic;
private @Nullable String transport;
public Builder() {}
public Builder(IngressResponse defaults) {
Objects.requireNonNull(defaults);
this.allowInsecure = defaults.allowInsecure;
this.clientCertificateMode = defaults.clientCertificateMode;
this.corsPolicy = defaults.corsPolicy;
this.customDomains = defaults.customDomains;
this.exposedPort = defaults.exposedPort;
this.external = defaults.external;
this.fqdn = defaults.fqdn;
this.ipSecurityRestrictions = defaults.ipSecurityRestrictions;
this.targetPort = defaults.targetPort;
this.traffic = defaults.traffic;
this.transport = defaults.transport;
}
@CustomType.Setter
public Builder allowInsecure(@Nullable Boolean allowInsecure) {
this.allowInsecure = allowInsecure;
return this;
}
@CustomType.Setter
public Builder clientCertificateMode(@Nullable String clientCertificateMode) {
this.clientCertificateMode = clientCertificateMode;
return this;
}
@CustomType.Setter
public Builder corsPolicy(@Nullable CorsPolicyResponse corsPolicy) {
this.corsPolicy = corsPolicy;
return this;
}
@CustomType.Setter
public Builder customDomains(@Nullable List customDomains) {
this.customDomains = customDomains;
return this;
}
public Builder customDomains(CustomDomainResponse... customDomains) {
return customDomains(List.of(customDomains));
}
@CustomType.Setter
public Builder exposedPort(@Nullable Integer exposedPort) {
this.exposedPort = exposedPort;
return this;
}
@CustomType.Setter
public Builder external(@Nullable Boolean external) {
this.external = external;
return this;
}
@CustomType.Setter
public Builder fqdn(String fqdn) {
if (fqdn == null) {
throw new MissingRequiredPropertyException("IngressResponse", "fqdn");
}
this.fqdn = fqdn;
return this;
}
@CustomType.Setter
public Builder ipSecurityRestrictions(@Nullable List ipSecurityRestrictions) {
this.ipSecurityRestrictions = ipSecurityRestrictions;
return this;
}
public Builder ipSecurityRestrictions(IpSecurityRestrictionRuleResponse... ipSecurityRestrictions) {
return ipSecurityRestrictions(List.of(ipSecurityRestrictions));
}
@CustomType.Setter
public Builder targetPort(@Nullable Integer targetPort) {
this.targetPort = targetPort;
return this;
}
@CustomType.Setter
public Builder traffic(@Nullable List traffic) {
this.traffic = traffic;
return this;
}
public Builder traffic(TrafficWeightResponse... traffic) {
return traffic(List.of(traffic));
}
@CustomType.Setter
public Builder transport(@Nullable String transport) {
this.transport = transport;
return this;
}
public IngressResponse build() {
final var _resultValue = new IngressResponse();
_resultValue.allowInsecure = allowInsecure;
_resultValue.clientCertificateMode = clientCertificateMode;
_resultValue.corsPolicy = corsPolicy;
_resultValue.customDomains = customDomains;
_resultValue.exposedPort = exposedPort;
_resultValue.external = external;
_resultValue.fqdn = fqdn;
_resultValue.ipSecurityRestrictions = ipSecurityRestrictions;
_resultValue.targetPort = targetPort;
_resultValue.traffic = traffic;
_resultValue.transport = transport;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy