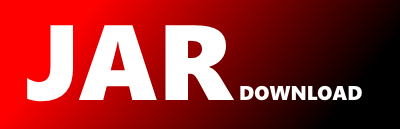
com.pulumi.azurenative.appconfiguration.outputs.GetKeyValueResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appconfiguration.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetKeyValueResult {
/**
* @return The content type of the key-value's value.
* Providing a proper content-type can enable transformations of values when they are retrieved by applications.
*
*/
private @Nullable String contentType;
/**
* @return An ETag indicating the state of a key-value within a configuration store.
*
*/
private String eTag;
/**
* @return The resource ID.
*
*/
private String id;
/**
* @return The primary identifier of a key-value.
* The key is used in unison with the label to uniquely identify a key-value.
*
*/
private String key;
/**
* @return A value used to group key-values.
* The label is used in unison with the key to uniquely identify a key-value.
*
*/
private String label;
/**
* @return The last time a modifying operation was performed on the given key-value.
*
*/
private String lastModified;
/**
* @return A value indicating whether the key-value is locked.
* A locked key-value may not be modified until it is unlocked.
*
*/
private Boolean locked;
/**
* @return The name of the resource.
*
*/
private String name;
/**
* @return A dictionary of tags that can help identify what a key-value may be applicable for.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource.
*
*/
private String type;
/**
* @return The value of the key-value.
*
*/
private @Nullable String value;
private GetKeyValueResult() {}
/**
* @return The content type of the key-value's value.
* Providing a proper content-type can enable transformations of values when they are retrieved by applications.
*
*/
public Optional contentType() {
return Optional.ofNullable(this.contentType);
}
/**
* @return An ETag indicating the state of a key-value within a configuration store.
*
*/
public String eTag() {
return this.eTag;
}
/**
* @return The resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return The primary identifier of a key-value.
* The key is used in unison with the label to uniquely identify a key-value.
*
*/
public String key() {
return this.key;
}
/**
* @return A value used to group key-values.
* The label is used in unison with the key to uniquely identify a key-value.
*
*/
public String label() {
return this.label;
}
/**
* @return The last time a modifying operation was performed on the given key-value.
*
*/
public String lastModified() {
return this.lastModified;
}
/**
* @return A value indicating whether the key-value is locked.
* A locked key-value may not be modified until it is unlocked.
*
*/
public Boolean locked() {
return this.locked;
}
/**
* @return The name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return A dictionary of tags that can help identify what a key-value may be applicable for.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The value of the key-value.
*
*/
public Optional value() {
return Optional.ofNullable(this.value);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetKeyValueResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String contentType;
private String eTag;
private String id;
private String key;
private String label;
private String lastModified;
private Boolean locked;
private String name;
private @Nullable Map tags;
private String type;
private @Nullable String value;
public Builder() {}
public Builder(GetKeyValueResult defaults) {
Objects.requireNonNull(defaults);
this.contentType = defaults.contentType;
this.eTag = defaults.eTag;
this.id = defaults.id;
this.key = defaults.key;
this.label = defaults.label;
this.lastModified = defaults.lastModified;
this.locked = defaults.locked;
this.name = defaults.name;
this.tags = defaults.tags;
this.type = defaults.type;
this.value = defaults.value;
}
@CustomType.Setter
public Builder contentType(@Nullable String contentType) {
this.contentType = contentType;
return this;
}
@CustomType.Setter
public Builder eTag(String eTag) {
if (eTag == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "eTag");
}
this.eTag = eTag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder key(String key) {
if (key == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "key");
}
this.key = key;
return this;
}
@CustomType.Setter
public Builder label(String label) {
if (label == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "label");
}
this.label = label;
return this;
}
@CustomType.Setter
public Builder lastModified(String lastModified) {
if (lastModified == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "lastModified");
}
this.lastModified = lastModified;
return this;
}
@CustomType.Setter
public Builder locked(Boolean locked) {
if (locked == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "locked");
}
this.locked = locked;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetKeyValueResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder value(@Nullable String value) {
this.value = value;
return this;
}
public GetKeyValueResult build() {
final var _resultValue = new GetKeyValueResult();
_resultValue.contentType = contentType;
_resultValue.eTag = eTag;
_resultValue.id = id;
_resultValue.key = key;
_resultValue.label = label;
_resultValue.lastModified = lastModified;
_resultValue.locked = locked;
_resultValue.name = name;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.value = value;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy