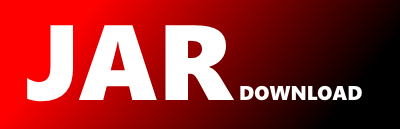
com.pulumi.azurenative.azurefleet.inputs.ComputeProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurefleet.inputs;
import com.pulumi.azurenative.azurefleet.inputs.BaseVirtualMachineProfileArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Compute Profile to use for running user's workloads.
*
*/
public final class ComputeProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final ComputeProfileArgs Empty = new ComputeProfileArgs();
/**
* Base Virtual Machine Profile Properties to be specified according to "specification/compute/resource-manager/Microsoft.Compute/ComputeRP/stable/{computeApiVersion}/virtualMachineScaleSet.json#/definitions/VirtualMachineScaleSetVMProfile"
*
*/
@Import(name="baseVirtualMachineProfile", required=true)
private Output baseVirtualMachineProfile;
/**
* @return Base Virtual Machine Profile Properties to be specified according to "specification/compute/resource-manager/Microsoft.Compute/ComputeRP/stable/{computeApiVersion}/virtualMachineScaleSet.json#/definitions/VirtualMachineScaleSetVMProfile"
*
*/
public Output baseVirtualMachineProfile() {
return this.baseVirtualMachineProfile;
}
/**
* Specifies the Microsoft.Compute API version to use when creating underlying Virtual Machine scale sets and Virtual Machines.
* The default value will be the latest supported computeApiVersion by Compute Fleet.
*
*/
@Import(name="computeApiVersion")
private @Nullable Output computeApiVersion;
/**
* @return Specifies the Microsoft.Compute API version to use when creating underlying Virtual Machine scale sets and Virtual Machines.
* The default value will be the latest supported computeApiVersion by Compute Fleet.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy