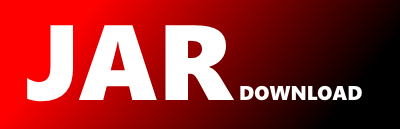
com.pulumi.azurenative.appplatform.inputs.NetworkProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.inputs;
import com.pulumi.azurenative.appplatform.inputs.IngressConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Service network profile payload
*
*/
public final class NetworkProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkProfileArgs Empty = new NetworkProfileArgs();
/**
* Name of the resource group containing network resources for customer apps in Azure Spring Apps
*
*/
@Import(name="appNetworkResourceGroup")
private @Nullable Output appNetworkResourceGroup;
/**
* @return Name of the resource group containing network resources for customer apps in Azure Spring Apps
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy