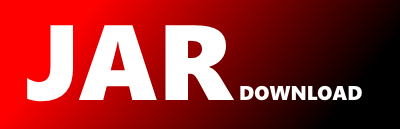
com.pulumi.azurenative.appplatform.outputs.GitPatternRepositoryResponse Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GitPatternRepositoryResponse {
/**
* @return Public sshKey of git repository.
*
*/
private @Nullable String hostKey;
/**
* @return SshKey algorithm of git repository.
*
*/
private @Nullable String hostKeyAlgorithm;
/**
* @return Label of the repository
*
*/
private @Nullable String label;
/**
* @return Name of the repository
*
*/
private String name;
/**
* @return Password of git repository basic auth.
*
*/
private @Nullable String password;
/**
* @return Collection of pattern of the repository
*
*/
private @Nullable List pattern;
/**
* @return Private sshKey algorithm of git repository.
*
*/
private @Nullable String privateKey;
/**
* @return Searching path of the repository
*
*/
private @Nullable List searchPaths;
/**
* @return Strict host key checking or not.
*
*/
private @Nullable Boolean strictHostKeyChecking;
/**
* @return URI of the repository
*
*/
private String uri;
/**
* @return Username of git repository basic auth.
*
*/
private @Nullable String username;
private GitPatternRepositoryResponse() {}
/**
* @return Public sshKey of git repository.
*
*/
public Optional hostKey() {
return Optional.ofNullable(this.hostKey);
}
/**
* @return SshKey algorithm of git repository.
*
*/
public Optional hostKeyAlgorithm() {
return Optional.ofNullable(this.hostKeyAlgorithm);
}
/**
* @return Label of the repository
*
*/
public Optional label() {
return Optional.ofNullable(this.label);
}
/**
* @return Name of the repository
*
*/
public String name() {
return this.name;
}
/**
* @return Password of git repository basic auth.
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return Collection of pattern of the repository
*
*/
public List pattern() {
return this.pattern == null ? List.of() : this.pattern;
}
/**
* @return Private sshKey algorithm of git repository.
*
*/
public Optional privateKey() {
return Optional.ofNullable(this.privateKey);
}
/**
* @return Searching path of the repository
*
*/
public List searchPaths() {
return this.searchPaths == null ? List.of() : this.searchPaths;
}
/**
* @return Strict host key checking or not.
*
*/
public Optional strictHostKeyChecking() {
return Optional.ofNullable(this.strictHostKeyChecking);
}
/**
* @return URI of the repository
*
*/
public String uri() {
return this.uri;
}
/**
* @return Username of git repository basic auth.
*
*/
public Optional username() {
return Optional.ofNullable(this.username);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GitPatternRepositoryResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String hostKey;
private @Nullable String hostKeyAlgorithm;
private @Nullable String label;
private String name;
private @Nullable String password;
private @Nullable List pattern;
private @Nullable String privateKey;
private @Nullable List searchPaths;
private @Nullable Boolean strictHostKeyChecking;
private String uri;
private @Nullable String username;
public Builder() {}
public Builder(GitPatternRepositoryResponse defaults) {
Objects.requireNonNull(defaults);
this.hostKey = defaults.hostKey;
this.hostKeyAlgorithm = defaults.hostKeyAlgorithm;
this.label = defaults.label;
this.name = defaults.name;
this.password = defaults.password;
this.pattern = defaults.pattern;
this.privateKey = defaults.privateKey;
this.searchPaths = defaults.searchPaths;
this.strictHostKeyChecking = defaults.strictHostKeyChecking;
this.uri = defaults.uri;
this.username = defaults.username;
}
@CustomType.Setter
public Builder hostKey(@Nullable String hostKey) {
this.hostKey = hostKey;
return this;
}
@CustomType.Setter
public Builder hostKeyAlgorithm(@Nullable String hostKeyAlgorithm) {
this.hostKeyAlgorithm = hostKeyAlgorithm;
return this;
}
@CustomType.Setter
public Builder label(@Nullable String label) {
this.label = label;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GitPatternRepositoryResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder pattern(@Nullable List pattern) {
this.pattern = pattern;
return this;
}
public Builder pattern(String... pattern) {
return pattern(List.of(pattern));
}
@CustomType.Setter
public Builder privateKey(@Nullable String privateKey) {
this.privateKey = privateKey;
return this;
}
@CustomType.Setter
public Builder searchPaths(@Nullable List searchPaths) {
this.searchPaths = searchPaths;
return this;
}
public Builder searchPaths(String... searchPaths) {
return searchPaths(List.of(searchPaths));
}
@CustomType.Setter
public Builder strictHostKeyChecking(@Nullable Boolean strictHostKeyChecking) {
this.strictHostKeyChecking = strictHostKeyChecking;
return this;
}
@CustomType.Setter
public Builder uri(String uri) {
if (uri == null) {
throw new MissingRequiredPropertyException("GitPatternRepositoryResponse", "uri");
}
this.uri = uri;
return this;
}
@CustomType.Setter
public Builder username(@Nullable String username) {
this.username = username;
return this;
}
public GitPatternRepositoryResponse build() {
final var _resultValue = new GitPatternRepositoryResponse();
_resultValue.hostKey = hostKey;
_resultValue.hostKeyAlgorithm = hostKeyAlgorithm;
_resultValue.label = label;
_resultValue.name = name;
_resultValue.password = password;
_resultValue.pattern = pattern;
_resultValue.privateKey = privateKey;
_resultValue.searchPaths = searchPaths;
_resultValue.strictHostKeyChecking = strictHostKeyChecking;
_resultValue.uri = uri;
_resultValue.username = username;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy