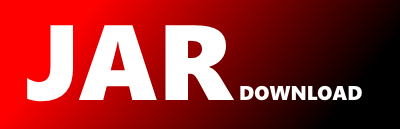
com.pulumi.azurenative.authorization.outputs.GetScopeAccessReviewScheduleDefinitionByIdResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization.outputs;
import com.pulumi.azurenative.authorization.outputs.AccessReviewInstanceResponse;
import com.pulumi.azurenative.authorization.outputs.AccessReviewReviewerResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetScopeAccessReviewScheduleDefinitionByIdResult {
/**
* @return The role assignment state eligible/active to review
*
*/
private String assignmentState;
/**
* @return Flag to indicate whether auto-apply capability, to automatically change the target object access resource, is enabled. If not enabled, a user must, after the review completes, apply the access review.
*
*/
private @Nullable Boolean autoApplyDecisionsEnabled;
/**
* @return This is the collection of backup reviewers.
*
*/
private @Nullable List backupReviewers;
/**
* @return This specifies the behavior for the autoReview feature when an access review completes.
*
*/
private @Nullable String defaultDecision;
/**
* @return Flag to indicate whether reviewers are required to provide a justification when reviewing access.
*
*/
private @Nullable Boolean defaultDecisionEnabled;
/**
* @return The description provided by the access review creator and visible to admins.
*
*/
private @Nullable String descriptionForAdmins;
/**
* @return The description provided by the access review creator to be shown to reviewers.
*
*/
private @Nullable String descriptionForReviewers;
/**
* @return The display name for the schedule definition.
*
*/
private @Nullable String displayName;
/**
* @return The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
private @Nullable String endDate;
/**
* @return This is used to indicate the resource id(s) to exclude
*
*/
private @Nullable String excludeResourceId;
/**
* @return This is used to indicate the role definition id(s) to exclude
*
*/
private @Nullable String excludeRoleDefinitionId;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
private @Nullable Boolean expandNestedMemberships;
/**
* @return The access review schedule definition id.
*
*/
private String id;
/**
* @return Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
private @Nullable String inactiveDuration;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
private @Nullable Boolean includeAccessBelowResource;
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
private @Nullable Boolean includeInheritedAccess;
/**
* @return The duration in days for an instance.
*
*/
private @Nullable Integer instanceDurationInDays;
/**
* @return This is the collection of instances returned when one does an expand on it.
*
*/
private @Nullable List instances;
/**
* @return The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
private @Nullable Integer interval;
/**
* @return Flag to indicate whether the reviewer is required to pass justification when recording a decision.
*
*/
private @Nullable Boolean justificationRequiredOnApproval;
/**
* @return Flag to indicate whether sending mails to reviewers and the review creator is enabled.
*
*/
private @Nullable Boolean mailNotificationsEnabled;
/**
* @return The access review schedule definition unique id.
*
*/
private String name;
/**
* @return The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
private @Nullable Integer numberOfOccurrences;
/**
* @return The identity id
*
*/
private String principalId;
/**
* @return The identity display name
*
*/
private String principalName;
/**
* @return The identity type user/servicePrincipal to review
*
*/
private String principalType;
/**
* @return Recommendations for access reviews are calculated by looking back at 30 days of data(w.r.t the start date of the review) by default. However, in some scenarios, customers want to change how far back to look at and want to configure 60 days, 90 days, etc. instead. This setting allows customers to configure this duration. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
private @Nullable String recommendationLookBackDuration;
/**
* @return Flag to indicate whether showing recommendations to reviewers is enabled.
*
*/
private @Nullable Boolean recommendationsEnabled;
/**
* @return Flag to indicate whether sending reminder emails to reviewers are enabled.
*
*/
private @Nullable Boolean reminderNotificationsEnabled;
/**
* @return ResourceId in which this review is getting created
*
*/
private String resourceId;
/**
* @return This is the collection of reviewers.
*
*/
private @Nullable List reviewers;
/**
* @return This field specifies the type of reviewers for a review. Usually for a review, reviewers are explicitly assigned. However, in some cases, the reviewers may not be assigned and instead be chosen dynamically. For example managers review or self review.
*
*/
private String reviewersType;
/**
* @return This is used to indicate the role being reviewed
*
*/
private String roleDefinitionId;
/**
* @return The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
private @Nullable String startDate;
/**
* @return This read-only field specifies the status of an accessReview.
*
*/
private String status;
/**
* @return The resource type.
*
*/
private String type;
/**
* @return The user principal name(if valid)
*
*/
private String userPrincipalName;
private GetScopeAccessReviewScheduleDefinitionByIdResult() {}
/**
* @return The role assignment state eligible/active to review
*
*/
public String assignmentState() {
return this.assignmentState;
}
/**
* @return Flag to indicate whether auto-apply capability, to automatically change the target object access resource, is enabled. If not enabled, a user must, after the review completes, apply the access review.
*
*/
public Optional autoApplyDecisionsEnabled() {
return Optional.ofNullable(this.autoApplyDecisionsEnabled);
}
/**
* @return This is the collection of backup reviewers.
*
*/
public List backupReviewers() {
return this.backupReviewers == null ? List.of() : this.backupReviewers;
}
/**
* @return This specifies the behavior for the autoReview feature when an access review completes.
*
*/
public Optional defaultDecision() {
return Optional.ofNullable(this.defaultDecision);
}
/**
* @return Flag to indicate whether reviewers are required to provide a justification when reviewing access.
*
*/
public Optional defaultDecisionEnabled() {
return Optional.ofNullable(this.defaultDecisionEnabled);
}
/**
* @return The description provided by the access review creator and visible to admins.
*
*/
public Optional descriptionForAdmins() {
return Optional.ofNullable(this.descriptionForAdmins);
}
/**
* @return The description provided by the access review creator to be shown to reviewers.
*
*/
public Optional descriptionForReviewers() {
return Optional.ofNullable(this.descriptionForReviewers);
}
/**
* @return The display name for the schedule definition.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return The DateTime when the review is scheduled to end. Required if type is endDate
*
*/
public Optional endDate() {
return Optional.ofNullable(this.endDate);
}
/**
* @return This is used to indicate the resource id(s) to exclude
*
*/
public Optional excludeResourceId() {
return Optional.ofNullable(this.excludeResourceId);
}
/**
* @return This is used to indicate the role definition id(s) to exclude
*
*/
public Optional excludeRoleDefinitionId() {
return Optional.ofNullable(this.excludeRoleDefinitionId);
}
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Optional expandNestedMemberships() {
return Optional.ofNullable(this.expandNestedMemberships);
}
/**
* @return The access review schedule definition id.
*
*/
public String id() {
return this.id;
}
/**
* @return Duration users are inactive for. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
public Optional inactiveDuration() {
return Optional.ofNullable(this.inactiveDuration);
}
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Optional includeAccessBelowResource() {
return Optional.ofNullable(this.includeAccessBelowResource);
}
/**
* @return Flag to indicate whether to expand nested memberships or not.
*
*/
public Optional includeInheritedAccess() {
return Optional.ofNullable(this.includeInheritedAccess);
}
/**
* @return The duration in days for an instance.
*
*/
public Optional instanceDurationInDays() {
return Optional.ofNullable(this.instanceDurationInDays);
}
/**
* @return This is the collection of instances returned when one does an expand on it.
*
*/
public List instances() {
return this.instances == null ? List.of() : this.instances;
}
/**
* @return The interval for recurrence. For a quarterly review, the interval is 3 for type : absoluteMonthly.
*
*/
public Optional interval() {
return Optional.ofNullable(this.interval);
}
/**
* @return Flag to indicate whether the reviewer is required to pass justification when recording a decision.
*
*/
public Optional justificationRequiredOnApproval() {
return Optional.ofNullable(this.justificationRequiredOnApproval);
}
/**
* @return Flag to indicate whether sending mails to reviewers and the review creator is enabled.
*
*/
public Optional mailNotificationsEnabled() {
return Optional.ofNullable(this.mailNotificationsEnabled);
}
/**
* @return The access review schedule definition unique id.
*
*/
public String name() {
return this.name;
}
/**
* @return The number of times to repeat the access review. Required and must be positive if type is numbered.
*
*/
public Optional numberOfOccurrences() {
return Optional.ofNullable(this.numberOfOccurrences);
}
/**
* @return The identity id
*
*/
public String principalId() {
return this.principalId;
}
/**
* @return The identity display name
*
*/
public String principalName() {
return this.principalName;
}
/**
* @return The identity type user/servicePrincipal to review
*
*/
public String principalType() {
return this.principalType;
}
/**
* @return Recommendations for access reviews are calculated by looking back at 30 days of data(w.r.t the start date of the review) by default. However, in some scenarios, customers want to change how far back to look at and want to configure 60 days, 90 days, etc. instead. This setting allows customers to configure this duration. The value should be in ISO 8601 format (http://en.wikipedia.org/wiki/ISO_8601#Durations).This code can be used to convert TimeSpan to a valid interval string: XmlConvert.ToString(new TimeSpan(hours, minutes, seconds))
*
*/
public Optional recommendationLookBackDuration() {
return Optional.ofNullable(this.recommendationLookBackDuration);
}
/**
* @return Flag to indicate whether showing recommendations to reviewers is enabled.
*
*/
public Optional recommendationsEnabled() {
return Optional.ofNullable(this.recommendationsEnabled);
}
/**
* @return Flag to indicate whether sending reminder emails to reviewers are enabled.
*
*/
public Optional reminderNotificationsEnabled() {
return Optional.ofNullable(this.reminderNotificationsEnabled);
}
/**
* @return ResourceId in which this review is getting created
*
*/
public String resourceId() {
return this.resourceId;
}
/**
* @return This is the collection of reviewers.
*
*/
public List reviewers() {
return this.reviewers == null ? List.of() : this.reviewers;
}
/**
* @return This field specifies the type of reviewers for a review. Usually for a review, reviewers are explicitly assigned. However, in some cases, the reviewers may not be assigned and instead be chosen dynamically. For example managers review or self review.
*
*/
public String reviewersType() {
return this.reviewersType;
}
/**
* @return This is used to indicate the role being reviewed
*
*/
public String roleDefinitionId() {
return this.roleDefinitionId;
}
/**
* @return The DateTime when the review is scheduled to be start. This could be a date in the future. Required on create.
*
*/
public Optional startDate() {
return Optional.ofNullable(this.startDate);
}
/**
* @return This read-only field specifies the status of an accessReview.
*
*/
public String status() {
return this.status;
}
/**
* @return The resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The user principal name(if valid)
*
*/
public String userPrincipalName() {
return this.userPrincipalName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScopeAccessReviewScheduleDefinitionByIdResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String assignmentState;
private @Nullable Boolean autoApplyDecisionsEnabled;
private @Nullable List backupReviewers;
private @Nullable String defaultDecision;
private @Nullable Boolean defaultDecisionEnabled;
private @Nullable String descriptionForAdmins;
private @Nullable String descriptionForReviewers;
private @Nullable String displayName;
private @Nullable String endDate;
private @Nullable String excludeResourceId;
private @Nullable String excludeRoleDefinitionId;
private @Nullable Boolean expandNestedMemberships;
private String id;
private @Nullable String inactiveDuration;
private @Nullable Boolean includeAccessBelowResource;
private @Nullable Boolean includeInheritedAccess;
private @Nullable Integer instanceDurationInDays;
private @Nullable List instances;
private @Nullable Integer interval;
private @Nullable Boolean justificationRequiredOnApproval;
private @Nullable Boolean mailNotificationsEnabled;
private String name;
private @Nullable Integer numberOfOccurrences;
private String principalId;
private String principalName;
private String principalType;
private @Nullable String recommendationLookBackDuration;
private @Nullable Boolean recommendationsEnabled;
private @Nullable Boolean reminderNotificationsEnabled;
private String resourceId;
private @Nullable List reviewers;
private String reviewersType;
private String roleDefinitionId;
private @Nullable String startDate;
private String status;
private String type;
private String userPrincipalName;
public Builder() {}
public Builder(GetScopeAccessReviewScheduleDefinitionByIdResult defaults) {
Objects.requireNonNull(defaults);
this.assignmentState = defaults.assignmentState;
this.autoApplyDecisionsEnabled = defaults.autoApplyDecisionsEnabled;
this.backupReviewers = defaults.backupReviewers;
this.defaultDecision = defaults.defaultDecision;
this.defaultDecisionEnabled = defaults.defaultDecisionEnabled;
this.descriptionForAdmins = defaults.descriptionForAdmins;
this.descriptionForReviewers = defaults.descriptionForReviewers;
this.displayName = defaults.displayName;
this.endDate = defaults.endDate;
this.excludeResourceId = defaults.excludeResourceId;
this.excludeRoleDefinitionId = defaults.excludeRoleDefinitionId;
this.expandNestedMemberships = defaults.expandNestedMemberships;
this.id = defaults.id;
this.inactiveDuration = defaults.inactiveDuration;
this.includeAccessBelowResource = defaults.includeAccessBelowResource;
this.includeInheritedAccess = defaults.includeInheritedAccess;
this.instanceDurationInDays = defaults.instanceDurationInDays;
this.instances = defaults.instances;
this.interval = defaults.interval;
this.justificationRequiredOnApproval = defaults.justificationRequiredOnApproval;
this.mailNotificationsEnabled = defaults.mailNotificationsEnabled;
this.name = defaults.name;
this.numberOfOccurrences = defaults.numberOfOccurrences;
this.principalId = defaults.principalId;
this.principalName = defaults.principalName;
this.principalType = defaults.principalType;
this.recommendationLookBackDuration = defaults.recommendationLookBackDuration;
this.recommendationsEnabled = defaults.recommendationsEnabled;
this.reminderNotificationsEnabled = defaults.reminderNotificationsEnabled;
this.resourceId = defaults.resourceId;
this.reviewers = defaults.reviewers;
this.reviewersType = defaults.reviewersType;
this.roleDefinitionId = defaults.roleDefinitionId;
this.startDate = defaults.startDate;
this.status = defaults.status;
this.type = defaults.type;
this.userPrincipalName = defaults.userPrincipalName;
}
@CustomType.Setter
public Builder assignmentState(String assignmentState) {
if (assignmentState == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "assignmentState");
}
this.assignmentState = assignmentState;
return this;
}
@CustomType.Setter
public Builder autoApplyDecisionsEnabled(@Nullable Boolean autoApplyDecisionsEnabled) {
this.autoApplyDecisionsEnabled = autoApplyDecisionsEnabled;
return this;
}
@CustomType.Setter
public Builder backupReviewers(@Nullable List backupReviewers) {
this.backupReviewers = backupReviewers;
return this;
}
public Builder backupReviewers(AccessReviewReviewerResponse... backupReviewers) {
return backupReviewers(List.of(backupReviewers));
}
@CustomType.Setter
public Builder defaultDecision(@Nullable String defaultDecision) {
this.defaultDecision = defaultDecision;
return this;
}
@CustomType.Setter
public Builder defaultDecisionEnabled(@Nullable Boolean defaultDecisionEnabled) {
this.defaultDecisionEnabled = defaultDecisionEnabled;
return this;
}
@CustomType.Setter
public Builder descriptionForAdmins(@Nullable String descriptionForAdmins) {
this.descriptionForAdmins = descriptionForAdmins;
return this;
}
@CustomType.Setter
public Builder descriptionForReviewers(@Nullable String descriptionForReviewers) {
this.descriptionForReviewers = descriptionForReviewers;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder endDate(@Nullable String endDate) {
this.endDate = endDate;
return this;
}
@CustomType.Setter
public Builder excludeResourceId(@Nullable String excludeResourceId) {
this.excludeResourceId = excludeResourceId;
return this;
}
@CustomType.Setter
public Builder excludeRoleDefinitionId(@Nullable String excludeRoleDefinitionId) {
this.excludeRoleDefinitionId = excludeRoleDefinitionId;
return this;
}
@CustomType.Setter
public Builder expandNestedMemberships(@Nullable Boolean expandNestedMemberships) {
this.expandNestedMemberships = expandNestedMemberships;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder inactiveDuration(@Nullable String inactiveDuration) {
this.inactiveDuration = inactiveDuration;
return this;
}
@CustomType.Setter
public Builder includeAccessBelowResource(@Nullable Boolean includeAccessBelowResource) {
this.includeAccessBelowResource = includeAccessBelowResource;
return this;
}
@CustomType.Setter
public Builder includeInheritedAccess(@Nullable Boolean includeInheritedAccess) {
this.includeInheritedAccess = includeInheritedAccess;
return this;
}
@CustomType.Setter
public Builder instanceDurationInDays(@Nullable Integer instanceDurationInDays) {
this.instanceDurationInDays = instanceDurationInDays;
return this;
}
@CustomType.Setter
public Builder instances(@Nullable List instances) {
this.instances = instances;
return this;
}
public Builder instances(AccessReviewInstanceResponse... instances) {
return instances(List.of(instances));
}
@CustomType.Setter
public Builder interval(@Nullable Integer interval) {
this.interval = interval;
return this;
}
@CustomType.Setter
public Builder justificationRequiredOnApproval(@Nullable Boolean justificationRequiredOnApproval) {
this.justificationRequiredOnApproval = justificationRequiredOnApproval;
return this;
}
@CustomType.Setter
public Builder mailNotificationsEnabled(@Nullable Boolean mailNotificationsEnabled) {
this.mailNotificationsEnabled = mailNotificationsEnabled;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder numberOfOccurrences(@Nullable Integer numberOfOccurrences) {
this.numberOfOccurrences = numberOfOccurrences;
return this;
}
@CustomType.Setter
public Builder principalId(String principalId) {
if (principalId == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "principalId");
}
this.principalId = principalId;
return this;
}
@CustomType.Setter
public Builder principalName(String principalName) {
if (principalName == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "principalName");
}
this.principalName = principalName;
return this;
}
@CustomType.Setter
public Builder principalType(String principalType) {
if (principalType == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "principalType");
}
this.principalType = principalType;
return this;
}
@CustomType.Setter
public Builder recommendationLookBackDuration(@Nullable String recommendationLookBackDuration) {
this.recommendationLookBackDuration = recommendationLookBackDuration;
return this;
}
@CustomType.Setter
public Builder recommendationsEnabled(@Nullable Boolean recommendationsEnabled) {
this.recommendationsEnabled = recommendationsEnabled;
return this;
}
@CustomType.Setter
public Builder reminderNotificationsEnabled(@Nullable Boolean reminderNotificationsEnabled) {
this.reminderNotificationsEnabled = reminderNotificationsEnabled;
return this;
}
@CustomType.Setter
public Builder resourceId(String resourceId) {
if (resourceId == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "resourceId");
}
this.resourceId = resourceId;
return this;
}
@CustomType.Setter
public Builder reviewers(@Nullable List reviewers) {
this.reviewers = reviewers;
return this;
}
public Builder reviewers(AccessReviewReviewerResponse... reviewers) {
return reviewers(List.of(reviewers));
}
@CustomType.Setter
public Builder reviewersType(String reviewersType) {
if (reviewersType == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "reviewersType");
}
this.reviewersType = reviewersType;
return this;
}
@CustomType.Setter
public Builder roleDefinitionId(String roleDefinitionId) {
if (roleDefinitionId == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "roleDefinitionId");
}
this.roleDefinitionId = roleDefinitionId;
return this;
}
@CustomType.Setter
public Builder startDate(@Nullable String startDate) {
this.startDate = startDate;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userPrincipalName(String userPrincipalName) {
if (userPrincipalName == null) {
throw new MissingRequiredPropertyException("GetScopeAccessReviewScheduleDefinitionByIdResult", "userPrincipalName");
}
this.userPrincipalName = userPrincipalName;
return this;
}
public GetScopeAccessReviewScheduleDefinitionByIdResult build() {
final var _resultValue = new GetScopeAccessReviewScheduleDefinitionByIdResult();
_resultValue.assignmentState = assignmentState;
_resultValue.autoApplyDecisionsEnabled = autoApplyDecisionsEnabled;
_resultValue.backupReviewers = backupReviewers;
_resultValue.defaultDecision = defaultDecision;
_resultValue.defaultDecisionEnabled = defaultDecisionEnabled;
_resultValue.descriptionForAdmins = descriptionForAdmins;
_resultValue.descriptionForReviewers = descriptionForReviewers;
_resultValue.displayName = displayName;
_resultValue.endDate = endDate;
_resultValue.excludeResourceId = excludeResourceId;
_resultValue.excludeRoleDefinitionId = excludeRoleDefinitionId;
_resultValue.expandNestedMemberships = expandNestedMemberships;
_resultValue.id = id;
_resultValue.inactiveDuration = inactiveDuration;
_resultValue.includeAccessBelowResource = includeAccessBelowResource;
_resultValue.includeInheritedAccess = includeInheritedAccess;
_resultValue.instanceDurationInDays = instanceDurationInDays;
_resultValue.instances = instances;
_resultValue.interval = interval;
_resultValue.justificationRequiredOnApproval = justificationRequiredOnApproval;
_resultValue.mailNotificationsEnabled = mailNotificationsEnabled;
_resultValue.name = name;
_resultValue.numberOfOccurrences = numberOfOccurrences;
_resultValue.principalId = principalId;
_resultValue.principalName = principalName;
_resultValue.principalType = principalType;
_resultValue.recommendationLookBackDuration = recommendationLookBackDuration;
_resultValue.recommendationsEnabled = recommendationsEnabled;
_resultValue.reminderNotificationsEnabled = reminderNotificationsEnabled;
_resultValue.resourceId = resourceId;
_resultValue.reviewers = reviewers;
_resultValue.reviewersType = reviewersType;
_resultValue.roleDefinitionId = roleDefinitionId;
_resultValue.startDate = startDate;
_resultValue.status = status;
_resultValue.type = type;
_resultValue.userPrincipalName = userPrincipalName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy