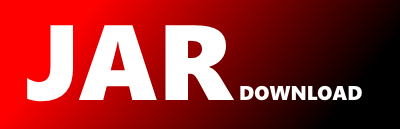
com.pulumi.azurenative.azuresphere.outputs.DeviceInsightResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azuresphere.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class DeviceInsightResponse {
/**
* @return Event description
*
*/
private String description;
/**
* @return Device ID
*
*/
private String deviceId;
/**
* @return Event end timestamp
*
*/
private String endTimestampUtc;
/**
* @return Event category
*
*/
private String eventCategory;
/**
* @return Event class
*
*/
private String eventClass;
/**
* @return Event count
*
*/
private Integer eventCount;
/**
* @return Event type
*
*/
private String eventType;
/**
* @return Event start timestamp
*
*/
private String startTimestampUtc;
private DeviceInsightResponse() {}
/**
* @return Event description
*
*/
public String description() {
return this.description;
}
/**
* @return Device ID
*
*/
public String deviceId() {
return this.deviceId;
}
/**
* @return Event end timestamp
*
*/
public String endTimestampUtc() {
return this.endTimestampUtc;
}
/**
* @return Event category
*
*/
public String eventCategory() {
return this.eventCategory;
}
/**
* @return Event class
*
*/
public String eventClass() {
return this.eventClass;
}
/**
* @return Event count
*
*/
public Integer eventCount() {
return this.eventCount;
}
/**
* @return Event type
*
*/
public String eventType() {
return this.eventType;
}
/**
* @return Event start timestamp
*
*/
public String startTimestampUtc() {
return this.startTimestampUtc;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DeviceInsightResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String description;
private String deviceId;
private String endTimestampUtc;
private String eventCategory;
private String eventClass;
private Integer eventCount;
private String eventType;
private String startTimestampUtc;
public Builder() {}
public Builder(DeviceInsightResponse defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.deviceId = defaults.deviceId;
this.endTimestampUtc = defaults.endTimestampUtc;
this.eventCategory = defaults.eventCategory;
this.eventClass = defaults.eventClass;
this.eventCount = defaults.eventCount;
this.eventType = defaults.eventType;
this.startTimestampUtc = defaults.startTimestampUtc;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder deviceId(String deviceId) {
if (deviceId == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "deviceId");
}
this.deviceId = deviceId;
return this;
}
@CustomType.Setter
public Builder endTimestampUtc(String endTimestampUtc) {
if (endTimestampUtc == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "endTimestampUtc");
}
this.endTimestampUtc = endTimestampUtc;
return this;
}
@CustomType.Setter
public Builder eventCategory(String eventCategory) {
if (eventCategory == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "eventCategory");
}
this.eventCategory = eventCategory;
return this;
}
@CustomType.Setter
public Builder eventClass(String eventClass) {
if (eventClass == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "eventClass");
}
this.eventClass = eventClass;
return this;
}
@CustomType.Setter
public Builder eventCount(Integer eventCount) {
if (eventCount == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "eventCount");
}
this.eventCount = eventCount;
return this;
}
@CustomType.Setter
public Builder eventType(String eventType) {
if (eventType == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "eventType");
}
this.eventType = eventType;
return this;
}
@CustomType.Setter
public Builder startTimestampUtc(String startTimestampUtc) {
if (startTimestampUtc == null) {
throw new MissingRequiredPropertyException("DeviceInsightResponse", "startTimestampUtc");
}
this.startTimestampUtc = startTimestampUtc;
return this;
}
public DeviceInsightResponse build() {
final var _resultValue = new DeviceInsightResponse();
_resultValue.description = description;
_resultValue.deviceId = deviceId;
_resultValue.endTimestampUtc = endTimestampUtc;
_resultValue.eventCategory = eventCategory;
_resultValue.eventClass = eventClass;
_resultValue.eventCount = eventCount;
_resultValue.eventType = eventType;
_resultValue.startTimestampUtc = startTimestampUtc;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy