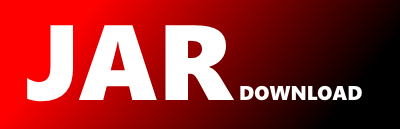
com.pulumi.azurenative.azurestackhci.outputs.ClusterReportedPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci.outputs;
import com.pulumi.azurenative.azurestackhci.outputs.ClusterNodeResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterReportedPropertiesResponse {
/**
* @return Unique id generated by the on-prem cluster.
*
*/
private String clusterId;
/**
* @return Name of the on-prem cluster connected to this resource.
*
*/
private String clusterName;
/**
* @return The node type of all the nodes of the cluster.
*
*/
private String clusterType;
/**
* @return Version of the cluster software.
*
*/
private String clusterVersion;
/**
* @return Level of diagnostic data emitted by the cluster.
*
*/
private @Nullable String diagnosticLevel;
/**
* @return IMDS attestation status of the cluster.
*
*/
private String imdsAttestation;
/**
* @return Last time the cluster reported the data.
*
*/
private String lastUpdated;
/**
* @return The manufacturer of all the nodes of the cluster.
*
*/
private String manufacturer;
/**
* @return List of nodes reported by the cluster.
*
*/
private List nodes;
/**
* @return Capabilities supported by the cluster.
*
*/
private List supportedCapabilities;
private ClusterReportedPropertiesResponse() {}
/**
* @return Unique id generated by the on-prem cluster.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return Name of the on-prem cluster connected to this resource.
*
*/
public String clusterName() {
return this.clusterName;
}
/**
* @return The node type of all the nodes of the cluster.
*
*/
public String clusterType() {
return this.clusterType;
}
/**
* @return Version of the cluster software.
*
*/
public String clusterVersion() {
return this.clusterVersion;
}
/**
* @return Level of diagnostic data emitted by the cluster.
*
*/
public Optional diagnosticLevel() {
return Optional.ofNullable(this.diagnosticLevel);
}
/**
* @return IMDS attestation status of the cluster.
*
*/
public String imdsAttestation() {
return this.imdsAttestation;
}
/**
* @return Last time the cluster reported the data.
*
*/
public String lastUpdated() {
return this.lastUpdated;
}
/**
* @return The manufacturer of all the nodes of the cluster.
*
*/
public String manufacturer() {
return this.manufacturer;
}
/**
* @return List of nodes reported by the cluster.
*
*/
public List nodes() {
return this.nodes;
}
/**
* @return Capabilities supported by the cluster.
*
*/
public List supportedCapabilities() {
return this.supportedCapabilities;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterReportedPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String clusterId;
private String clusterName;
private String clusterType;
private String clusterVersion;
private @Nullable String diagnosticLevel;
private String imdsAttestation;
private String lastUpdated;
private String manufacturer;
private List nodes;
private List supportedCapabilities;
public Builder() {}
public Builder(ClusterReportedPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.clusterId = defaults.clusterId;
this.clusterName = defaults.clusterName;
this.clusterType = defaults.clusterType;
this.clusterVersion = defaults.clusterVersion;
this.diagnosticLevel = defaults.diagnosticLevel;
this.imdsAttestation = defaults.imdsAttestation;
this.lastUpdated = defaults.lastUpdated;
this.manufacturer = defaults.manufacturer;
this.nodes = defaults.nodes;
this.supportedCapabilities = defaults.supportedCapabilities;
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder clusterName(String clusterName) {
if (clusterName == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "clusterName");
}
this.clusterName = clusterName;
return this;
}
@CustomType.Setter
public Builder clusterType(String clusterType) {
if (clusterType == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "clusterType");
}
this.clusterType = clusterType;
return this;
}
@CustomType.Setter
public Builder clusterVersion(String clusterVersion) {
if (clusterVersion == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "clusterVersion");
}
this.clusterVersion = clusterVersion;
return this;
}
@CustomType.Setter
public Builder diagnosticLevel(@Nullable String diagnosticLevel) {
this.diagnosticLevel = diagnosticLevel;
return this;
}
@CustomType.Setter
public Builder imdsAttestation(String imdsAttestation) {
if (imdsAttestation == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "imdsAttestation");
}
this.imdsAttestation = imdsAttestation;
return this;
}
@CustomType.Setter
public Builder lastUpdated(String lastUpdated) {
if (lastUpdated == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "lastUpdated");
}
this.lastUpdated = lastUpdated;
return this;
}
@CustomType.Setter
public Builder manufacturer(String manufacturer) {
if (manufacturer == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "manufacturer");
}
this.manufacturer = manufacturer;
return this;
}
@CustomType.Setter
public Builder nodes(List nodes) {
if (nodes == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "nodes");
}
this.nodes = nodes;
return this;
}
public Builder nodes(ClusterNodeResponse... nodes) {
return nodes(List.of(nodes));
}
@CustomType.Setter
public Builder supportedCapabilities(List supportedCapabilities) {
if (supportedCapabilities == null) {
throw new MissingRequiredPropertyException("ClusterReportedPropertiesResponse", "supportedCapabilities");
}
this.supportedCapabilities = supportedCapabilities;
return this;
}
public Builder supportedCapabilities(String... supportedCapabilities) {
return supportedCapabilities(List.of(supportedCapabilities));
}
public ClusterReportedPropertiesResponse build() {
final var _resultValue = new ClusterReportedPropertiesResponse();
_resultValue.clusterId = clusterId;
_resultValue.clusterName = clusterName;
_resultValue.clusterType = clusterType;
_resultValue.clusterVersion = clusterVersion;
_resultValue.diagnosticLevel = diagnosticLevel;
_resultValue.imdsAttestation = imdsAttestation;
_resultValue.lastUpdated = lastUpdated;
_resultValue.manufacturer = manufacturer;
_resultValue.nodes = nodes;
_resultValue.supportedCapabilities = supportedCapabilities;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy