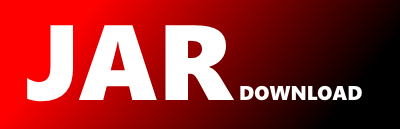
com.pulumi.azurenative.batch.outputs.AzureBlobFileSystemConfigurationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.batch.outputs;
import com.pulumi.azurenative.batch.outputs.ComputeNodeIdentityReferenceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AzureBlobFileSystemConfigurationResponse {
/**
* @return This property is mutually exclusive with both sasKey and identity; exactly one must be specified.
*
*/
private @Nullable String accountKey;
private String accountName;
/**
* @return These are 'net use' options in Windows and 'mount' options in Linux.
*
*/
private @Nullable String blobfuseOptions;
private String containerName;
/**
* @return This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
*
*/
private @Nullable ComputeNodeIdentityReferenceResponse identityReference;
/**
* @return All file systems are mounted relative to the Batch mounts directory, accessible via the AZ_BATCH_NODE_MOUNTS_DIR environment variable.
*
*/
private String relativeMountPath;
/**
* @return This property is mutually exclusive with both accountKey and identity; exactly one must be specified.
*
*/
private @Nullable String sasKey;
private AzureBlobFileSystemConfigurationResponse() {}
/**
* @return This property is mutually exclusive with both sasKey and identity; exactly one must be specified.
*
*/
public Optional accountKey() {
return Optional.ofNullable(this.accountKey);
}
public String accountName() {
return this.accountName;
}
/**
* @return These are 'net use' options in Windows and 'mount' options in Linux.
*
*/
public Optional blobfuseOptions() {
return Optional.ofNullable(this.blobfuseOptions);
}
public String containerName() {
return this.containerName;
}
/**
* @return This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
*
*/
public Optional identityReference() {
return Optional.ofNullable(this.identityReference);
}
/**
* @return All file systems are mounted relative to the Batch mounts directory, accessible via the AZ_BATCH_NODE_MOUNTS_DIR environment variable.
*
*/
public String relativeMountPath() {
return this.relativeMountPath;
}
/**
* @return This property is mutually exclusive with both accountKey and identity; exactly one must be specified.
*
*/
public Optional sasKey() {
return Optional.ofNullable(this.sasKey);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzureBlobFileSystemConfigurationResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String accountKey;
private String accountName;
private @Nullable String blobfuseOptions;
private String containerName;
private @Nullable ComputeNodeIdentityReferenceResponse identityReference;
private String relativeMountPath;
private @Nullable String sasKey;
public Builder() {}
public Builder(AzureBlobFileSystemConfigurationResponse defaults) {
Objects.requireNonNull(defaults);
this.accountKey = defaults.accountKey;
this.accountName = defaults.accountName;
this.blobfuseOptions = defaults.blobfuseOptions;
this.containerName = defaults.containerName;
this.identityReference = defaults.identityReference;
this.relativeMountPath = defaults.relativeMountPath;
this.sasKey = defaults.sasKey;
}
@CustomType.Setter
public Builder accountKey(@Nullable String accountKey) {
this.accountKey = accountKey;
return this;
}
@CustomType.Setter
public Builder accountName(String accountName) {
if (accountName == null) {
throw new MissingRequiredPropertyException("AzureBlobFileSystemConfigurationResponse", "accountName");
}
this.accountName = accountName;
return this;
}
@CustomType.Setter
public Builder blobfuseOptions(@Nullable String blobfuseOptions) {
this.blobfuseOptions = blobfuseOptions;
return this;
}
@CustomType.Setter
public Builder containerName(String containerName) {
if (containerName == null) {
throw new MissingRequiredPropertyException("AzureBlobFileSystemConfigurationResponse", "containerName");
}
this.containerName = containerName;
return this;
}
@CustomType.Setter
public Builder identityReference(@Nullable ComputeNodeIdentityReferenceResponse identityReference) {
this.identityReference = identityReference;
return this;
}
@CustomType.Setter
public Builder relativeMountPath(String relativeMountPath) {
if (relativeMountPath == null) {
throw new MissingRequiredPropertyException("AzureBlobFileSystemConfigurationResponse", "relativeMountPath");
}
this.relativeMountPath = relativeMountPath;
return this;
}
@CustomType.Setter
public Builder sasKey(@Nullable String sasKey) {
this.sasKey = sasKey;
return this;
}
public AzureBlobFileSystemConfigurationResponse build() {
final var _resultValue = new AzureBlobFileSystemConfigurationResponse();
_resultValue.accountKey = accountKey;
_resultValue.accountName = accountName;
_resultValue.blobfuseOptions = blobfuseOptions;
_resultValue.containerName = containerName;
_resultValue.identityReference = identityReference;
_resultValue.relativeMountPath = relativeMountPath;
_resultValue.sasKey = sasKey;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy