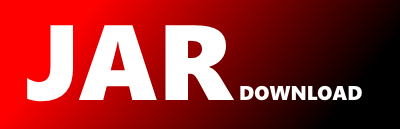
com.pulumi.azurenative.batch.BatchAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.batch.BatchAccountArgs;
import com.pulumi.azurenative.batch.outputs.AutoStoragePropertiesResponse;
import com.pulumi.azurenative.batch.outputs.BatchAccountIdentityResponse;
import com.pulumi.azurenative.batch.outputs.EncryptionPropertiesResponse;
import com.pulumi.azurenative.batch.outputs.KeyVaultReferenceResponse;
import com.pulumi.azurenative.batch.outputs.NetworkProfileResponse;
import com.pulumi.azurenative.batch.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.batch.outputs.VirtualMachineFamilyCoreQuotaResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Contains information about an Azure Batch account.
* Azure REST API version: 2023-05-01. Prior API version in Azure Native 1.x: 2021-01-01.
*
* Other available API versions: 2017-01-01, 2022-01-01, 2023-11-01, 2024-02-01, 2024-07-01.
*
* ## Example Usage
* ### BatchAccountCreate_BYOS
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.BatchAccount;
* import com.pulumi.azurenative.batch.BatchAccountArgs;
* import com.pulumi.azurenative.batch.inputs.AutoStorageBasePropertiesArgs;
* import com.pulumi.azurenative.batch.inputs.KeyVaultReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var batchAccount = new BatchAccount("batchAccount", BatchAccountArgs.builder()
* .accountName("sampleacct")
* .autoStorage(AutoStorageBasePropertiesArgs.builder()
* .storageAccountId("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Storage/storageAccounts/samplestorage")
* .build())
* .keyVaultReference(KeyVaultReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.KeyVault/vaults/sample")
* .url("http://sample.vault.azure.net/")
* .build())
* .location("japaneast")
* .poolAllocationMode("UserSubscription")
* .resourceGroupName("default-azurebatch-japaneast")
* .build());
*
* }
* }
*
* }
*
* ### BatchAccountCreate_Default
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.BatchAccount;
* import com.pulumi.azurenative.batch.BatchAccountArgs;
* import com.pulumi.azurenative.batch.inputs.AutoStorageBasePropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var batchAccount = new BatchAccount("batchAccount", BatchAccountArgs.builder()
* .accountName("sampleacct")
* .autoStorage(AutoStorageBasePropertiesArgs.builder()
* .storageAccountId("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Storage/storageAccounts/samplestorage")
* .build())
* .location("japaneast")
* .resourceGroupName("default-azurebatch-japaneast")
* .build());
*
* }
* }
*
* }
*
* ### BatchAccountCreate_SystemAssignedIdentity
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.BatchAccount;
* import com.pulumi.azurenative.batch.BatchAccountArgs;
* import com.pulumi.azurenative.batch.inputs.AutoStorageBasePropertiesArgs;
* import com.pulumi.azurenative.batch.inputs.BatchAccountIdentityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var batchAccount = new BatchAccount("batchAccount", BatchAccountArgs.builder()
* .accountName("sampleacct")
* .autoStorage(AutoStorageBasePropertiesArgs.builder()
* .storageAccountId("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Storage/storageAccounts/samplestorage")
* .build())
* .identity(BatchAccountIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .location("japaneast")
* .resourceGroupName("default-azurebatch-japaneast")
* .build());
*
* }
* }
*
* }
*
* ### PrivateBatchAccountCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.BatchAccount;
* import com.pulumi.azurenative.batch.BatchAccountArgs;
* import com.pulumi.azurenative.batch.inputs.AutoStorageBasePropertiesArgs;
* import com.pulumi.azurenative.batch.inputs.KeyVaultReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var batchAccount = new BatchAccount("batchAccount", BatchAccountArgs.builder()
* .accountName("sampleacct")
* .autoStorage(AutoStorageBasePropertiesArgs.builder()
* .storageAccountId("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Storage/storageAccounts/samplestorage")
* .build())
* .keyVaultReference(KeyVaultReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.KeyVault/vaults/sample")
* .url("http://sample.vault.azure.net/")
* .build())
* .location("japaneast")
* .publicNetworkAccess("Disabled")
* .resourceGroupName("default-azurebatch-japaneast")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:batch:BatchAccount sampleacct /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}
* ```
*
*/
@ResourceType(type="azure-native:batch:BatchAccount")
public class BatchAccount extends com.pulumi.resources.CustomResource {
/**
* The account endpoint used to interact with the Batch service.
*
*/
@Export(name="accountEndpoint", refs={String.class}, tree="[0]")
private Output accountEndpoint;
/**
* @return The account endpoint used to interact with the Batch service.
*
*/
public Output accountEndpoint() {
return this.accountEndpoint;
}
@Export(name="activeJobAndJobScheduleQuota", refs={Integer.class}, tree="[0]")
private Output activeJobAndJobScheduleQuota;
public Output activeJobAndJobScheduleQuota() {
return this.activeJobAndJobScheduleQuota;
}
/**
* List of allowed authentication modes for the Batch account that can be used to authenticate with the data plane. This does not affect authentication with the control plane.
*
*/
@Export(name="allowedAuthenticationModes", refs={List.class,String.class}, tree="[0,1]")
private Output> allowedAuthenticationModes;
/**
* @return List of allowed authentication modes for the Batch account that can be used to authenticate with the data plane. This does not affect authentication with the control plane.
*
*/
public Output> allowedAuthenticationModes() {
return this.allowedAuthenticationModes;
}
/**
* Contains information about the auto-storage account associated with a Batch account.
*
*/
@Export(name="autoStorage", refs={AutoStoragePropertiesResponse.class}, tree="[0]")
private Output autoStorage;
/**
* @return Contains information about the auto-storage account associated with a Batch account.
*
*/
public Output autoStorage() {
return this.autoStorage;
}
/**
* For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
@Export(name="dedicatedCoreQuota", refs={Integer.class}, tree="[0]")
private Output dedicatedCoreQuota;
/**
* @return For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
public Output dedicatedCoreQuota() {
return this.dedicatedCoreQuota;
}
/**
* A list of the dedicated core quota per Virtual Machine family for the Batch account. For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
@Export(name="dedicatedCoreQuotaPerVMFamily", refs={List.class,VirtualMachineFamilyCoreQuotaResponse.class}, tree="[0,1]")
private Output> dedicatedCoreQuotaPerVMFamily;
/**
* @return A list of the dedicated core quota per Virtual Machine family for the Batch account. For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
public Output> dedicatedCoreQuotaPerVMFamily() {
return this.dedicatedCoreQuotaPerVMFamily;
}
/**
* If this flag is true, dedicated core quota is enforced via both the dedicatedCoreQuotaPerVMFamily and dedicatedCoreQuota properties on the account. If this flag is false, dedicated core quota is enforced only via the dedicatedCoreQuota property on the account and does not consider Virtual Machine family.
*
*/
@Export(name="dedicatedCoreQuotaPerVMFamilyEnforced", refs={Boolean.class}, tree="[0]")
private Output dedicatedCoreQuotaPerVMFamilyEnforced;
/**
* @return If this flag is true, dedicated core quota is enforced via both the dedicatedCoreQuotaPerVMFamily and dedicatedCoreQuota properties on the account. If this flag is false, dedicated core quota is enforced only via the dedicatedCoreQuota property on the account and does not consider Virtual Machine family.
*
*/
public Output dedicatedCoreQuotaPerVMFamilyEnforced() {
return this.dedicatedCoreQuotaPerVMFamilyEnforced;
}
/**
* Configures how customer data is encrypted inside the Batch account. By default, accounts are encrypted using a Microsoft managed key. For additional control, a customer-managed key can be used instead.
*
*/
@Export(name="encryption", refs={EncryptionPropertiesResponse.class}, tree="[0]")
private Output encryption;
/**
* @return Configures how customer data is encrypted inside the Batch account. By default, accounts are encrypted using a Microsoft managed key. For additional control, a customer-managed key can be used instead.
*
*/
public Output encryption() {
return this.encryption;
}
/**
* The identity of the Batch account.
*
*/
@Export(name="identity", refs={BatchAccountIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ BatchAccountIdentityResponse> identity;
/**
* @return The identity of the Batch account.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* Identifies the Azure key vault associated with a Batch account.
*
*/
@Export(name="keyVaultReference", refs={KeyVaultReferenceResponse.class}, tree="[0]")
private Output keyVaultReference;
/**
* @return Identifies the Azure key vault associated with a Batch account.
*
*/
public Output keyVaultReference() {
return this.keyVaultReference;
}
/**
* The location of the resource.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The location of the resource.
*
*/
public Output location() {
return this.location;
}
/**
* For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
@Export(name="lowPriorityCoreQuota", refs={Integer.class}, tree="[0]")
private Output lowPriorityCoreQuota;
/**
* @return For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
*
*/
public Output lowPriorityCoreQuota() {
return this.lowPriorityCoreQuota;
}
/**
* The name of the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource.
*
*/
public Output name() {
return this.name;
}
/**
* The network profile only takes effect when publicNetworkAccess is enabled.
*
*/
@Export(name="networkProfile", refs={NetworkProfileResponse.class}, tree="[0]")
private Output* @Nullable */ NetworkProfileResponse> networkProfile;
/**
* @return The network profile only takes effect when publicNetworkAccess is enabled.
*
*/
public Output> networkProfile() {
return Codegen.optional(this.networkProfile);
}
/**
* The endpoint used by compute node to connect to the Batch node management service.
*
*/
@Export(name="nodeManagementEndpoint", refs={String.class}, tree="[0]")
private Output nodeManagementEndpoint;
/**
* @return The endpoint used by compute node to connect to the Batch node management service.
*
*/
public Output nodeManagementEndpoint() {
return this.nodeManagementEndpoint;
}
/**
* The allocation mode for creating pools in the Batch account.
*
*/
@Export(name="poolAllocationMode", refs={String.class}, tree="[0]")
private Output poolAllocationMode;
/**
* @return The allocation mode for creating pools in the Batch account.
*
*/
public Output poolAllocationMode() {
return this.poolAllocationMode;
}
@Export(name="poolQuota", refs={Integer.class}, tree="[0]")
private Output poolQuota;
public Output poolQuota() {
return this.poolQuota;
}
/**
* List of private endpoint connections associated with the Batch account
*
*/
@Export(name="privateEndpointConnections", refs={List.class,PrivateEndpointConnectionResponse.class}, tree="[0,1]")
private Output> privateEndpointConnections;
/**
* @return List of private endpoint connections associated with the Batch account
*
*/
public Output> privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* The provisioned state of the resource
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioned state of the resource
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* If not specified, the default value is 'enabled'.
*
*/
@Export(name="publicNetworkAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicNetworkAccess;
/**
* @return If not specified, the default value is 'enabled'.
*
*/
public Output> publicNetworkAccess() {
return Codegen.optional(this.publicNetworkAccess);
}
/**
* The tags of the resource.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy