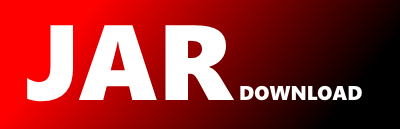
com.pulumi.azurenative.authorization.ScopeAccessReviewScheduleDefinitionById Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.authorization.ScopeAccessReviewScheduleDefinitionByIdArgs;
import com.pulumi.azurenative.authorization.outputs.AccessReviewInstanceResponse;
import com.pulumi.azurenative.authorization.outputs.AccessReviewReviewerResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Access Review Schedule Definition.
* Azure REST API version: 2021-12-01-preview. Prior API version in Azure Native 1.x: 2021-12-01-preview.
*
* ## Example Usage
* ### PutAccessReview
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.authorization.ScopeAccessReviewScheduleDefinitionById;
* import com.pulumi.azurenative.authorization.ScopeAccessReviewScheduleDefinitionByIdArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var scopeAccessReviewScheduleDefinitionById = new ScopeAccessReviewScheduleDefinitionById("scopeAccessReviewScheduleDefinitionById", ScopeAccessReviewScheduleDefinitionByIdArgs.builder()
* .scheduleDefinitionId("fa73e90b-5bf1-45fd-a182-35ce5fc0674d")
* .scope("subscriptions/fa73e90b-5bf1-45fd-a182-35ce5fc0674d")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:authorization:ScopeAccessReviewScheduleDefinitionById fa73e90b-5bf1-45fd-a182-35ce5fc0674d /{scope}/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}
* ```
*
*/
@ResourceType(type="azure-native:authorization:ScopeAccessReviewScheduleDefinitionById")
public class ScopeAccessReviewScheduleDefinitionById extends com.pulumi.resources.CustomResource {
/**
* The role assignment state eligible/active to review
*
*/
@Export(name="assignmentState", refs={String.class}, tree="[0]")
private Output assignmentState;
/**
* @return The role assignment state eligible/active to review
*
*/
public Output assignmentState() {
return this.assignmentState;
}
/**
* Flag to indicate whether auto-apply capability, to automatically change the target object access resource, is enabled. If not enabled, a user must, after the review completes, apply the access review.
*
*/
@Export(name="autoApplyDecisionsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoApplyDecisionsEnabled;
/**
* @return Flag to indicate whether auto-apply capability, to automatically change the target object access resource, is enabled. If not enabled, a user must, after the review completes, apply the access review.
*
*/
public Output> autoApplyDecisionsEnabled() {
return Codegen.optional(this.autoApplyDecisionsEnabled);
}
/**
* This is the collection of backup reviewers.
*
*/
@Export(name="backupReviewers", refs={List.class,AccessReviewReviewerResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> backupReviewers;
/**
* @return This is the collection of backup reviewers.
*
*/
public Output>> backupReviewers() {
return Codegen.optional(this.backupReviewers);
}
/**
* This specifies the behavior for the autoReview feature when an access review completes.
*
*/
@Export(name="defaultDecision", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> defaultDecision;
/**
* @return This specifies the behavior for the autoReview feature when an access review completes.
*
*/
public Output> defaultDecision() {
return Codegen.optional(this.defaultDecision);
}
/**
* Flag to indicate whether reviewers are required to provide a justification when reviewing access.
*
*/
@Export(name="defaultDecisionEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> defaultDecisionEnabled;
/**
* @return Flag to indicate whether reviewers are required to provide a justification when reviewing access.
*
*/
public Output> defaultDecisionEnabled() {
return Codegen.optional(this.defaultDecisionEnabled);
}
/**
* The description provided by the access review creator and visible to admins.
*
*/
@Export(name="descriptionForAdmins", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> descriptionForAdmins;
/**
* @return The description provided by the access review creator and visible to admins.
*
*/
public Output> descriptionForAdmins() {
return Codegen.optional(this.descriptionForAdmins);
}
/**
* The description provided by the access review creator to be shown to reviewers.
*
*/
@Export(name="descriptionForReviewers", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> descriptionForReviewers;
/**
* @return The description provided by the access review creator to be shown to reviewers.
*
*/
public Output> descriptionForReviewers() {
return Codegen.optional(this.descriptionForReviewers);
}
/**
* The display name for the schedule definition.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return The display name for the schedule definition.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
@Export(name="endDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> endDate;
/**
* @return The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
public Output> endDate() {
return Codegen.optional(this.endDate);
}
/**
* This is used to indicate the resource id(s) to exclude
*
*/
@Export(name="excludeResourceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> excludeResourceId;
/**
* @return This is used to indicate the resource id(s) to exclude
*
*/
public Output> excludeResourceId() {
return Codegen.optional(this.excludeResourceId);
}
/**
* This is used to indicate the role definition id(s) to exclude
*
*/
@Export(name="excludeRoleDefinitionId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> excludeRoleDefinitionId;
/**
* @return This is used to indicate the role definition id(s) to exclude
*
*/
public Output> excludeRoleDefinitionId() {
return Codegen.optional(this.excludeRoleDefinitionId);
}
/**
* Flag to indicate whether to expand nested memberships or not.
*
*/
@Export(name="expandNestedMemberships", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> expandNestedMemberships;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Output> expandNestedMemberships() {
return Codegen.optional(this.expandNestedMemberships);
}
/**
* Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
@Export(name="inactiveDuration", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> inactiveDuration;
/**
* @return Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
public Output> inactiveDuration() {
return Codegen.optional(this.inactiveDuration);
}
/**
* Flag to indicate whether to expand nested memberships or not.
*
*/
@Export(name="includeAccessBelowResource", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> includeAccessBelowResource;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Output> includeAccessBelowResource() {
return Codegen.optional(this.includeAccessBelowResource);
}
/**
* Flag to indicate whether to expand nested memberships or not.
*
*/
@Export(name="includeInheritedAccess", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> includeInheritedAccess;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Output> includeInheritedAccess() {
return Codegen.optional(this.includeInheritedAccess);
}
/**
* The duration in days for an instance.
*
*/
@Export(name="instanceDurationInDays", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> instanceDurationInDays;
/**
* @return The duration in days for an instance.
*
*/
public Output> instanceDurationInDays() {
return Codegen.optional(this.instanceDurationInDays);
}
/**
* This is the collection of instances returned when one does an expand on it.
*
*/
@Export(name="instances", refs={List.class,AccessReviewInstanceResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> instances;
/**
* @return This is the collection of instances returned when one does an expand on it.
*
*/
public Output>> instances() {
return Codegen.optional(this.instances);
}
/**
* The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
@Export(name="interval", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> interval;
/**
* @return The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
public Output> interval() {
return Codegen.optional(this.interval);
}
/**
* Flag to indicate whether the reviewer is required to pass justification when recording a decision.
*
*/
@Export(name="justificationRequiredOnApproval", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> justificationRequiredOnApproval;
/**
* @return Flag to indicate whether the reviewer is required to pass justification when recording a decision.
*
*/
public Output> justificationRequiredOnApproval() {
return Codegen.optional(this.justificationRequiredOnApproval);
}
/**
* Flag to indicate whether sending mails to reviewers and the review creator is enabled.
*
*/
@Export(name="mailNotificationsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> mailNotificationsEnabled;
/**
* @return Flag to indicate whether sending mails to reviewers and the review creator is enabled.
*
*/
public Output> mailNotificationsEnabled() {
return Codegen.optional(this.mailNotificationsEnabled);
}
/**
* The access review schedule definition unique id.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The access review schedule definition unique id.
*
*/
public Output name() {
return this.name;
}
/**
* The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
@Export(name="numberOfOccurrences", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> numberOfOccurrences;
/**
* @return The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
public Output> numberOfOccurrences() {
return Codegen.optional(this.numberOfOccurrences);
}
/**
* The identity id
*
*/
@Export(name="principalId", refs={String.class}, tree="[0]")
private Output principalId;
/**
* @return The identity id
*
*/
public Output principalId() {
return this.principalId;
}
/**
* The identity display name
*
*/
@Export(name="principalName", refs={String.class}, tree="[0]")
private Output principalName;
/**
* @return The identity display name
*
*/
public Output principalName() {
return this.principalName;
}
/**
* The identity type user/servicePrincipal to review
*
*/
@Export(name="principalType", refs={String.class}, tree="[0]")
private Output principalType;
/**
* @return The identity type user/servicePrincipal to review
*
*/
public Output principalType() {
return this.principalType;
}
/**
* Recommendations for access reviews are calculated by looking back at 30 days of data(w.r.t the start date of the review) by default. However, in some scenarios, customers want to change how far back to look at and want to configure 60 days, 90 days, etc. instead. This setting allows customers to configure this duration. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
@Export(name="recommendationLookBackDuration", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> recommendationLookBackDuration;
/**
* @return Recommendations for access reviews are calculated by looking back at 30 days of data(w.r.t the start date of the review) by default. However, in some scenarios, customers want to change how far back to look at and want to configure 60 days, 90 days, etc. instead. This setting allows customers to configure this duration. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
public Output> recommendationLookBackDuration() {
return Codegen.optional(this.recommendationLookBackDuration);
}
/**
* Flag to indicate whether showing recommendations to reviewers is enabled.
*
*/
@Export(name="recommendationsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> recommendationsEnabled;
/**
* @return Flag to indicate whether showing recommendations to reviewers is enabled.
*
*/
public Output> recommendationsEnabled() {
return Codegen.optional(this.recommendationsEnabled);
}
/**
* Flag to indicate whether sending reminder emails to reviewers are enabled.
*
*/
@Export(name="reminderNotificationsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> reminderNotificationsEnabled;
/**
* @return Flag to indicate whether sending reminder emails to reviewers are enabled.
*
*/
public Output> reminderNotificationsEnabled() {
return Codegen.optional(this.reminderNotificationsEnabled);
}
/**
* ResourceId in which this review is getting created
*
*/
@Export(name="resourceId", refs={String.class}, tree="[0]")
private Output resourceId;
/**
* @return ResourceId in which this review is getting created
*
*/
public Output resourceId() {
return this.resourceId;
}
/**
* This is the collection of reviewers.
*
*/
@Export(name="reviewers", refs={List.class,AccessReviewReviewerResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> reviewers;
/**
* @return This is the collection of reviewers.
*
*/
public Output>> reviewers() {
return Codegen.optional(this.reviewers);
}
/**
* This field specifies the type of reviewers for a review. Usually for a review, reviewers are explicitly assigned. However, in some cases, the reviewers may not be assigned and instead be chosen dynamically. For example managers review or self review.
*
*/
@Export(name="reviewersType", refs={String.class}, tree="[0]")
private Output reviewersType;
/**
* @return This field specifies the type of reviewers for a review. Usually for a review, reviewers are explicitly assigned. However, in some cases, the reviewers may not be assigned and instead be chosen dynamically. For example managers review or self review.
*
*/
public Output reviewersType() {
return this.reviewersType;
}
/**
* This is used to indicate the role being reviewed
*
*/
@Export(name="roleDefinitionId", refs={String.class}, tree="[0]")
private Output roleDefinitionId;
/**
* @return This is used to indicate the role being reviewed
*
*/
public Output roleDefinitionId() {
return this.roleDefinitionId;
}
/**
* The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
@Export(name="startDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> startDate;
/**
* @return The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
public Output> startDate() {
return Codegen.optional(this.startDate);
}
/**
* This read-only field specifies the status of an accessReview.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return This read-only field specifies the status of an accessReview.
*
*/
public Output status() {
return this.status;
}
/**
* The resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The resource type.
*
*/
public Output type() {
return this.type;
}
/**
* The user principal name(if valid)
*
*/
@Export(name="userPrincipalName", refs={String.class}, tree="[0]")
private Output userPrincipalName;
/**
* @return The user principal name(if valid)
*
*/
public Output userPrincipalName() {
return this.userPrincipalName;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ScopeAccessReviewScheduleDefinitionById(java.lang.String name) {
this(name, ScopeAccessReviewScheduleDefinitionByIdArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ScopeAccessReviewScheduleDefinitionById(java.lang.String name, ScopeAccessReviewScheduleDefinitionByIdArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ScopeAccessReviewScheduleDefinitionById(java.lang.String name, ScopeAccessReviewScheduleDefinitionByIdArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:ScopeAccessReviewScheduleDefinitionById", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ScopeAccessReviewScheduleDefinitionById(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:ScopeAccessReviewScheduleDefinitionById", name, null, makeResourceOptions(options, id), false);
}
private static ScopeAccessReviewScheduleDefinitionByIdArgs makeArgs(ScopeAccessReviewScheduleDefinitionByIdArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ScopeAccessReviewScheduleDefinitionByIdArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:authorization/v20211201preview:ScopeAccessReviewScheduleDefinitionById").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ScopeAccessReviewScheduleDefinitionById get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ScopeAccessReviewScheduleDefinitionById(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy