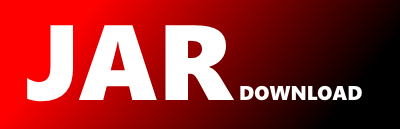
com.pulumi.azurenative.authorization.outputs.PermissionResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class PermissionResponse {
/**
* @return Allowed actions.
*
*/
private @Nullable List actions;
/**
* @return The conditions on the role definition. This limits the resources it can be assigned to. e.g.: {@literal @}Resource[Microsoft.Storage/storageAccounts/blobServices/containers:ContainerName] StringEqualsIgnoreCase 'foo_storage_container'
*
*/
private String condition;
/**
* @return Version of the condition. Currently the only accepted value is '2.0'
*
*/
private String conditionVersion;
/**
* @return Allowed Data actions.
*
*/
private @Nullable List dataActions;
/**
* @return Denied actions.
*
*/
private @Nullable List notActions;
/**
* @return Denied Data actions.
*
*/
private @Nullable List notDataActions;
private PermissionResponse() {}
/**
* @return Allowed actions.
*
*/
public List actions() {
return this.actions == null ? List.of() : this.actions;
}
/**
* @return The conditions on the role definition. This limits the resources it can be assigned to. e.g.: {@literal @}Resource[Microsoft.Storage/storageAccounts/blobServices/containers:ContainerName] StringEqualsIgnoreCase 'foo_storage_container'
*
*/
public String condition() {
return this.condition;
}
/**
* @return Version of the condition. Currently the only accepted value is '2.0'
*
*/
public String conditionVersion() {
return this.conditionVersion;
}
/**
* @return Allowed Data actions.
*
*/
public List dataActions() {
return this.dataActions == null ? List.of() : this.dataActions;
}
/**
* @return Denied actions.
*
*/
public List notActions() {
return this.notActions == null ? List.of() : this.notActions;
}
/**
* @return Denied Data actions.
*
*/
public List notDataActions() {
return this.notDataActions == null ? List.of() : this.notDataActions;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PermissionResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List actions;
private String condition;
private String conditionVersion;
private @Nullable List dataActions;
private @Nullable List notActions;
private @Nullable List notDataActions;
public Builder() {}
public Builder(PermissionResponse defaults) {
Objects.requireNonNull(defaults);
this.actions = defaults.actions;
this.condition = defaults.condition;
this.conditionVersion = defaults.conditionVersion;
this.dataActions = defaults.dataActions;
this.notActions = defaults.notActions;
this.notDataActions = defaults.notDataActions;
}
@CustomType.Setter
public Builder actions(@Nullable List actions) {
this.actions = actions;
return this;
}
public Builder actions(String... actions) {
return actions(List.of(actions));
}
@CustomType.Setter
public Builder condition(String condition) {
if (condition == null) {
throw new MissingRequiredPropertyException("PermissionResponse", "condition");
}
this.condition = condition;
return this;
}
@CustomType.Setter
public Builder conditionVersion(String conditionVersion) {
if (conditionVersion == null) {
throw new MissingRequiredPropertyException("PermissionResponse", "conditionVersion");
}
this.conditionVersion = conditionVersion;
return this;
}
@CustomType.Setter
public Builder dataActions(@Nullable List dataActions) {
this.dataActions = dataActions;
return this;
}
public Builder dataActions(String... dataActions) {
return dataActions(List.of(dataActions));
}
@CustomType.Setter
public Builder notActions(@Nullable List notActions) {
this.notActions = notActions;
return this;
}
public Builder notActions(String... notActions) {
return notActions(List.of(notActions));
}
@CustomType.Setter
public Builder notDataActions(@Nullable List notDataActions) {
this.notDataActions = notDataActions;
return this;
}
public Builder notDataActions(String... notDataActions) {
return notDataActions(List.of(notDataActions));
}
public PermissionResponse build() {
final var _resultValue = new PermissionResponse();
_resultValue.actions = actions;
_resultValue.condition = condition;
_resultValue.conditionVersion = conditionVersion;
_resultValue.dataActions = dataActions;
_resultValue.notActions = notActions;
_resultValue.notDataActions = notDataActions;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy