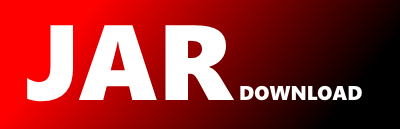
com.pulumi.azurenative.azurestackhci.Update Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.azurestackhci.UpdateArgs;
import com.pulumi.azurenative.azurestackhci.outputs.SystemDataResponse;
import com.pulumi.azurenative.azurestackhci.outputs.UpdatePrerequisiteResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Update details
* Azure REST API version: 2023-03-01.
*
* Other available API versions: 2022-12-15-preview, 2023-06-01, 2023-08-01, 2023-08-01-preview, 2023-11-01-preview, 2024-01-01, 2024-02-15-preview, 2024-04-01.
*
* ## Example Usage
* ### Put a specific update
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.Update;
* import com.pulumi.azurenative.azurestackhci.UpdateArgs;
* import com.pulumi.azurenative.azurestackhci.inputs.UpdatePrerequisiteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var update = new Update("update", UpdateArgs.builder()
* .additionalProperties("additional properties")
* .availabilityType("Local")
* .clusterName("testcluster")
* .description("AzS Update 4.2203.2.32")
* .displayName("AzS Update - 4.2203.2.32")
* .installedDate("2022-04-06T14:08:18.254Z")
* .notifyMessage("Brief message with instructions for updates of AvailabilityType Notify")
* .packagePath("\\\\SU1FileServer\\SU1_Infrastructure_2\\Updates\\Packages\\Microsoft4.2203.2.32")
* .packageSizeInMb(18858)
* .packageType("Infrastructure")
* .prerequisites(UpdatePrerequisiteArgs.builder()
* .packageName("update package name")
* .updateType("update type")
* .version("prerequisite version")
* .build())
* .progressPercentage(0)
* .publisher("Microsoft")
* .releaseLink("https://docs.microsoft.com/azure-stack/operator/release-notes?view=azs-2203")
* .resourceGroupName("testrg")
* .state("Installed")
* .updateName("Microsoft4.2203.2.32")
* .version("4.2203.2.32")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:azurestackhci:Update Microsoft4.2203.2.32 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AzureStackHCI/clusters/{clusterName}/updates/{updateName}
* ```
*
*/
@ResourceType(type="azure-native:azurestackhci:Update")
public class Update extends com.pulumi.resources.CustomResource {
/**
* Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
*
*/
@Export(name="additionalProperties", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> additionalProperties;
/**
* @return Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
*
*/
public Output> additionalProperties() {
return Codegen.optional(this.additionalProperties);
}
/**
* Indicates the way the update content can be downloaded.
*
*/
@Export(name="availabilityType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> availabilityType;
/**
* @return Indicates the way the update content can be downloaded.
*
*/
public Output> availabilityType() {
return Codegen.optional(this.availabilityType);
}
/**
* Description of the update.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of the update.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Display name of the Update
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return Display name of the Update
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Last time the package-specific checks were run.
*
*/
@Export(name="healthCheckDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> healthCheckDate;
/**
* @return Last time the package-specific checks were run.
*
*/
public Output> healthCheckDate() {
return Codegen.optional(this.healthCheckDate);
}
/**
* Date that the update was installed.
*
*/
@Export(name="installedDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> installedDate;
/**
* @return Date that the update was installed.
*
*/
public Output> installedDate() {
return Codegen.optional(this.installedDate);
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> location;
/**
* @return The geo-location where the resource lives
*
*/
public Output> location() {
return Codegen.optional(this.location);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Brief message with instructions for updates of AvailabilityType Notify.
*
*/
@Export(name="notifyMessage", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> notifyMessage;
/**
* @return Brief message with instructions for updates of AvailabilityType Notify.
*
*/
public Output> notifyMessage() {
return Codegen.optional(this.notifyMessage);
}
/**
* Path where the update package is available.
*
*/
@Export(name="packagePath", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> packagePath;
/**
* @return Path where the update package is available.
*
*/
public Output> packagePath() {
return Codegen.optional(this.packagePath);
}
/**
* Size of the package. This value is a combination of the size from update metadata and size of the payload that results from the live scan operation for OS update content.
*
*/
@Export(name="packageSizeInMb", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> packageSizeInMb;
/**
* @return Size of the package. This value is a combination of the size from update metadata and size of the payload that results from the live scan operation for OS update content.
*
*/
public Output> packageSizeInMb() {
return Codegen.optional(this.packageSizeInMb);
}
/**
* Customer-visible type of the update.
*
*/
@Export(name="packageType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> packageType;
/**
* @return Customer-visible type of the update.
*
*/
public Output> packageType() {
return Codegen.optional(this.packageType);
}
/**
* If update State is HasPrerequisite, this property contains an array of objects describing prerequisite updates before installing this update. Otherwise, it is empty.
*
*/
@Export(name="prerequisites", refs={List.class,UpdatePrerequisiteResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> prerequisites;
/**
* @return If update State is HasPrerequisite, this property contains an array of objects describing prerequisite updates before installing this update. Otherwise, it is empty.
*
*/
public Output>> prerequisites() {
return Codegen.optional(this.prerequisites);
}
/**
* Progress percentage of ongoing operation. Currently this property is only valid when the update is in the Downloading state, where it maps to how much of the update content has been downloaded.
*
*/
@Export(name="progressPercentage", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> progressPercentage;
/**
* @return Progress percentage of ongoing operation. Currently this property is only valid when the update is in the Downloading state, where it maps to how much of the update content has been downloaded.
*
*/
public Output> progressPercentage() {
return Codegen.optional(this.progressPercentage);
}
/**
* Provisioning state of the Updates proxy resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the Updates proxy resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Publisher of the update package.
*
*/
@Export(name="publisher", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publisher;
/**
* @return Publisher of the update package.
*
*/
public Output> publisher() {
return Codegen.optional(this.publisher);
}
/**
* Link to release notes for the update.
*
*/
@Export(name="releaseLink", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> releaseLink;
/**
* @return Link to release notes for the update.
*
*/
public Output> releaseLink() {
return Codegen.optional(this.releaseLink);
}
/**
* State of the update as it relates to this stamp.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> state;
/**
* @return State of the update as it relates to this stamp.
*
*/
public Output> state() {
return Codegen.optional(this.state);
}
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* Version of the update.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> version;
/**
* @return Version of the update.
*
*/
public Output> version() {
return Codegen.optional(this.version);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Update(java.lang.String name) {
this(name, UpdateArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Update(java.lang.String name, UpdateArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Update(java.lang.String name, UpdateArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:Update", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Update(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:azurestackhci:Update", name, null, makeResourceOptions(options, id), false);
}
private static UpdateArgs makeArgs(UpdateArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? UpdateArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:azurestackhci/v20221201:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20221215preview:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230201:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230301:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230601:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230801:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20230801preview:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20231101preview:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240101:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240215preview:Update").build()),
Output.of(Alias.builder().type("azure-native:azurestackhci/v20240401:Update").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Update get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Update(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy