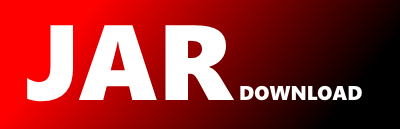
com.pulumi.azurenative.azurefleet.outputs.BaseVirtualMachineProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurefleet.outputs;
import com.pulumi.azurenative.azurefleet.outputs.ApplicationProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.CapacityReservationProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.DiagnosticsProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.ScheduledEventsProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.SecurityPostureReferenceResponse;
import com.pulumi.azurenative.azurefleet.outputs.SecurityProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.ServiceArtifactReferenceResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetExtensionProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetHardwareProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetNetworkProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetOSProfileResponse;
import com.pulumi.azurenative.azurefleet.outputs.VirtualMachineScaleSetStorageProfileResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BaseVirtualMachineProfileResponse {
/**
* @return Specifies the gallery applications that should be made available to the VM/VMSS
*
*/
private @Nullable ApplicationProfileResponse applicationProfile;
/**
* @return Specifies the capacity reservation related details of a scale set. Minimum
* api-version: 2021-04-01.
*
*/
private @Nullable CapacityReservationProfileResponse capacityReservation;
/**
* @return Specifies the boot diagnostic settings state.
*
*/
private @Nullable DiagnosticsProfileResponse diagnosticsProfile;
/**
* @return Specifies a collection of settings for extensions installed on virtual machines
* in the scale set.
*
*/
private @Nullable VirtualMachineScaleSetExtensionProfileResponse extensionProfile;
/**
* @return Specifies the hardware profile related details of a scale set. Minimum
* api-version: 2021-11-01.
*
*/
private @Nullable VirtualMachineScaleSetHardwareProfileResponse hardwareProfile;
/**
* @return Specifies that the image or disk that is being used was licensed on-premises.
* <br><br> Possible values for Windows Server operating system are: <br><br>
* Windows_Client <br><br> Windows_Server <br><br> Possible values for Linux
* Server operating system are: <br><br> RHEL_BYOS (for RHEL) <br><br> SLES_BYOS
* (for SUSE) <br><br> For more information, see [Azure Hybrid Use Benefit for
* Windows
* Server](https://docs.microsoft.com/azure/virtual-machines/windows/hybrid-use-benefit-licensing)
* <br><br> [Azure Hybrid Use Benefit for Linux
* Server](https://docs.microsoft.com/azure/virtual-machines/linux/azure-hybrid-benefit-linux)
* <br><br> Minimum api-version: 2015-06-15
*
*/
private @Nullable String licenseType;
/**
* @return Specifies properties of the network interfaces of the virtual machines in the
* scale set.
*
*/
private @Nullable VirtualMachineScaleSetNetworkProfileResponse networkProfile;
/**
* @return Specifies the operating system settings for the virtual machines in the scale
* set.
*
*/
private @Nullable VirtualMachineScaleSetOSProfileResponse osProfile;
/**
* @return Specifies Scheduled Event related configurations.
*
*/
private @Nullable ScheduledEventsProfileResponse scheduledEventsProfile;
/**
* @return Specifies the security posture to be used for all virtual machines in the scale
* set. Minimum api-version: 2023-03-01
*
*/
private @Nullable SecurityPostureReferenceResponse securityPostureReference;
/**
* @return Specifies the Security related profile settings for the virtual machines in the
* scale set.
*
*/
private @Nullable SecurityProfileResponse securityProfile;
/**
* @return Specifies the service artifact reference id used to set same image version for
* all virtual machines in the scale set when using 'latest' image version.
* Minimum api-version: 2022-11-01
*
*/
private @Nullable ServiceArtifactReferenceResponse serviceArtifactReference;
/**
* @return Specifies the storage settings for the virtual machine disks.
*
*/
private @Nullable VirtualMachineScaleSetStorageProfileResponse storageProfile;
/**
* @return Specifies the time in which this VM profile for the Virtual Machine Scale Set
* was created. Minimum API version for this property is 2023-09-01. This value
* will be added to VMSS Flex VM tags when creating/updating the VMSS VM Profile
* with minimum api-version 2023-09-01. Examples: "2024-07-01T00:00:01.1234567+00:00"
*
*/
private String timeCreated;
/**
* @return UserData for the virtual machines in the scale set, which must be base-64
* encoded. Customer should not pass any secrets in here. Minimum api-version:
* 2021-03-01.
*
*/
private @Nullable String userData;
private BaseVirtualMachineProfileResponse() {}
/**
* @return Specifies the gallery applications that should be made available to the VM/VMSS
*
*/
public Optional applicationProfile() {
return Optional.ofNullable(this.applicationProfile);
}
/**
* @return Specifies the capacity reservation related details of a scale set. Minimum
* api-version: 2021-04-01.
*
*/
public Optional capacityReservation() {
return Optional.ofNullable(this.capacityReservation);
}
/**
* @return Specifies the boot diagnostic settings state.
*
*/
public Optional diagnosticsProfile() {
return Optional.ofNullable(this.diagnosticsProfile);
}
/**
* @return Specifies a collection of settings for extensions installed on virtual machines
* in the scale set.
*
*/
public Optional extensionProfile() {
return Optional.ofNullable(this.extensionProfile);
}
/**
* @return Specifies the hardware profile related details of a scale set. Minimum
* api-version: 2021-11-01.
*
*/
public Optional hardwareProfile() {
return Optional.ofNullable(this.hardwareProfile);
}
/**
* @return Specifies that the image or disk that is being used was licensed on-premises.
* <br><br> Possible values for Windows Server operating system are: <br><br>
* Windows_Client <br><br> Windows_Server <br><br> Possible values for Linux
* Server operating system are: <br><br> RHEL_BYOS (for RHEL) <br><br> SLES_BYOS
* (for SUSE) <br><br> For more information, see [Azure Hybrid Use Benefit for
* Windows
* Server](https://docs.microsoft.com/azure/virtual-machines/windows/hybrid-use-benefit-licensing)
* <br><br> [Azure Hybrid Use Benefit for Linux
* Server](https://docs.microsoft.com/azure/virtual-machines/linux/azure-hybrid-benefit-linux)
* <br><br> Minimum api-version: 2015-06-15
*
*/
public Optional licenseType() {
return Optional.ofNullable(this.licenseType);
}
/**
* @return Specifies properties of the network interfaces of the virtual machines in the
* scale set.
*
*/
public Optional networkProfile() {
return Optional.ofNullable(this.networkProfile);
}
/**
* @return Specifies the operating system settings for the virtual machines in the scale
* set.
*
*/
public Optional osProfile() {
return Optional.ofNullable(this.osProfile);
}
/**
* @return Specifies Scheduled Event related configurations.
*
*/
public Optional scheduledEventsProfile() {
return Optional.ofNullable(this.scheduledEventsProfile);
}
/**
* @return Specifies the security posture to be used for all virtual machines in the scale
* set. Minimum api-version: 2023-03-01
*
*/
public Optional securityPostureReference() {
return Optional.ofNullable(this.securityPostureReference);
}
/**
* @return Specifies the Security related profile settings for the virtual machines in the
* scale set.
*
*/
public Optional securityProfile() {
return Optional.ofNullable(this.securityProfile);
}
/**
* @return Specifies the service artifact reference id used to set same image version for
* all virtual machines in the scale set when using 'latest' image version.
* Minimum api-version: 2022-11-01
*
*/
public Optional serviceArtifactReference() {
return Optional.ofNullable(this.serviceArtifactReference);
}
/**
* @return Specifies the storage settings for the virtual machine disks.
*
*/
public Optional storageProfile() {
return Optional.ofNullable(this.storageProfile);
}
/**
* @return Specifies the time in which this VM profile for the Virtual Machine Scale Set
* was created. Minimum API version for this property is 2023-09-01. This value
* will be added to VMSS Flex VM tags when creating/updating the VMSS VM Profile
* with minimum api-version 2023-09-01. Examples: "2024-07-01T00:00:01.1234567+00:00"
*
*/
public String timeCreated() {
return this.timeCreated;
}
/**
* @return UserData for the virtual machines in the scale set, which must be base-64
* encoded. Customer should not pass any secrets in here. Minimum api-version:
* 2021-03-01.
*
*/
public Optional userData() {
return Optional.ofNullable(this.userData);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BaseVirtualMachineProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable ApplicationProfileResponse applicationProfile;
private @Nullable CapacityReservationProfileResponse capacityReservation;
private @Nullable DiagnosticsProfileResponse diagnosticsProfile;
private @Nullable VirtualMachineScaleSetExtensionProfileResponse extensionProfile;
private @Nullable VirtualMachineScaleSetHardwareProfileResponse hardwareProfile;
private @Nullable String licenseType;
private @Nullable VirtualMachineScaleSetNetworkProfileResponse networkProfile;
private @Nullable VirtualMachineScaleSetOSProfileResponse osProfile;
private @Nullable ScheduledEventsProfileResponse scheduledEventsProfile;
private @Nullable SecurityPostureReferenceResponse securityPostureReference;
private @Nullable SecurityProfileResponse securityProfile;
private @Nullable ServiceArtifactReferenceResponse serviceArtifactReference;
private @Nullable VirtualMachineScaleSetStorageProfileResponse storageProfile;
private String timeCreated;
private @Nullable String userData;
public Builder() {}
public Builder(BaseVirtualMachineProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.applicationProfile = defaults.applicationProfile;
this.capacityReservation = defaults.capacityReservation;
this.diagnosticsProfile = defaults.diagnosticsProfile;
this.extensionProfile = defaults.extensionProfile;
this.hardwareProfile = defaults.hardwareProfile;
this.licenseType = defaults.licenseType;
this.networkProfile = defaults.networkProfile;
this.osProfile = defaults.osProfile;
this.scheduledEventsProfile = defaults.scheduledEventsProfile;
this.securityPostureReference = defaults.securityPostureReference;
this.securityProfile = defaults.securityProfile;
this.serviceArtifactReference = defaults.serviceArtifactReference;
this.storageProfile = defaults.storageProfile;
this.timeCreated = defaults.timeCreated;
this.userData = defaults.userData;
}
@CustomType.Setter
public Builder applicationProfile(@Nullable ApplicationProfileResponse applicationProfile) {
this.applicationProfile = applicationProfile;
return this;
}
@CustomType.Setter
public Builder capacityReservation(@Nullable CapacityReservationProfileResponse capacityReservation) {
this.capacityReservation = capacityReservation;
return this;
}
@CustomType.Setter
public Builder diagnosticsProfile(@Nullable DiagnosticsProfileResponse diagnosticsProfile) {
this.diagnosticsProfile = diagnosticsProfile;
return this;
}
@CustomType.Setter
public Builder extensionProfile(@Nullable VirtualMachineScaleSetExtensionProfileResponse extensionProfile) {
this.extensionProfile = extensionProfile;
return this;
}
@CustomType.Setter
public Builder hardwareProfile(@Nullable VirtualMachineScaleSetHardwareProfileResponse hardwareProfile) {
this.hardwareProfile = hardwareProfile;
return this;
}
@CustomType.Setter
public Builder licenseType(@Nullable String licenseType) {
this.licenseType = licenseType;
return this;
}
@CustomType.Setter
public Builder networkProfile(@Nullable VirtualMachineScaleSetNetworkProfileResponse networkProfile) {
this.networkProfile = networkProfile;
return this;
}
@CustomType.Setter
public Builder osProfile(@Nullable VirtualMachineScaleSetOSProfileResponse osProfile) {
this.osProfile = osProfile;
return this;
}
@CustomType.Setter
public Builder scheduledEventsProfile(@Nullable ScheduledEventsProfileResponse scheduledEventsProfile) {
this.scheduledEventsProfile = scheduledEventsProfile;
return this;
}
@CustomType.Setter
public Builder securityPostureReference(@Nullable SecurityPostureReferenceResponse securityPostureReference) {
this.securityPostureReference = securityPostureReference;
return this;
}
@CustomType.Setter
public Builder securityProfile(@Nullable SecurityProfileResponse securityProfile) {
this.securityProfile = securityProfile;
return this;
}
@CustomType.Setter
public Builder serviceArtifactReference(@Nullable ServiceArtifactReferenceResponse serviceArtifactReference) {
this.serviceArtifactReference = serviceArtifactReference;
return this;
}
@CustomType.Setter
public Builder storageProfile(@Nullable VirtualMachineScaleSetStorageProfileResponse storageProfile) {
this.storageProfile = storageProfile;
return this;
}
@CustomType.Setter
public Builder timeCreated(String timeCreated) {
if (timeCreated == null) {
throw new MissingRequiredPropertyException("BaseVirtualMachineProfileResponse", "timeCreated");
}
this.timeCreated = timeCreated;
return this;
}
@CustomType.Setter
public Builder userData(@Nullable String userData) {
this.userData = userData;
return this;
}
public BaseVirtualMachineProfileResponse build() {
final var _resultValue = new BaseVirtualMachineProfileResponse();
_resultValue.applicationProfile = applicationProfile;
_resultValue.capacityReservation = capacityReservation;
_resultValue.diagnosticsProfile = diagnosticsProfile;
_resultValue.extensionProfile = extensionProfile;
_resultValue.hardwareProfile = hardwareProfile;
_resultValue.licenseType = licenseType;
_resultValue.networkProfile = networkProfile;
_resultValue.osProfile = osProfile;
_resultValue.scheduledEventsProfile = scheduledEventsProfile;
_resultValue.securityPostureReference = securityPostureReference;
_resultValue.securityProfile = securityProfile;
_resultValue.serviceArtifactReference = serviceArtifactReference;
_resultValue.storageProfile = storageProfile;
_resultValue.timeCreated = timeCreated;
_resultValue.userData = userData;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy