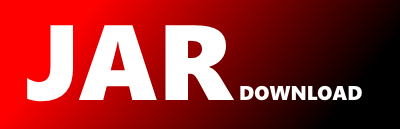
com.pulumi.azurenative.batch.BatchAccountArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch;
import com.pulumi.azurenative.batch.enums.AuthenticationMode;
import com.pulumi.azurenative.batch.enums.PoolAllocationMode;
import com.pulumi.azurenative.batch.enums.PublicNetworkAccessType;
import com.pulumi.azurenative.batch.inputs.AutoStorageBasePropertiesArgs;
import com.pulumi.azurenative.batch.inputs.BatchAccountIdentityArgs;
import com.pulumi.azurenative.batch.inputs.EncryptionPropertiesArgs;
import com.pulumi.azurenative.batch.inputs.KeyVaultReferenceArgs;
import com.pulumi.azurenative.batch.inputs.NetworkProfileArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BatchAccountArgs extends com.pulumi.resources.ResourceArgs {
public static final BatchAccountArgs Empty = new BatchAccountArgs();
/**
* A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
*
*/
@Import(name="accountName")
private @Nullable Output accountName;
/**
* @return A name for the Batch account which must be unique within the region. Batch account names must be between 3 and 24 characters in length and must use only numbers and lowercase letters. This name is used as part of the DNS name that is used to access the Batch service in the region in which the account is created. For example: http://accountname.region.batch.azure.com/.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy