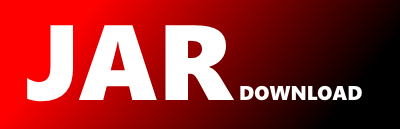
com.pulumi.azurenative.batch.inputs.DataDiskArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch.inputs;
import com.pulumi.azurenative.batch.enums.CachingType;
import com.pulumi.azurenative.batch.enums.StorageAccountType;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Settings which will be used by the data disks associated to Compute Nodes in the Pool. When using attached data disks, you need to mount and format the disks from within a VM to use them.
*
*/
public final class DataDiskArgs extends com.pulumi.resources.ResourceArgs {
public static final DataDiskArgs Empty = new DataDiskArgs();
/**
* Values are:
*
* none - The caching mode for the disk is not enabled.
* readOnly - The caching mode for the disk is read only.
* readWrite - The caching mode for the disk is read and write.
*
* The default value for caching is none. For information about the caching options see: https://blogs.msdn.microsoft.com/windowsazurestorage/2012/06/27/exploring-windows-azure-drives-disks-and-images/.
*
*/
@Import(name="caching")
private @Nullable Output caching;
/**
* @return Values are:
*
* none - The caching mode for the disk is not enabled.
* readOnly - The caching mode for the disk is read only.
* readWrite - The caching mode for the disk is read and write.
*
* The default value for caching is none. For information about the caching options see: https://blogs.msdn.microsoft.com/windowsazurestorage/2012/06/27/exploring-windows-azure-drives-disks-and-images/.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy